How to Exit a Function in C#
-
Use the
break
Statement to Exit a Function inC#
-
Use the
continue
Statement to Exit a Function inC#
-
Use the
goto
Statement to Exit a Function inC#
-
Use the
return
Statement to Exit a Function inC#
-
Use the
throw
Statement to Exit a Function inC#
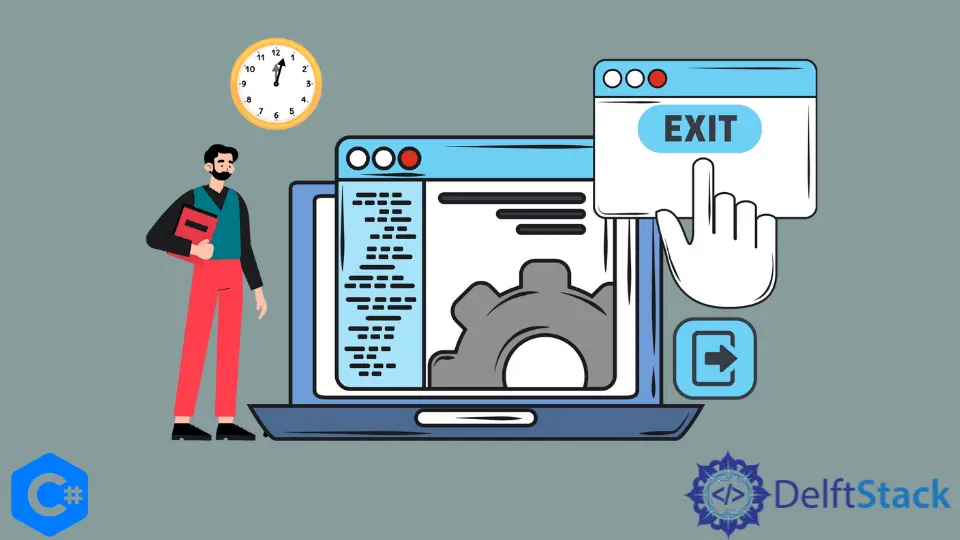
This article will introduce how to exit a function in C#.
Jump statements are generally used to control the flow of program execution. In other words, jump statements unconditionally transfer control from one point to another in the executing program.
Further discussion is available via this reference.
Below are the five statements in C# categorized as Jump statements.
break
statement;continue
statement;goto
statement;return
statement;throw
statement.
Use the break
Statement to Exit a Function in C#
The break
statement stops the loop where it is present. Then, if available, the control will pass to the statement that follows the terminated statement.
If the break
statement is present in the nested loop, it terminates only those loops containing the break statement.
Example:
// C# program to illustrate the
// use of break statement
using System;
class Test {
// Main Method
static public void Main() {
int[] Numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 };
foreach (int number in Numbers) {
// print only the first 10 numbers
if (number > 10) {
break;
}
Console.Write($"{number} ");
}
}
}
Output:
1 2 3 4 5 6 7 8 9 10
Use the continue
Statement to Exit a Function in C#
The continue
statement skips the execution of a block of code when a certain condition is true. Unlike the break
statement, the continue
statement transfers the control to the beginning of the loop.
Below is an example of code using a foreach
method.
// C# program to illustrate the
// use of continue statement
using System;
class Test {
// Main Method
static public void Main() {
int[] Numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 };
foreach (int oddNumber in Numbers) {
// print only the odd numbers 10 numbers
if (oddNumber % 2 == 0) {
continue;
}
Console.Write($"{oddNumber} ");
}
}
}
Output:
1 3 5 7 9 11 13 15 17 19
Use the goto
Statement to Exit a Function in C#
We use the goto
statement to transfer control to a labeled statement in the program. The label must be a valid identifier placed before the goto
statement.
In other words, it forces the execution of the code on the label.
In the example below, the goto
statement forces the execution of case 5.
// C# program to illustrate the
// use of goto statement
using System;
class Test {
// Main Method
static public void Main() {
int age = 18;
switch (age) {
case 5:
Console.WriteLine("5yrs is less than the recognized age for adulthood");
break;
case 10:
Console.WriteLine("Age 10 is still underage");
break;
case 18:
Console.WriteLine("18yrs! You are now an adult and old enough to drink");
// goto statement transfer
// the control to case 5
goto case 5;
default:
Console.WriteLine("18yrs is the recognized age for adulthood");
break;
}
}
}
Output:
18yrs! You are now an adult and old enough to drink
5yrs is less than the recognized age for adulthood
Use the return
Statement to Exit a Function in C#
The return
statement terminates the function execution in which it appears then returns control to the calling method’s result, if available. However, if a function does not have a value, the return
statement is used without expression.
Example:
// C# program to illustrate the
// use of return statement
using System;
class Test {
// Main Method
static public void Main() {
int[] Numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 };
foreach (int number in Numbers) {
// print only the first 10 numbers
if (number > 10) {
return;
}
return;
Console.Write($"{number} ");
}
}
}
Output:
No output
Use the throw
Statement to Exit a Function in C#
Exceptions indicate that an error has occurred or altered a program’s execution. The throw
statement creates an object of a valid Exception class
using the new
keyword.
All Exception classes have the Stacktrace and Message properties.
Note that the valid exception must be derived from the Exception class. Valid Exception class includes ArgumentException
, InvalidOperationException
, NullReferenceException
and IndexOutOfRangeException
.
Further discussion is available via this reference.
Example:
// C# program to illustrate the
// use of throw statement
using System;
class Test {
// Main Method
static public void Main() {
int[] Numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20 };
foreach (int number in Numbers) {
// using try catch block to
// handle the Exception
try {
// print only the first 10 numbers
if (number > 10) {
Console.WriteLine();
throw new NullReferenceException("Number is greater than 10");
}
Console.Write($"{number} ");
} catch (Exception exp) {
Console.WriteLine(exp.Message);
return;
}
}
}
}
Output:
1 2 3 4 5 6 7 8 9 10
Number is greater than 10