How to Export Data to an Excel File Using C#
-
Export Data to Excel Using
C#
-
Export Data From Array to Excel Using
C#
-
Export Data From DataTable to Excel Using
C#
-
Export Data From JSON to Excel Using
C#
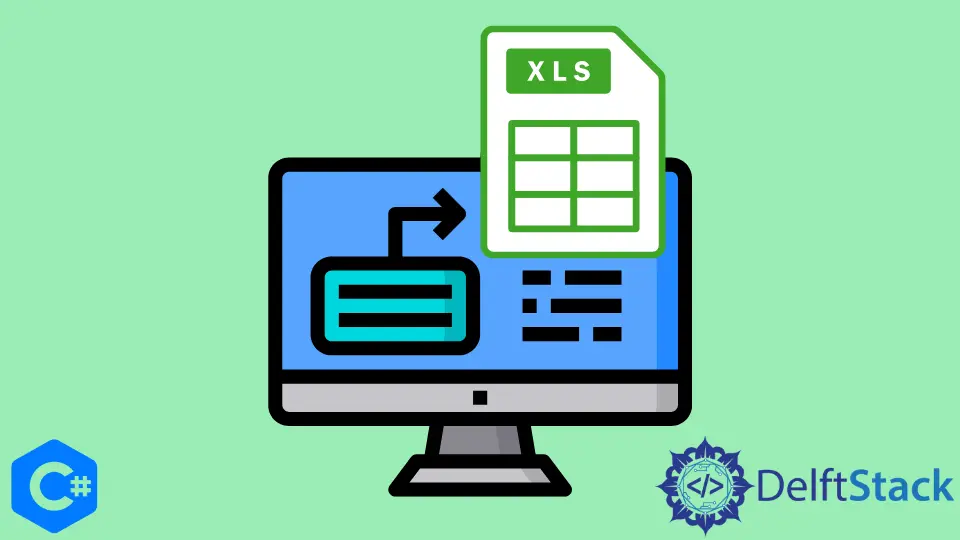
This tutorial will teach how to export data to an Excel file in C#.
Export Data to Excel Using C#
Adding a reference to build an Excel file and exporting it is necessary to move on with this project.
Add the Reference Package
For different operations of excel, we’ll add the ExcelMapper
package. To do that, follow the below steps:
-
Open Visual Studio, create a Console Application and name it.
-
Right-click on the Solution Explorer panel and select Manage NuGet Packages.
-
Now click on the Browse option, search for
ExcelMapper
, and install it.
After adding the package, we’ll write the code of an example program. In the below example, we’ll use random data from students.
Example:
-
To begin, import the following libraries:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Ganss.Excel;
-
Now create a
Student
class havingname
andmarks
data members.public class Student { public String name { get; set; } public double marks { get; set; } }
-
Create another class named
Program
and create theMain()
method in that class.public class Program { public static void Main(string[] args) {} }
-
In the
Main()
method, we’ll create aList<>
type object of theStudent
class.var s = new List<Student> {}
-
This object will be populated with some random
Student
data as shown below:var s = new List<Student> { new Student { name = "M.Zeeshan", marks = 94.0 }, new Student { name = "Shani", marks = 84.0 }, new Student { name = "Saada g", marks = 74.0 }, new Student { name = "Haseeba", marks = 34.0 }, };
-
Then, create an
ExcelMapper
object namedmaper
.ExcelMapper maper = new ExcelMapper();
-
To save the data we just created, we’ll create a sample file in the required path or directory.
var newFile = @"D:\sample.xlsx";
-
Lastly, we’ll save the file using ExcelMapper’s
maper.Save()
function.maper.Save(newFile, s, "ShaniData", true);
Complete Source Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Ganss.Excel;
namespace DatatoExcelbyZeeshan {
public class Program {
public static void Main(string[] args) {
var s = new List<Student> {
new Student { name = "M.Zeeshan", marks = 94.0 },
new Student { name = "Shani", marks = 84.0 },
new Student { name = "Saada g", marks = 74.0 },
new Student { name = "Haseeba", marks = 34.0 },
};
ExcelMapper maper = new ExcelMapper();
var newFile = @"D:\sample.xlsx";
maper.Save(newFile, s, "ShaniData", true);
Console.ReadKey();
}
}
public class Student {
public String name { get; set; }
public double marks { get; set; }
}
}
You’ll get the following output in the sample.xlsx
file:
Export Data From Array to Excel Using C#
Let’s have an example to understand it practically.
Add the Reference Package
For different operations of excel, we’ll add the ExcelMapper
package. To do that, follow the below steps:
-
First, open Visual Studio, create a Console Application and name it.
-
Right-click on the Solution Explorer panel and select Manage NuGet Packages.
-
Now click on the Browse option, search for
Spire.Xls
, and install it.
Example:
-
To begin, import the following libraries:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Spire.Xls;
-
Now, create a new instance of
Workbook
and give it the namewb
.Workbook wb = new Workbook();
-
Include a new
Worksheet
and give it the namews
.Worksheet ws = wb.Worksheets.Add("ShaniArray");
-
Make a new array and give it the name
ProgramSubjectResults
, then add some data.string[,] ProgramSubjectResults = new string[,] { { "Name", "City", "Gpa", "Language" }, { "Zeeshan", "ISB", "3.7", "Flutter Development" }, { "Saad Jaan", "RWP", "4.0", "Full Stack" }, { "Haseeba", "FATA", "3.53", "Andriod Development" } };
-
To include the array on the
Worksheet
, begin with the cell.ws.InsertArray(ProgramSubjectResults, 2, 1); ws.AllocatedRange.AutoFitColumns();
-
Finally, give the array you just created a name and save it as an Excel file.
wb.SaveToFile(@"D:\ArraytoExcel.xlsx", ExcelVersion.Version2016);
Complete Source Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Spire.Xls;
namespace ExportArraytoExcelbyZeeshan {
class Program {
static void Main(string[] args) {
Workbook wb = new Workbook();
wb.Worksheets.Clear();
Worksheet ws = wb.Worksheets.Add("ShaniArray");
string[,] ProgramSubjectResults =
new string[,] { { "Name", "City", "Gpa", "Language" },
{ "Zeeshan", "ISB", "3.7", "Flutter Development" },
{ "Saad Jaan", "RWP", "4.0", "Full Stack" },
{ "Haseeba", "FATA", "3.53", "Andriod Development" } };
ws.InsertArray(ProgramSubjectResults, 2, 1);
ws.AllocatedRange.AutoFitColumns();
wb.SaveToFile(@"D:\ArraytoExcel.xlsx", ExcelVersion.Version2016);
}
}
}
Output:
Export Data From DataTable to Excel Using C#
Let’s see how this can be done using the following example.
Add the Reference Package
For exporting DataTable
to Excel, we’ll add the ExcelMapper
package. To do that, follow the steps we discussed before.
Example:
-
To begin, import the following libraries:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Data; using Spire.Xls;
-
Now, create a new instance of
Workbook
and give it the namewb
.Workbook wb = new Workbook(); wb.Worksheets.Clear();
-
Include a new
Worksheet
instance and give it the namews
.Worksheet ws = wb.Worksheets.Add("DataTableToExcel");
-
Construct an object of type
DataTable
and give it the namedt
.DataTable dt = new DataTable();
-
Add
Roll Number
,Student Name
, andSections
as columns.dt.Columns.Add("Roll Number", typeof(String)); dt.Columns.Add("Student Name", typeof(String)); dt.Columns.Add("Section", typeof(String));
-
Now we need to create an object of the
DataRow
type and give it the namedtr
.DataRow dtr = dt.NewRow();
-
Insert data into the
DataRow
column as shown below.dtr[0] = "130-Arid-3227"; dtr[1] = "Muhammad Zeeshan"; dtr[2] = "Bs(IT)8A"; dt.Rows.Add(dtr); dtr = dt.NewRow(); dtr[0] = "123-Arid-3156"; dtr[1] = "Bubby Shah"; dtr[2] = "Bs(AI)7C"; dt.Rows.Add(dtr); dtr = dt.NewRow();
-
Write the
DataTable
column in the spreadsheet we created.ws.InsertDataTable(dt, true, 1, 1, true);
-
Finally, give the document a name and save it as an Excel file.
wb.SaveToFile(@"C:\DataTableToExcel.xlsx", ExcelVersion.Version2016);
Complete Source Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data;
using Spire.Xls;
namespace ExportDataTableToExcelByZeeshan {
class Program {
static void Main(string[] args) {
Workbook wb = new Workbook();
wb.Worksheets.Clear();
Worksheet ws = wb.Worksheets.Add("DataTableToExcel");
DataTable dt = new DataTable();
dt.Columns.Add("Roll Number", typeof(String));
dt.Columns.Add("Student Name", typeof(String));
dt.Columns.Add("Section", typeof(String));
DataRow dtr = dt.NewRow();
dtr[0] = "130-Arid-3227";
dtr[1] = "Muhammad Zeeshan";
dtr[2] = "Bs(IT)8A";
dt.Rows.Add(dtr);
dtr = dt.NewRow();
dtr[0] = "123-Arid-3156";
dtr[1] = "Bubby Shah";
dtr[2] = "Bs(AI)7C";
dt.Rows.Add(dtr);
dtr = dt.NewRow();
ws.InsertDataTable(dt, true, 1, 1, true);
wb.SaveToFile(@"C:\DataTableToExcel.xlsx", ExcelVersion.Version2016);
}
}
}
Output:
Export Data From JSON to Excel Using C#
The example below will help us understand how to export JSON data to Excel using C# practically.
Add the Reference Package
For the Json
operations of Excel, we’ll add the GemBox.Spreadsheet
and Newtonsoft.Json
packages. To do that, follow the below steps:
-
First, open Visual Studio, create a Console Application and name it.
-
Right-click on the Solution Explorer panel and select Manage NuGet Packages.
-
Now click on the Browse option, search for
GemBox.Spreadsheet
andNewtonsoft.Json
and install it.
Example:
-
To begin, import the following libraries:
using System; using System.Collections.Generic; using GemBox.Spreadsheet; using Newtonsoft.Json;
-
Before using any other class from the
GemBox.Spreadsheet
package, it is necessary to initialize the license using theSpreadsheetInfo.SetLicense()
function.SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
-
Now, create a JSON file with the name
JsonData
and fill it with theStudents
data.string JsonData = @"{ ""0"": { ""Name"": ""Muhammad Zeeshan"", ""Roll"": ""3227"", ""GPA"": 3.11, ""Fyp"": ""Garden Guide System"" }, ""1"" : { ""Name"": ""Bubby Shah"", ""Roll"": ""3265"", ""GPA"": 2.9, ""Fyp"": ""Football Field Management"" }, ""2"" : { ""Name"": ""Haseeb-Ur-Rehman"", ""Roll"": ""3156"", ""GPA"": 3.9, ""Fyp"": ""Plants Recognition"" } }";
-
We’ll deserialize the JSON string after defining the JSON file.
Dictionary<string, Student> shani = JsonConvert.DeserializeObject<Dictionary<string, Student>>(JsonData);
-
Create a new worksheet in Excel and name it
ws
. After that, create the header values for the worksheet.ExcelWorksheet ws = wb.Worksheets.Add("ShaniJSON" + ""); ws.Cells[0, 0].Value = "Student Name"; ws.Cells[0, 1].Value = "Roll No"; ws.Cells[0, 2].Value = "GPA"; ws.Cells[0, 3].Value = "FYP";
-
After the worksheet has been created, we’ll write the deserialized data into the worksheet.
int row = 0; foreach (Student user in shani.Values) { ws.Cells[++row, 0].Value = user.Fname; ws.Cells[row, 1].Value = user.Roll; ws.Cells[row, 2].Value = user.Gpa; ws.Cells[row, 3].Value = user.Fyp; }
-
In the last step, we will save the file as an Excel file in the specified directory.
wb.Save(@"D:\JSON TO Excel by Zeeshan.xlsx");
Complete Source Code:
using System;
using System.Collections.Generic;
using GemBox.Spreadsheet;
using Newtonsoft.Json;
namespace JsontoExcelByZeeshan {
class Program {
static void Main(string[] args) {
SpreadsheetInfo.SetLicense("FREE-LIMITED-KEY");
string JsonData =
@"{
""0"": {
""Name"": ""Muhammad Zeeshan"",
""Roll"": ""3227"",
""GPA"": 3.11,
""Fyp"": ""Garden Guide System""
},
""1"" : {
""Name"": ""Bubby Shah"",
""Roll"": ""3265"",
""GPA"": 2.9,
""Fyp"": ""Football Field Management""
},
""2"" : {
""Name"": ""Haseeb-Ur-Rehman"",
""Roll"": ""3156"",
""GPA"": 3.9,
""Fyp"": ""Plants Recognition""
}
}";
Dictionary<string, Student> shani =
JsonConvert.DeserializeObject<Dictionary<string, Student>>(JsonData);
ExcelFile wb = new ExcelFile();
ExcelWorksheet ws = wb.Worksheets.Add("ShaniJSON" + "");
ws.Cells[0, 0].Value = "Student Name";
ws.Cells[0, 1].Value = "Roll No";
ws.Cells[0, 2].Value = "GPA";
ws.Cells[0, 3].Value = "FYP";
int row = 0;
foreach (Student user in shani.Values) {
ws.Cells[++row, 0].Value = user.Fname;
ws.Cells[row, 1].Value = user.Roll;
ws.Cells[row, 2].Value = user.Gpa;
ws.Cells[row, 3].Value = user.Fyp;
}
wb.Save(@"D:\JSON TO Excel by Zeeshan.xlsx");
}
}
class Student {
[JsonProperty("Name")]
public string Fname { get; set; }
[JsonProperty("Roll")] public string Roll { get; set; }
[JsonProperty("GPA")] public float Gpa { get; set; }
[JsonProperty("Fyp")] public string Fyp { get; set; }
}
}
Output:
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn