How to Get URL of Current Page in C#
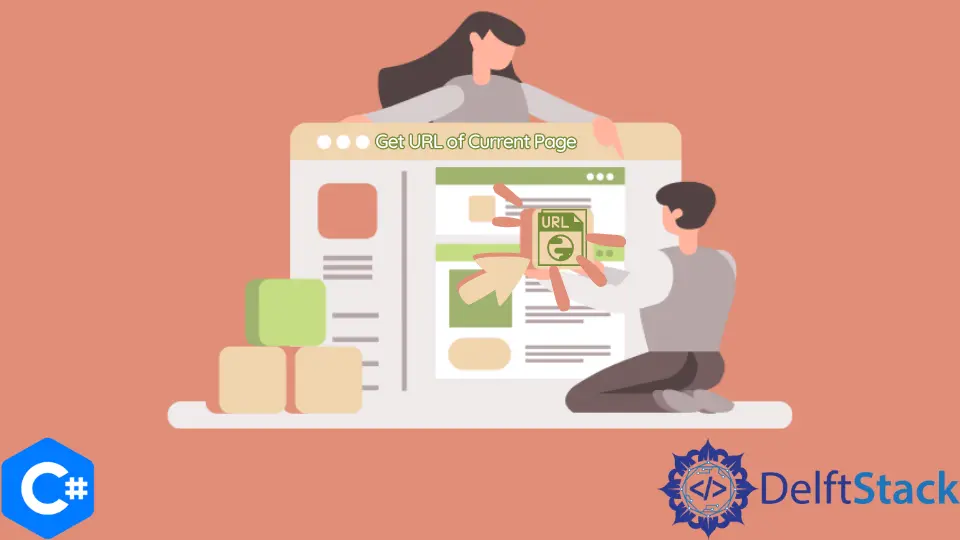
This tutorial will discuss the method for getting the URL of the current page in C#.
Get URL of the Current Page With the HttpContext
Class in C#
The HttpContext
class in C# handles all the information about a specific HTTP request. We can use the HttpContext
class to get the URL of the current web page. See the following example.
string url = HttpContext.Current.Request.Url.AbsoluteUri;
The above code will give us a URL as given below.
http: // localhost:5555/TUTORIAL/Default.aspx
We can also use the HttpContext
class to get different parts of the URL like hostname, port number, etc. The following code example shows us how we can get the hostname with the HttpContext
class.
string hostname = HttpContext.Current.Request.Url.Host;
This code will save the hostname inside the hostname
variable like.
localhost
We can also get the port number with the HttpContext
class. The following code example shows us how we can get the port number with the HttpContext
class in C#.
string portnumber = HttpContext.Current.Request.Url.Port;
The above code will save the port number inside the portnumber
variable like.
5555
We can also get the path of the current web page in C#. In the first example, the path is the part of the URL after the port number. The following code example shows us how we can get the path of the current web page in C#.
string path = HttpContext.Current.Request.Url.AbsolutePath;
The above code will save the following value inside the path
variable.
/ TUTORIAL / Default.aspx
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn