How to Calculate Distance Between 2 Points in C#
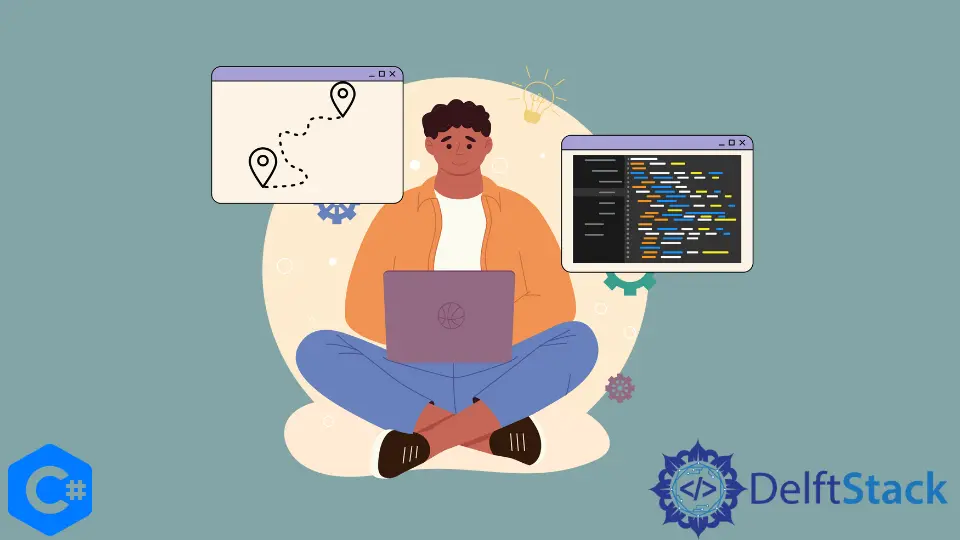
This tutorial will discuss the method for calculating the distance between 2 points in C#.
Calculate Distance Between 2 Points With the Euclidean Distance Formula in C#
The Euclidean distance formula is used to calculate the distance between 2 points. The formula is .
d
is the distance between points, p
and q
, respectively. We can use the Math
class for taking square and square-root of the coordinates in C#. The Math.Pow()
function calculates the square of the number by passing 2
as a parameter. The Math.Sqrt()
function calculates the square root of a number. So, We can apply the Euclidean distance formula with the var distance = Math.Sqrt((Math.Pow(x1 - x2, 2) + Math.Pow(y1 - y2, 2)));
statement in C#. The following code example shows us how to calculate the distance between two points with the Euclidean distance formula in C#.
using System;
namespace measure_distance {
class Program {
static void Main(string[] args) {
double x1, x2, y1, y2;
x1 = 12d;
x2 = 13d;
y1 = 11d;
y2 = 10d;
var distance = Math.Sqrt((Math.Pow(x1 - x2, 2) + Math.Pow(y1 - y2, 2)));
Console.WriteLine(distance);
}
}
}
Output:
1.4142135623731
In the above code, we initialized the x
and y
coordinates of point 1 and point 2. The variables x1
and y1
are the coordinates of point 1, and the variables x2
and y2
are the coordinates of point 2. We calculated the distance between these points with the Euclidean distance formula and displayed the result.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn