How to Get Current Time in C#
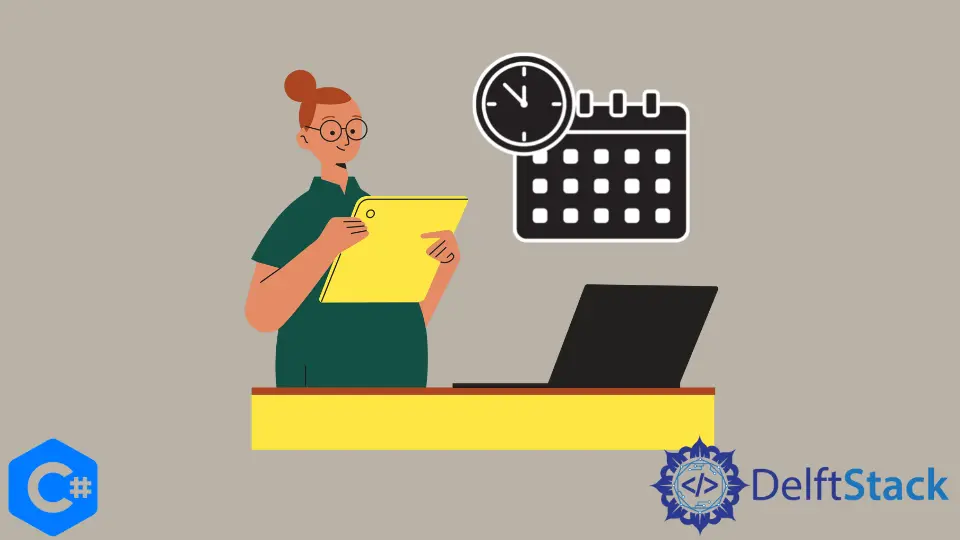
This tutorial will discuss methods to get the current time in a string variable in C#.
Get Current Time With the DateTime.Now
Property in C#
The DateTime
structure represents an instance of time in C#. The DateTime.Now
Property of the DateTime
structure gets the current date and time of our local machine expressed in our local time. We can convert the result of the DateTime.Now
property to a string variable with the DateTime.ToString()
method in C#. The following code example shows us how we can get our local machine’s current time in a string variable with the DateTime.Now
property in C#.
using System;
namespace get_current_time {
class Program {
static void Main(string[] args) {
string datetime = DateTime.Now.ToString("hh:mm:ss tt");
Console.WriteLine(datetime);
}
}
}
Output:
02:57:57 PM
We stored the current time our my local machine in the hh:mm:ss tt
format inside the datetime
string variable with the DateTime.Now
property and the DateTime.ToString()
function in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn