URL Encoding in C#
-
Use Simple
ASCII
to Encode Characters inC#
-
Different URL Encoding Options in
C#
-
Other Encoding Functions Present in
System.Web
inC#
-
Use
Uri.HexEscape()
to Modify Single Characters in a String inC#
-
Use Temboo to Encode URL in
C#
- Conclusion
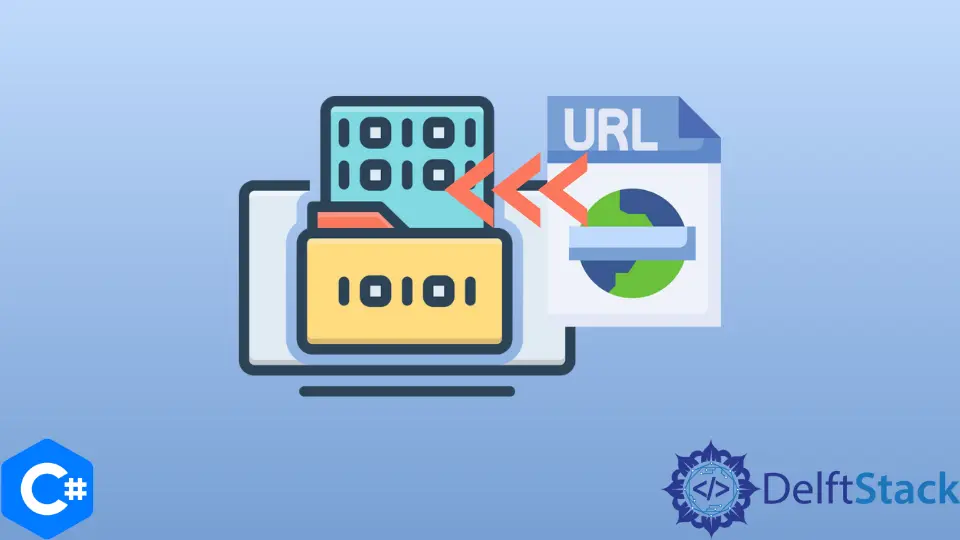
This article will discuss how to encode a URL in C#.
Use Simple ASCII
to Encode Characters in C#
It represents the DECIMAL
value of each character on the keyboard. Hence, this DECIMAL
value can be substituted for one of the characters in a string and then encoded efficiently.
using System;
class Program {
static void Main(string[] args) {
string s = "DelftStack";
string ascii_convert = "";
foreach (char c in s) {
ascii_convert += System.Convert.ToInt32(c);
}
Console.WriteLine("The converted ascii string is: " + ascii_convert);
}
}
Notice that the System.Convert.ToInt32()
tends to present the DECIMAL
representation of a character in a string. Once the character is converted, its value is then appended to the new string ASCII_CONVERT
, which now represents the encoded string.
Rather than using DECIMAL
, you can even choose BINARY
, OCTAL
, or HEXADECIMAL
conversions for your encoding needs.
Different URL Encoding Options in C#
In C#, we have a variety of encoding functions to use. Many convert characters to different notations, such as URL
, URI
, HEX
etc.
Let’s go forward and write a function to convert a simple URL to these various notations.
First, add the System.Web
namespace to our code file. Then call the function to encode the string into URL format that will convert the /, ., "
in the string to its ASCII (hexadecimal)
representation.
using System;
using System.Web;
class Program {
static void Main(string[] args) {
Console.WriteLine(HttpUtility.UrlEncode("https://www.google.com"));
}
}
Output:
https%3a%2f%2fwww.google.com
Many times such a representation will cause issues in recognition by other systems. It is recommended to make sure to encode only not-so-important information.
In the above example, we would always want google to be accessible. However, a representation in another format may render it void and unrecognizable.
Hence it is necessary to call encoding on terms that belong to the URL but are user-specific. Let’s say now that I search "World"
on Google.
The URL would become:
https://www.google.com/search?q=World
The q
here stands for Query. Use the following code to encode it.
Example Code:
using System;
using System.Web;
class Program {
static void Main(string[] args) {
Console.WriteLine("https://www.google.com/search" + HttpUtility.UrlEncode("?q=World"));
}
}
Output:
https://www.google.com/search%3fq%3dWorld
Other Encoding Functions Present in System.Web
in C#
Example Code:
using System;
using System.Web;
class Program {
static void Main(string[] args) {
Console.WriteLine("URL ENCODE UNICODE: " +
HttpUtility.UrlEncodeUnicode("https://www.google.com/search?q=World"));
Console.WriteLine("URL PATH ENCODE: " +
HttpUtility.UrlPathEncode("https://www.google.com/search?q=World"));
Console.WriteLine("URI ESCAPE DATASTRING: " +
Uri.EscapeDataString("https://www.google.com/search?q=World"));
Console.WriteLine("URL ESCAPE URI STRING: " +
Uri.EscapeUriString("https://www.google.com/search?q=World"));
Console.WriteLine("HTML ENCODE: " +
HttpUtility.HtmlEncode("https://www.google.com/search?q=World"));
Console.WriteLine("HTML ATTRIBUTE ENCODE: " +
HttpUtility.HtmlAttributeEncode("https://www.google.com/search?q=World"));
}
}
Output:
URL ENCODE UNICODE: https%3a%2f%2fwww.google.com%2fsearch%3fq%3dWorld
URL PATH ENCODE: https://www.google.com/search?q=World
URI ESCAPE DATASTRING: https%3A%2F%2Fwww.google.com%2Fsearch%3Fq%3DWorld
URL ESCAPE URI STRING: https://www.google.com/search?q=World
HTML ENCODE: https://www.google.com/search?q=World
HTML ATTRIBUTE ENCODE: https://www.google.com/search?q=World
Notice that the HttpUtility.UrlPathEncode()
, Uri.EscapeUriString()
, HttpUtility.HtmlEncode()
and HttpUtility.HtmlAttributeEncode()
tend to present the same results as the unencoded string.
Use Uri.HexEscape()
to Modify Single Characters in a String in C#
Let’s create a function to randomly modify characters in a URL string in C# and then return the encoded string.
static string encoded_url(string param) {
Random rd = new Random();
int rand_num = rd.Next(0, param.Length - 1);
param = param.Remove(rand_num, 1);
param = param.Insert(rand_num, Uri.HexEscape(param[rand_num]));
return param;
}
Call the in the main as follows.
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 1: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 2: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 3: " +
encoded_url("https://www.google.com/search?q=World"));
Complete Source Code:
using System;
using System.Web;
class Program {
static string encoded_url(string param) {
Random rd = new Random();
int rand_num = rd.Next(0, param.Length - 1);
param = param.Remove(rand_num, 1); /// returning the
param = param.Insert(rand_num, Uri.HexEscape(param[rand_num]));
return param;
}
static void Main(string[] args) {
// string s = Console.ReadLine();
// string ascii_convert = "";
// foreach (char c in s)
//{
// ascii_convert += System.Convert.ToInt32(c);
// }
// Console.WriteLine("The converted ascii string is: " + ascii_convert);
// Console.ReadKey();
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 1: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 2: " +
encoded_url("https://www.google.com/search?q=World"));
Console.WriteLine("URI HEX ESCAPE for 'GOOGLE', called at 3: " +
encoded_url("https://www.google.com/search?q=World"));
}
}
Output:
URI HEX ESCAPE for 'GOOGLE', called at 1: https://www.%6Foogle.com/search?q=World
URI HEX ESCAPE for 'GOOGLE', called at 2: https://www.go%67gle.com/search?q=World
URI HEX ESCAPE for 'GOOGLE', called at 3: https://www.google%63com/search?q=World
See how characters are converted to their HEX
representations at random positions. You can also use this URI.HexEscape()
function in a loop to modify the whole string or the desired characters.
URLENCODE
stays best for browser compatibility and use. Also, to clarify the use of URI
and HTTPUTILITY
, remember that URI
is inherited from the SYSTEM
class, already present within the client C# code.
If you are not using SYSTEM.WEB
, you are better off using URI
and then calling the encoding functions.
However, using SYSTEM.WEB
as a namespace shouldn’t be trouble in many instances. In case your project is compiled in .NET FRAMEWORK CLIENT PROFILE
, it isn’t much you can do except copy the code files into a new project.
The .NET FRAMEWORK CLIENT PROFILE
will not be able to use SYSTEM.WEB
. Simple .NET FRAMEWORK 4
must be utilized when naming SYSTEM.WEB
.
Use Temboo to Encode URL in C#
An API called TEMBOO tends to encode a URL perfectly.
Example Code:
// the NAMESPACES TO USE BEFORE UTILIZING THEIR CODE
using Temboo.Core;
using Temboo.Library.Utilities.Encoding;
TembooSession session = new TembooSession("ACCOUNT_NAME", "APP_NAME", "APP_KEY");
URLEncode urlEncodeChoreo = new URLEncode(session);
urlEncodeChoreo.setText("https://www.google.com/search?q=");
URLEncodeResultSet urlEncodeResults = urlEncodeChoreo.execute();
Console.WriteLine(urlEncodeResults.URLEncodedText);
Conclusion
While encoding, make sure there are illegal characters that will be converted. If you don’t, switch to the first solution and create a mixed-function that will use SWITCH
cases to determine which parts to encode and which not to encode.
In the end, encoding is up to the user. A function that might switch illegal characters and ASCII’s would need a similar decoder on the opposite end.
Hence, using it in a system with a global encoder/decoder for URL/Strings is best.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub