How to Split String by String in C#
- Using String.Split Method
- Using Regular Expressions
- Using StringBuilder for Performance
- Conclusion
- FAQ
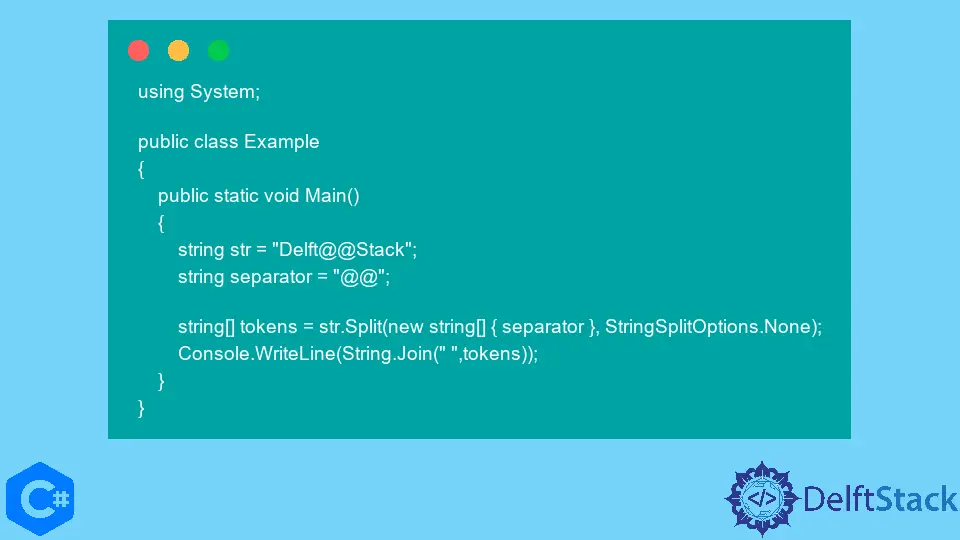
Splitting strings is a common task in programming, especially when you need to parse data or manipulate text. In C#, you often need to split a string based on another string, which can be a bit tricky if you’re used to simpler methods.
This tutorial will guide you through different approaches to achieve this in C#. By the end, you’ll have a solid understanding of how to split strings effectively, allowing you to handle text data with ease. Whether you’re working on a personal project or a professional application, mastering string manipulation is a valuable skill. Let’s dive in!
Using String.Split Method
One of the most straightforward ways to split a string in C# is by using the String.Split
method. While this method typically splits a string using a character or an array of characters, it can also be adapted to split by a string. To achieve this, you’ll need to combine it with the String.IndexOf
and String.Substring
methods.
Here’s how you can do it:
using System;
class Program
{
static void Main()
{
string input = "apple|banana|cherry|date";
string delimiter = "|";
string[] result = SplitByString(input, delimiter);
foreach (var item in result)
{
Console.WriteLine(item);
}
}
static string[] SplitByString(string input, string delimiter)
{
return input.Split(new string[] { delimiter }, StringSplitOptions.None);
}
}
Output:
apple
banana
cherry
date
In this example, we define a method called SplitByString
, which takes two parameters: the input string and the delimiter string. The Split
method is called with the delimiter wrapped in an array, allowing for string-based splitting. The StringSplitOptions.None
option ensures that all elements are included in the result, even if they are empty.
Using Regular Expressions
Another powerful method for splitting strings in C# is using Regular Expressions (Regex). This method is particularly useful when you need to split a string based on a more complex pattern rather than a fixed string. Regex allows you to define patterns that can match various string formats, which can be quite handy.
Here’s an example of how to use Regex for string splitting:
using System;
using System.Text.RegularExpressions;
class Program
{
static void Main()
{
string input = "apple|banana|cherry|date";
string delimiter = @"\|";
string[] result = Regex.Split(input, delimiter);
foreach (var item in result)
{
Console.WriteLine(item);
}
}
}
Output:
apple
banana
cherry
date
In this code, we use the Regex.Split
method to split the input string based on the delimiter defined as a regex pattern. The pattern @"\|"
matches the pipe character. This method is particularly useful for more complex delimiters or when you want to split based on multiple characters or patterns.
Using StringBuilder for Performance
When dealing with large strings or needing to perform multiple splits, using a StringBuilder
can enhance performance. This approach allows for efficient manipulation of the string data without creating multiple intermediate string instances.
Here’s how you can implement this:
using System;
using System.Text;
class Program
{
static void Main()
{
string input = "apple|banana|cherry|date";
string delimiter = "|";
string[] result = SplitWithBuilder(input, delimiter);
foreach (var item in result)
{
Console.WriteLine(item);
}
}
static string[] SplitWithBuilder(string input, string delimiter)
{
StringBuilder sb = new StringBuilder();
var list = new System.Collections.Generic.List<string>();
for (int i = 0; i < input.Length; i++)
{
if (input.Substring(i, delimiter.Length) == delimiter)
{
list.Add(sb.ToString());
sb.Clear();
i += delimiter.Length - 1; // Skip the delimiter
}
else
{
sb.Append(input[i]);
}
}
list.Add(sb.ToString()); // Add the last segment
return list.ToArray();
}
}
Output:
apple
banana
cherry
date
In this example, we create a StringBuilder
to accumulate characters until we encounter the delimiter. When we find the delimiter, we add the accumulated string to a list and clear the StringBuilder
for the next segment. This method is efficient and avoids creating multiple string instances during the splitting process.
Conclusion
Splitting strings by another string in C# can be accomplished through various methods, each with its own advantages. Whether you choose the straightforward String.Split
method, the flexibility of Regular Expressions, or the performance benefits of using StringBuilder
, understanding these techniques will enhance your string manipulation skills. As you work on your projects, consider the context and requirements to choose the best method for your needs. Happy coding!
FAQ
-
What is the best method to split a string in C#?
The best method depends on your specific needs. For simple cases,String.Split
is effective. For complex patterns, consider using Regular Expressions. -
Can I split a string by multiple delimiters in C#?
Yes, you can use an array of strings withString.Split
to specify multiple delimiters. -
Is using Regular Expressions for string splitting slower than other methods?
Regex can be slower than simpler methods likeString.Split
, especially for large strings or simple delimiters. Use it when you need pattern matching. -
How do I handle empty strings in the result of a split?
You can useStringSplitOptions.RemoveEmptyEntries
withString.Split
to exclude empty entries from the result. -
Can I split a string in C# without using any built-in methods?
Yes, you can manually iterate through the string and construct the result, similar to theStringBuilder
method shown above.
#. Explore various methods such as String.Split, Regular Expressions, and StringBuilder for efficient string manipulation in C#. Learn how to handle complex patterns and improve performance while working with strings in your C# applications.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn