How to Convert a String to a Byte Array in C#
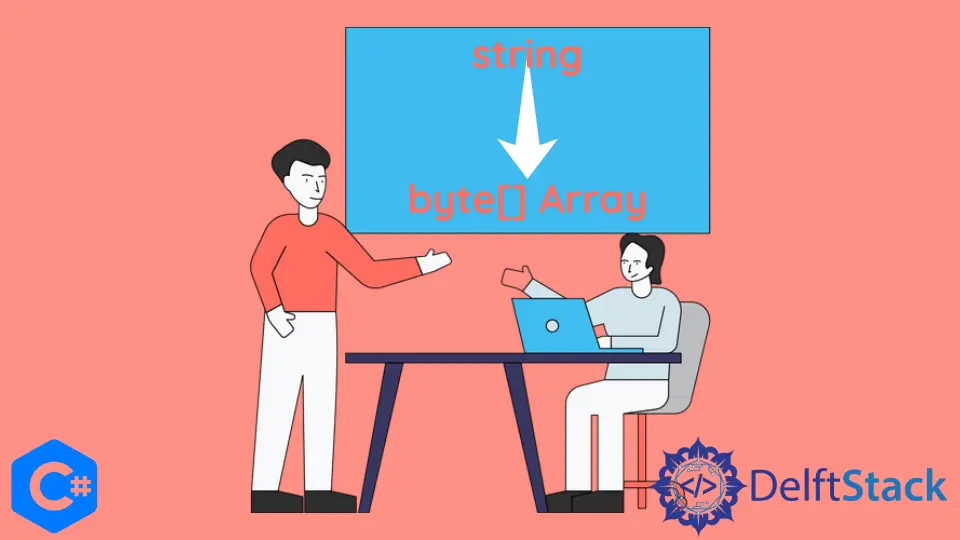
We have learned how to convert a byte array to a string in C# in the last article, and we will introduce a method to convert a string to a byte array in C#.
- Use the
GetBytes()
method
Use the GetBytes()
Method to Convert a String to a Byte Array in C#
In C#, we can use the GetBytes()
method of Encoding
class to convert a string to a byte array. There are multiple encodings that we can convert into a byte array. These encodings are ASCII
, Unicode
, UTF32
, etc. This method has multiple overloads. We will use the following overload in this case. The correct syntax to use this method is as follows.
Encoding.GetBytes(String stringName);
This overload of the method GetBytes()
has one parameter only. The detail of its parameter is as follows.
Parameters | Description | |
---|---|---|
stringName |
mandatory | This is the string that we want to convert to a byte array |
This function returns a byte array representing the given string in bytes.
The program below shows how we can use the GetBytes()
method to convert a string to a byte array.
using System;
using System.Text;
class StringToByteArray {
static void Main(string[] args) {
string myString = "This is a string.";
byte[] byteArray = Encoding.ASCII.GetBytes(myString);
Console.WriteLine("The Byte Array is:");
foreach (byte bytes in byteArray) {
Console.WriteLine(bytes);
}
}
}
Output:
The Byte Array is:
84
104
105
115
32
105
115
32
97
32
115
116
114
105
110
103
46
Related Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#