Random Number in a Range in C#
- Understanding the Random Class in C#
- Generating Random Integers Using Next()
- Generating Multiple Random Numbers
- Seeding Random Number Generation
- Conclusion
- FAQ
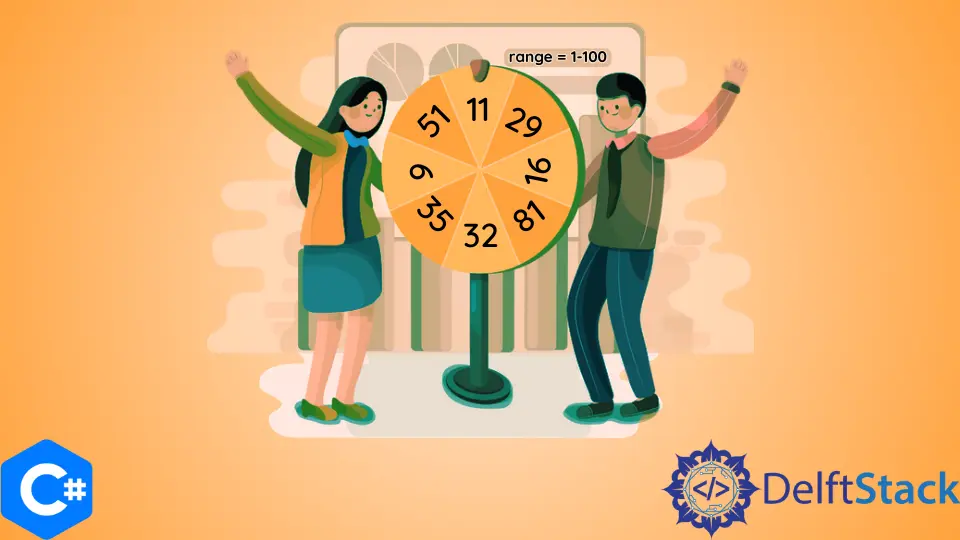
Generating random numbers is a common requirement in programming, especially in applications that involve simulations, games, or any scenario where unpredictability is key. In C#, the Random
class provides a straightforward way to generate random numbers.
This article will dive into how to generate a random integer within a specified range using the Next()
method. By the end of this guide, you’ll have a solid understanding of how to utilize this method effectively in your C# projects. Whether you’re a beginner looking to grasp basic concepts or an experienced developer seeking a refresher, this article will provide valuable insights and practical examples to enhance your coding toolkit.
Understanding the Random Class in C#
Before we jump into generating random numbers, it’s essential to understand the Random
class in C#. This class is part of the System
namespace and is designed to generate random numbers. When you create an instance of the Random
class, you can specify a seed value, which influences the sequence of random numbers generated. If no seed is provided, the current time is used as the default seed, ensuring a different sequence of numbers each time you run your program.
Here’s how you can create a simple instance of the Random
class:
Random random = new Random();
This line initializes a new instance of the Random
class, allowing you to start generating random numbers right away. The Random
class provides several methods, but the most commonly used one for generating integers is the Next()
method.
Generating Random Integers Using Next()
The Next()
method is the primary way to generate random integers in C#. It can be used in several ways, depending on whether you want a random integer within a specific range or just a random integer. The most common usage involves specifying a minimum and maximum value.
Here’s how you can use the Next()
method to generate a random integer within a specified range:
Random random = new Random();
int minValue = 1;
int maxValue = 100;
int randomNumber = random.Next(minValue, maxValue);
Output:
example of output
In this example, we create a new Random
object and define a range between 1 and 100. The Next(minValue, maxValue)
method then generates a random integer that is greater than or equal to minValue
and less than maxValue
. This means that the possible output could be any integer from 1 to 99.
Understanding how to set the minimum and maximum values is crucial. If you set minValue
to 5 and maxValue
to 10, the possible outputs will be 5, 6, 7, 8, or 9. This flexibility allows you to tailor the random numbers to suit your specific needs.
Generating Multiple Random Numbers
Sometimes, you might need to generate multiple random numbers at once. You can achieve this by using a loop in conjunction with the Next()
method. This approach is particularly useful in scenarios like games, where you might want to create an array of random scores or random positions.
Here’s an example of generating multiple random integers:
Random random = new Random();
int[] randomNumbers = new int[5];
for (int i = 0; i < randomNumbers.Length; i++)
{
randomNumbers[i] = random.Next(1, 100);
}
Output:
example of output
In this code, we create an array called randomNumbers
that can hold five integers. By using a for loop, we fill this array with random integers between 1 and 100. Each iteration calls the Next()
method to generate a new random integer, which is then stored in the array.
This method is highly effective for generating random numbers for various applications, such as creating randomized game elements or simulating random events in a program. The use of arrays allows you to manage and manipulate multiple random values easily.
Seeding Random Number Generation
Seeding is an important concept in random number generation. By providing a seed value when creating your Random
instance, you can produce a predictable sequence of random numbers. This is particularly useful for debugging or testing, where you might want to reproduce the same set of random numbers.
Here’s how to seed your random number generator:
Random random = new Random(42);
int randomNumber = random.Next(1, 100);
Output:
example of output
In this example, we initialize the Random
object with a seed of 42. This means that every time you run this code, it will produce the same sequence of random numbers. This predictability can be beneficial when you need to ensure consistent results across different runs of your application.
However, it’s important to note that using the same seed will yield the same sequence of random numbers. If you want different results on each run, you can omit the seed or use a variable seed based on the current time or other changing parameters.
Conclusion
Generating random numbers in C# is a straightforward process with the Random
class and its Next()
method. Whether you need a single random integer, multiple random values, or a predictable sequence of numbers, C# provides the tools necessary to achieve your goals. By understanding how to effectively use the Random
class, you can enhance your applications with dynamic and unpredictable elements, making them more engaging and realistic. Remember to consider the implications of seeding and how it affects the randomness of your outputs. Happy coding!
FAQ
-
How do I generate a random number between two specific values in C#?
You can use theNext(minValue, maxValue)
method of theRandom
class, whereminValue
is inclusive andmaxValue
is exclusive. -
Can I generate floating-point random numbers in C#?
Yes, you can use theNextDouble()
method of theRandom
class to generate a random floating-point number between 0.0 and 1.0. -
What happens if I create multiple instances of the Random class?
Creating multiple instances in quick succession can lead to the same random numbers being generated because they may be seeded with the same value (like the current time). -
Is it safe to use Random for cryptographic purposes?
No, theRandom
class is not suitable for cryptographic purposes. For secure random number generation, use theRNGCryptoServiceProvider
class. -
How can I ensure that my random numbers are unique?
You can store generated numbers in a collection and check for duplicates before adding a new number.