How to Get the Last Element of a List in C#
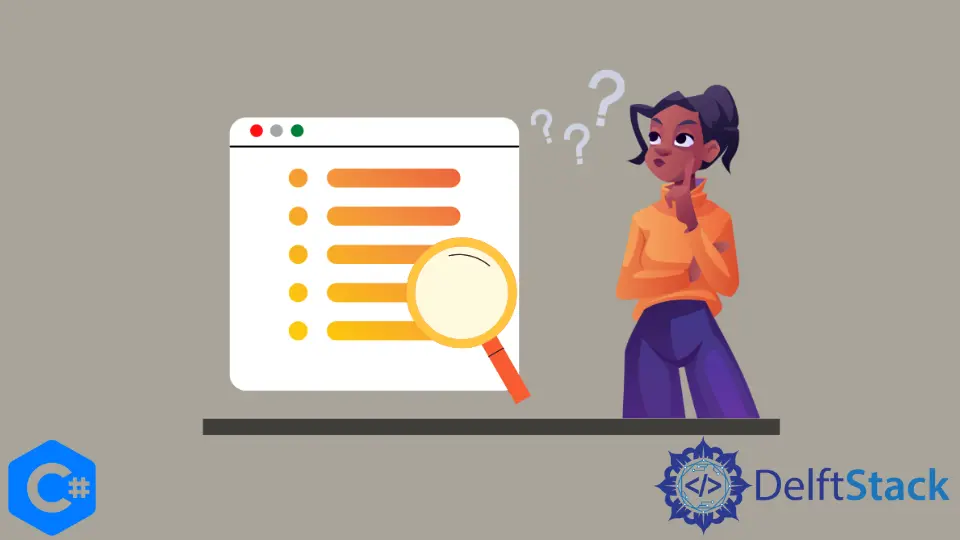
In the world of programming, manipulating lists is a common task, especially in C#. Whether you’re developing a complex application or a simple script, knowing how to access the last element of a list can save you time and effort. In C#, there are two primary methods to achieve this: using the List.Count
property and leveraging LINQ’s Last()
function. Each method has its own advantages, and understanding them will help you choose the right approach for your specific needs.
In this article, we will explore both methods in detail, providing clear examples and explanations to ensure you have a solid grasp of how to get the last element of a list in C#.
Method 1: Using List.Count Property
The first method to retrieve the last element of a list in C# is by utilizing the Count
property of the List
class. This approach is straightforward and efficient, especially when you want to access the last item directly without additional overhead.
Here’s a simple example:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<int> numbers = new List<int> { 10, 20, 30, 40, 50 };
int lastElement = numbers[numbers.Count - 1];
Console.WriteLine(lastElement);
}
}
Output:
50
In this example, we first create a list of integers called numbers
. By accessing numbers.Count
, we get the total number of elements in the list. Since lists are zero-indexed, we subtract one from the count to get the index of the last element. This method is efficient, as it directly accesses the required index without any additional processing. It works well for lists of any size, but keep in mind that if the list is empty, trying to access numbers[numbers.Count - 1]
will throw an ArgumentOutOfRangeException
. Thus, it’s a good practice to check if the list is not empty before executing this line of code.
Method 2: Using LINQ’s Last() Function
Another elegant way to retrieve the last element of a list in C# is by using LINQ’s Last()
function. This method is particularly useful for those who prefer a more functional programming style or when working with larger datasets where readability and expressiveness are important.
Here’s how you can use it:
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main()
{
List<int> numbers = new List<int> { 10, 20, 30, 40, 50 };
int lastElement = numbers.Last();
Console.WriteLine(lastElement);
}
}
Output:
50
In this code snippet, we again create a list of integers. By calling numbers.Last()
, we directly retrieve the last element of the list. This method is not only concise but also handles the empty list scenario gracefully by throwing an InvalidOperationException
if the list is empty. This can be beneficial as it clearly indicates that something went wrong, rather than silently failing. Using LINQ can enhance code readability, especially in more complex queries where you might be working with various collection manipulations.
Conclusion
Understanding how to get the last element of a list in C# is essential for efficient programming. Whether you choose to use the List.Count
property or LINQ’s Last()
function depends on your specific requirements and coding style. Both methods are effective and have their own advantages, allowing you to access the last element with ease. By mastering these techniques, you can enhance your coding skills and improve the efficiency of your C# applications.
FAQ
-
What is the difference between using List.Count and LINQ’s Last()?
Using List.Count allows direct access to the last element via its index, while LINQ’s Last() provides a more readable approach that handles empty lists with exceptions. -
Can I use these methods with other data types?
Yes, both methods can be used with any data type, including strings, custom objects, and more. -
What happens if the list is empty?
Accessing an index in an empty list using List.Count will throw an ArgumentOutOfRangeException, while using Last() will result in an InvalidOperationException.
-
Are there performance differences between these methods?
For small lists, the performance difference is negligible. However, LINQ’s Last() may introduce slight overhead due to its function call. -
Can I use these methods in asynchronous programming?
Yes, both methods can be used in asynchronous programming contexts, but ensure proper handling of asynchronous operations.
with two primary methods: using the List.Count property and LINQ’s Last() function. This informative article provides clear examples, explanations, and best practices to help you efficiently access the last element in your C# applications. Whether you’re a beginner or an experienced developer, mastering these techniques will enhance your coding skills.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn