Difference Between a Jagged Array and a Multi-Dimensional Array in C#
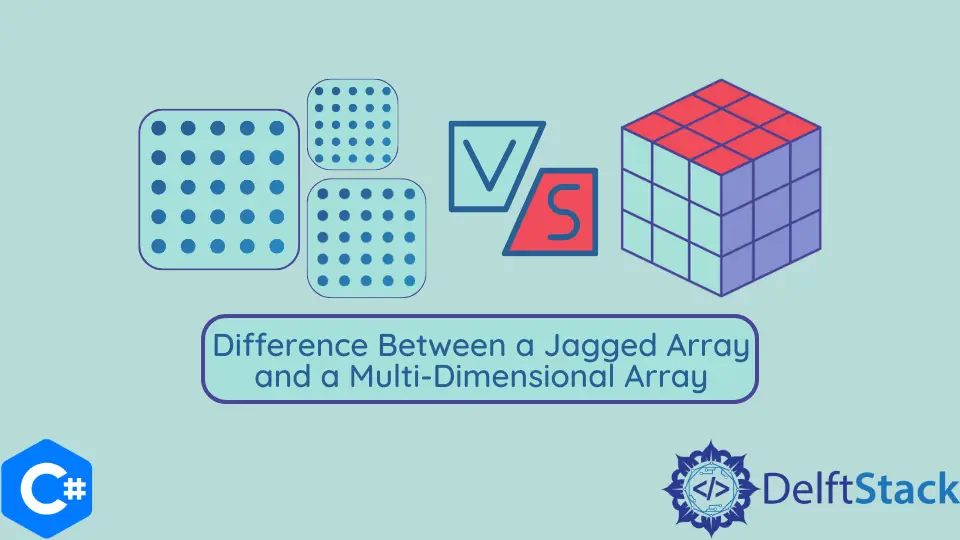
This tutorial will discuss the differences and similarities between a jagged array and a multi-dimensional array in C#.
Jagged Arrays in C#
A jagged array is an array of arrays in C#. It can constitute arrays of different sizes in it. The following code example shows us how we can declare a jagged array in C#.
namespace jagged_array_vs_multidimensional_array {
class Program {
static void Main(string[] args) {
int[][] jaggedArray = new int [3][];
jaggedArray[0] = new int[1];
jaggedArray[1] = new int[2];
jaggedArray[2] = new int[3];
}
}
}
In the above code, we created the jagged array jaggedArray
of size 3, which means that the jaggedArray
is an array of 3 arrays. These 3 arrays are at the index 0
, 1
, and 2
of the jaggedArray
. It is clear from the example that all these arrays are of different sizes.
Multi-Dimensional Arrays in C#
A Multi-dimensional array is a rectangular array in C#. It can only have a fixed number of elements in each dimension. The following code example shows us how we can declare a multi-dimensional array in C#.
namespace jagged_array_vs_multidimensional_array {
class Program {
static void Main(string[] args) {
int[,] mArray = new [3, 3]
}
}
}
In the above code, we created the multi-dimensional array mArray
with the size 3,3
, which means that it has three inner arrays and each of which has a size of 3
elements.
Jagged Arrays vs Multi-Dimensional Arrays in C#
The jagged arrays should be preferred over the conventional multi-dimensional arrays because of their flexibility in C#. For example, if we have to store a person’s hobbies, the preferred approach would be to use a jagged array because not everyone has the same number of hobbies. The same thing goes for interests and many other things.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn