How to Declare a Constant Array in C#
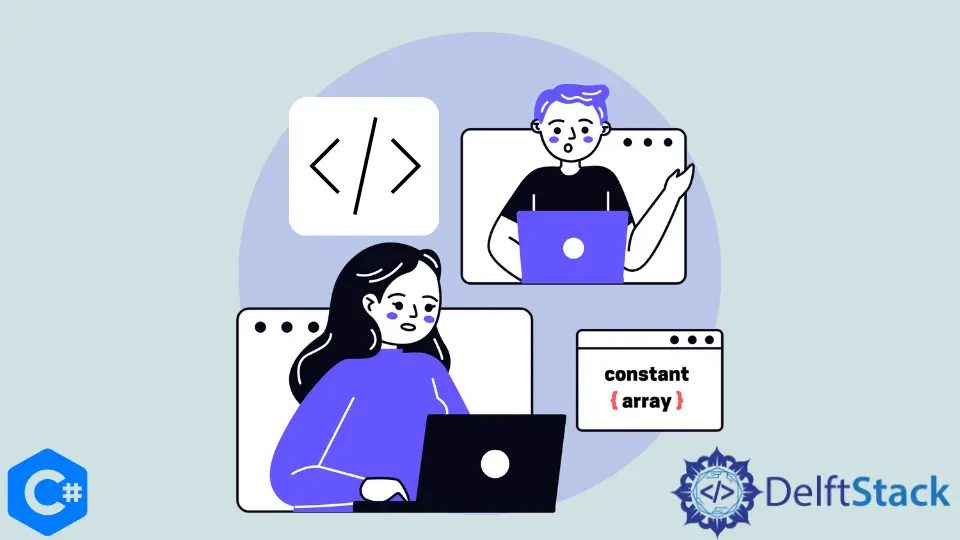
This tutorial will discuss methods to declare a constant array in C#.
Declare a Constant Array With the readonly
Keyword in C#
In C#, we cannot declare a constant array with the following syntax.
public const string[] Values = { "Value1", "Value2", "Value3", "Value4" };
This will give a compiler error because the const
keyword is used for values that are known at the compile-time. But an array does not get initialized during compile-time, so the array’s value is not known during the compile-time.
This error can be avoided by using the readonly
keyword in C#. The readonly
keyword is used to specify that a variable’s value cannot be modified after initialization. The following code example shows us how we can declare a constant array with the readonly
keyword in C#.
using System;
namespace constant_array {
class Program {
public static readonly string[] Values = { "Value1", "Value2", "Value3" };
static void Main(string[] args) {
foreach (var Value in Values) {
Console.WriteLine(Value);
}
}
}
}
Output:
Value1
Value2
Value3
In the above code, we declared the constant array Values
with the readonly
keyword in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn