How to Remove Quotes From String in C#
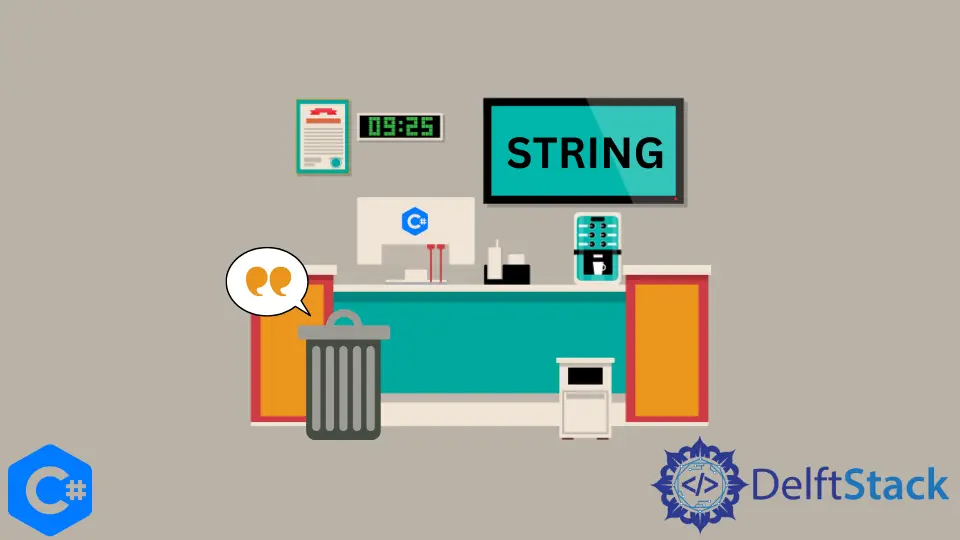
This tutorial will introduce the method to remove quotes from a string variable in C#.
Remove Quotes From String With the String.Replace()
Function in C#
The String.Replace(x, y)
function is used to replace all occurrences of the string x
with the string y
inside the main string in C#. The String.Replace()
function has a string return type. If we want to remove quotes from a string, we can replace it will an empty string. We can specify that we want to replace the double quotes with "\""
. The following code example shows us how we can remove quotes from a string with the String.Replace()
function in C#.
using System;
namespace remove_quotes_from_string {
class Program {
static void method1() {
string str = "He said \"This is too soon for me\" and left.";
Console.WriteLine(str);
string newstr = str.Replace("\"", "");
Console.WriteLine(newstr);
}
static void Main(string[] args) {
method1();
}
}
}
Output:
He said "This is too soon for me" and left.
He said This is too soon for me and left.
In the above code, we removed the quotes from the string variable str
by replacing the quotes with an empty string with the str.Replace()
function in C#. We used the \
escape character to write a double quote inside another pair of double quotes.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn