How to Send a Simple SSH Command in C#
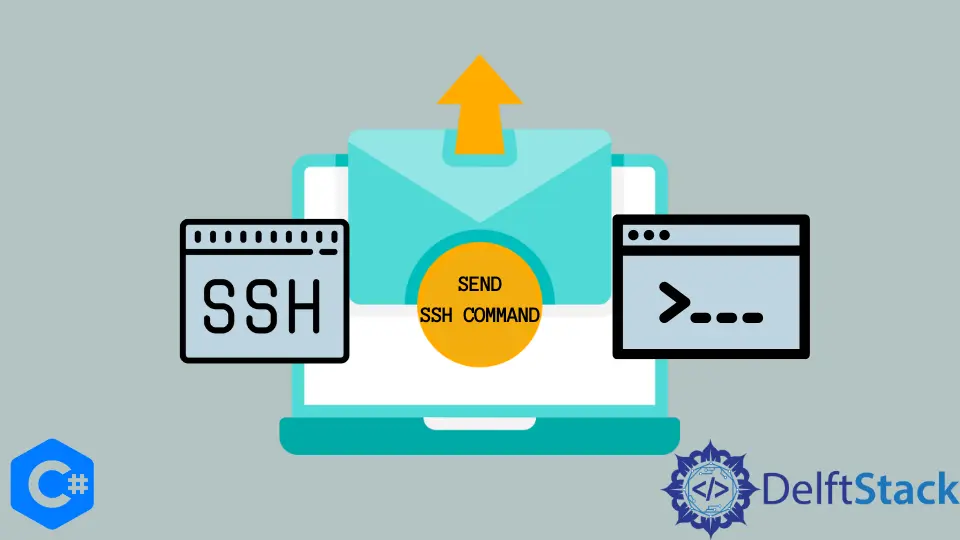
This guide will discuss how to send a simple SSH command in C#
.
Send a Simple SSH Command in C#
First, we recommend you use the SSH.Net library because it features excellent documentation and some samples that you can use.
To run a simple command, all you need to do is import a method from this library and pass the required IP, username, and password. After that, you can import all functions you need to - for instance, using a command to go into some folder on the connected device.
Take a look at the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace SSHCLIENT {
class Program {
static void Main(string[] args) { // demoip //username //password
using (var myclient = new SshClient("127.0.01", "username", "Password")) {
myclient.Connect();
myclient.RunCommand("cd /home/delftstack/blogs"); // TO Run Code....................
myclient.Disconnect();
}
}
}
}
As you can see, we are using Connect()
with the variable after passing the required data, including IP, username, and password. We run a command using the RunCommand()
and give the address.
Later, we are just disconnecting from the device. This is just to run an SSH command, and the actual connectivity involves software like Putty.
Learn more about SSH here.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn