How to Send HTML-Formatted Email Using C#
-
Create Windows Form to Send HTML-Formatted Email Using
C#
-
Write the Code to Send HTML-Formatted Email Using
C#
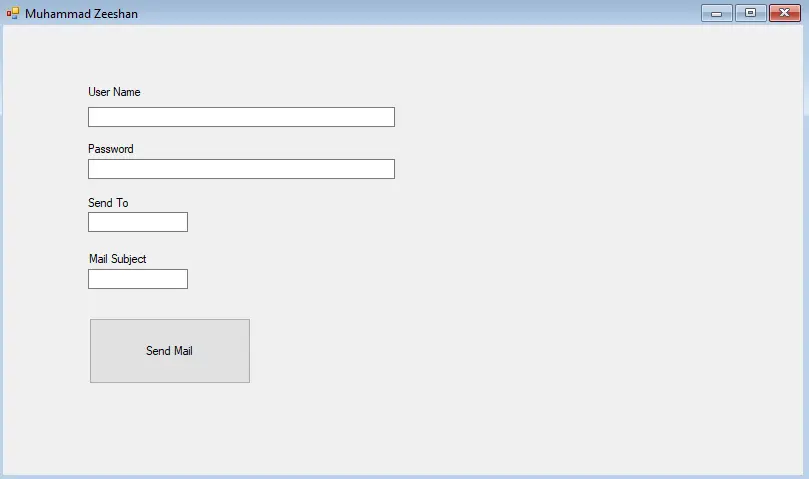
This article will teach you how to send HTML-formatted emails using C# in ASP.Net.
Sending emails over SMTP may be tested with either your current Gmail account or a whole new account. The Gmail Mailer client can be used for communication.
Create Windows Form to Send HTML-Formatted Email Using C#
-
Add a label and textbox for
User Name
. This will be the sender’s username. -
Add a label and textbox for
Password
. It will be the sender account’s password. -
Add a label and textbox for
Sendto
. It will be the receiver’s mail address. -
Add a label and textbox for
Subject
or title. -
Lastly, create a button named
Send Mail
.The complete form will look like this:
Write the Code to Send HTML-Formatted Email Using C#
Below are the necessary steps to write the code to send HTML-formatted email using C#:
-
To begin, import the following libraries:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; using System.Net.Mail;
-
Create
MailMessage
object andSmtpClient
object.MailMessage mail = new MailMessage(); SmtpClient smtp = new SmtpClient("smtp.gmail.com");
-
Now, assign values of properties like sender, receiver, subject, body, etc.
mail.To.Add(sendtotxt.Text); mail.Subject = subjecttxt.Text; var htmlbody = "Here your can write your html"; mail.Body = htmlbody; smtp.Port = 587; smtp.Credentials = new System.Net.NetworkCredential(usertxt.Text, passtxt.Text);
-
Enable
SSL
, and send the message.smtp.EnableSsl = true; smtp.Send(mail);
Complete Source Code
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Net.Mail;
namespace EmailExample {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void sendbtn_Click(object sender, EventArgs e) {
try {
MailMessage mail = new MailMessage();
SmtpClient smtp = new SmtpClient("smtp.gmail.com");
mail.From = new MailAddress(usertxt.Text);
mail.To.Add(sendtotxt.Text);
mail.Subject = subjecttxt.Text;
var htmlbody = "Here your can write your html";
mail.Body = htmlbody;
smtp.Port = 587;
smtp.Credentials = new System.Net.NetworkCredential(usertxt.Text, passtxt.Text);
smtp.EnableSsl = true;
smtp.Send(mail);
MessageBox.Show("Mail Sent!");
} catch (Exception ex) {
MessageBox.Show(ex.Message);
}
}
}
}
Output:
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn