TextBox New Line in C#
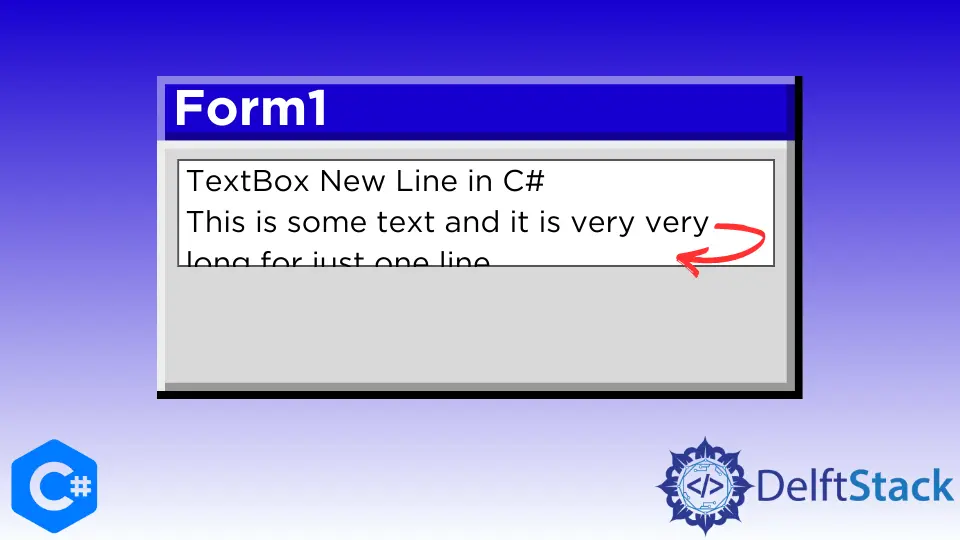
This tutorial will discuss the method to add a new line to a text box in C#.
TextBox New Line With the TextBox.Multiline
Property in C#
The TextBox.Multiline
property stores a boolean value in it. The value of the TextBox.Multiline
property determines whether the control is a multiline text box or not. The value of this property can only be true
or false
. The true
value indicates that this specific text box can have multiple lines in it. The false
value indicates that this specific text box cannot have multiple lines in it. The following code example shows us how to add a new line to a text box with the TextBox.Multiline
property in C#.
private void button1_Click(object sender, EventArgs e) {
string longtext = "This is some text and it is very very long for just one line";
textBox1.Text = longtext;
textBox1.AppendText(Environment.NewLine);
textBox1.Multiline = true;
}
Output:
In the above code, we inserted a very long string to the textBox1
with textBox1.Text = longtext
and added a new line to the textBox1
after that with textBox1.AppendText(Environment.NewLine)
. After that, we specified that the textBox1
is a multiline text box by setting the value of the textBox1.Multiline
property to true
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn