How to Delete a File in C#
- Understanding the File.Delete Method
- Deleting Files with Full Path and Handling Exceptions
- Deleting Files in a Loop
- Best Practices for File Deletion
- Conclusion
- FAQ
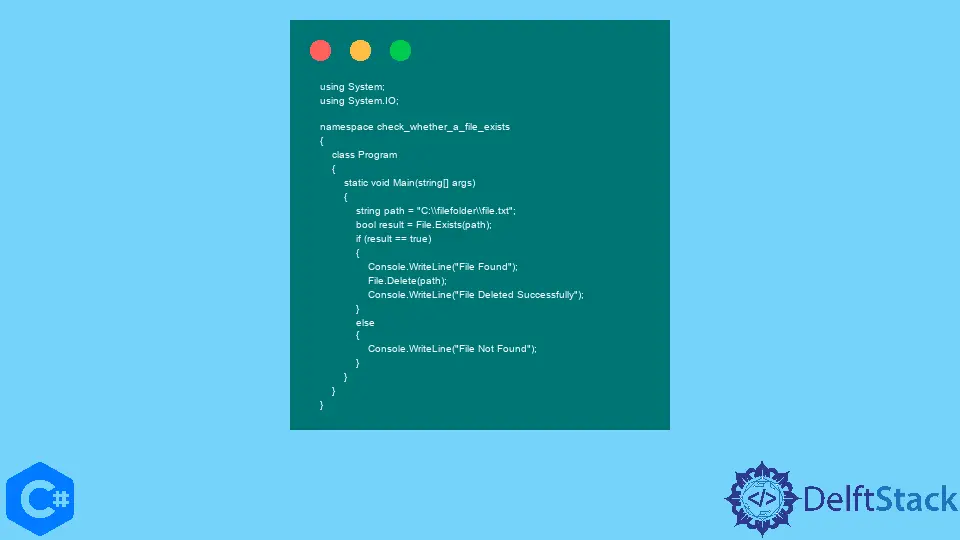
When working with files in C#, you may find yourself needing to delete a file for various reasons, such as cleaning up temporary files or managing user data. The File.Delete(path) function is a straightforward and effective way to accomplish this task.
In this article, we’ll explore how to use this function, along with some best practices and potential pitfalls to watch out for. Whether you’re a beginner or an experienced developer, understanding how to delete files in C# can enhance your programming skills and make your applications more efficient. Let’s dive into the details and discover how to handle file deletion effectively in your C# projects.
Understanding the File.Delete Method
The File.Delete method is a part of the System.IO namespace and allows you to remove a specified file from the file system. The method takes a single parameter, which is the path of the file you want to delete. If the file exists, it will be deleted; if it doesn’t, no action is taken, and no exception is thrown. This makes it a safe choice for file deletion.
Here’s a simple example of how to use the File.Delete method:
csharpCopyusing System;
using System.IO;
class Program
{
static void Main()
{
string filePath = @"C:\temp\example.txt";
try
{
File.Delete(filePath);
Console.WriteLine("File deleted successfully.");
}
catch (IOException ioEx)
{
Console.WriteLine($"An I/O error occurred: {ioEx.Message}");
}
catch (UnauthorizedAccessException uaEx)
{
Console.WriteLine($"Access denied: {uaEx.Message}");
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
}
}
}
Output:
textCopyFile deleted successfully.
In this code snippet, we first specify the file path we want to delete. The try-catch block handles potential exceptions that may arise during the deletion process. The IOException will catch issues related to file access, while UnauthorizedAccessException will handle scenarios where the program does not have permission to delete the file. If the deletion is successful, a confirmation message is printed to the console.
Deleting Files with Full Path and Handling Exceptions
When using the File.Delete method, it’s crucial to provide the full path of the file you want to delete. This ensures that the correct file is targeted, especially if there are multiple files with the same name in different directories.
Here’s an example that illustrates this:
csharpCopyusing System;
using System.IO;
class Program
{
static void Main()
{
string filePath = @"C:\temp\sample.txt";
if (File.Exists(filePath))
{
try
{
File.Delete(filePath);
Console.WriteLine("File deleted successfully.");
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
else
{
Console.WriteLine("File does not exist.");
}
}
}
Output:
textCopyFile deleted successfully.
In this example, we first check if the file exists using the File.Exists method. This is a good practice as it prevents unnecessary exceptions from being thrown when trying to delete a non-existent file. If the file exists, we proceed to delete it inside a try-catch block, which will catch any exceptions that may occur during the deletion process. If the file doesn’t exist, a message is displayed to inform the user.
Deleting Files in a Loop
In some cases, you may need to delete multiple files in a directory. The File.Delete method can be combined with a loop to achieve this. Here’s how you can do it:
csharpCopyusing System;
using System.IO;
class Program
{
static void Main()
{
string directoryPath = @"C:\temp";
try
{
string[] files = Directory.GetFiles(directoryPath);
foreach (string file in files)
{
File.Delete(file);
Console.WriteLine($"Deleted: {file}");
}
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
}
Output:
textCopyDeleted: C:\temp\file1.txt
Deleted: C:\temp\file2.txt
In this example, we retrieve all files in the specified directory using Directory.GetFiles. We then loop through each file and delete it using File.Delete. After each deletion, we print a message indicating which file was deleted. This method is efficient for cleaning up directories, but be cautious when using it to avoid unintended data loss.
Best Practices for File Deletion
While using File.Delete is straightforward, there are some best practices to follow to ensure safe and efficient file management.
-
Always Check for Existence: Before attempting to delete a file, check if it exists. This helps avoid unnecessary exceptions and ensures that your program runs smoothly.
-
Handle Exceptions Gracefully: Implement try-catch blocks to handle potential exceptions that may arise during file deletion. This will help you understand what went wrong and provide feedback to the user.
-
Use Full Paths: Always use the full path to the file you want to delete. This reduces the risk of accidentally deleting the wrong file.
-
Backup Important Files: If you’re working with critical files, consider backing them up before deletion to prevent data loss.
-
Test in a Safe Environment: When developing file management features, test your code in a controlled environment to avoid accidental deletions of important files.
By following these best practices, you can ensure that your file deletion operations are safe and effective.
Conclusion
Deleting files in C# is a simple yet essential task that can significantly impact your application’s performance and user experience. The File.Delete method provides an efficient way to remove files from the file system, and by understanding how to use it properly, you can enhance your coding skills. Remember to check for file existence, handle exceptions gracefully, and follow best practices to ensure that your file management operations are safe and reliable. Armed with this knowledge, you can confidently manage files in your C# applications.
FAQ
-
How do I delete a read-only file in C#?
To delete a read-only file, you must first change its attributes using the File.SetAttributes method. Set the file attribute to normal before deleting it. -
What happens if I try to delete a file that is currently open?
If you attempt to delete a file that is currently open in another program, you may encounter an IOException indicating that the file is in use. -
Can I delete multiple files at once in C#?
Yes, you can delete multiple files using a loop, as shown in the examples above, by iterating over an array of file paths.
-
Is it possible to recover a deleted file in C#?
Once a file is deleted using File.Delete, it is permanently removed from the file system and cannot be recovered through standard methods. Use file recovery software if you need to recover deleted files. -
What permissions do I need to delete a file in C#?
You must have the necessary permissions to delete a file. If you lack the required permissions, an UnauthorizedAccessException will be thrown.
using the File.Delete method. This comprehensive guide covers best practices, exception handling, and examples to help you manage files effectively in your applications. Whether you’re a beginner or an experienced developer, mastering file deletion in C# is essential for efficient file management.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn