How to Toggle a Boolean Variable in C#
-
Toggle a Boolean Variable in
C#
-
Use the
!
Operator to Toggle a Boolean Variable inC#
-
Why You Should Not Use the
^
(XOR) Operator to Toggle Boolean Variables inC#
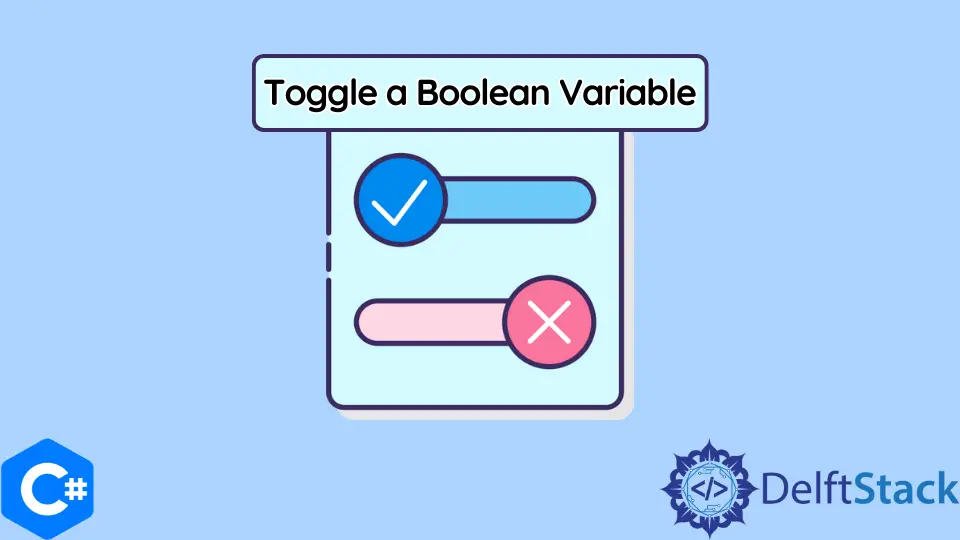
This article teaches us how we can toggle boolean variables in C#.
Toggle a Boolean Variable in C#
Toggling a Boolean variable means changing its value between true
and false
. One simple way to do that is to use the following code, which can be made into a function as well:
bool flag = false;
if (flag == false) {
flag = true;
} else {
flag = false;
}
In case you were to make a function, then you could do something like this:
using System;
class Program {
static void toggle(ref bool flag) {
if (flag == false) {
flag = true;
} else {
flag = false;
}
}
static void Main(String[] args) {
bool flag = false;
toggle(ref flag);
Console.WriteLine(flag);
}
}
We have used the ref
keyword to ensure the flag
parameter is passed by reference, so it is changed inside the function.
The output will be as follows:
True
Now let’s look at ways we can omit these long lines of code and toggle them into much shorter lines.
Use the !
Operator to Toggle a Boolean Variable in C#
One way to do this toggling in simple syntax is to use the !
operator, which converts the value into its opposite.
So we can write something as follows:
flag = !flag;
If the flag
is true
, it will become False
. If it is false
, it will become True
.
The output will be the same:
True
The full code has been documented below:
using System;
class Program {
static void Main(string[] args) {
bool flag = false;
flag = !flag;
Console.WriteLine(flag);
}
}
Why You Should Not Use the ^
(XOR) Operator to Toggle Boolean Variables in C#
The XOR operator tends to convert only the value of the bool variable if it is true
to false
. However, it won’t work for opposite cases where the value is already false
.
Let’s take a look at an example below:
using System;
class Program {
static void Main(string[] args) {
bool flag = true;
flag ^= flag;
Console.WriteLine(flag);
}
}
If we were to run the above, the output would become:
False
But if the flag
was set to false
, putting an XOR on it won’t change it to True
.
using System;
class Program {
static void Main(string[] args) {
bool flag = false;
flag ^= flag;
Console.WriteLine(flag);
}
}
The output is now:
False
Why? Well, 1
is true
, and 0
is false
. Putting an XOR with the flag
being true
, the result would become 0
or false
.
But if we put the XOR with the flag
being false
, the result would still stay 0
. If you remember the XOR table, that’s how it works:
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub