Random Int in C#
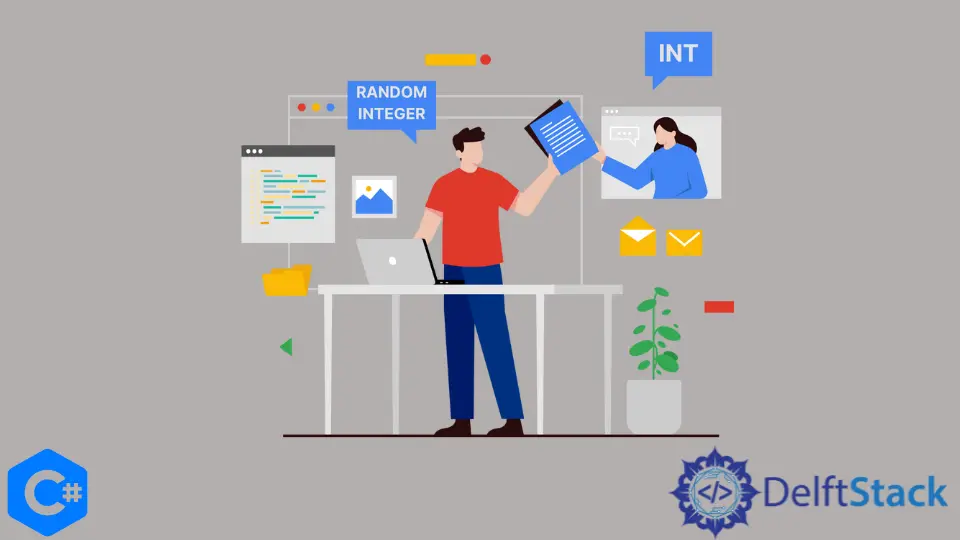
This article will introduce a method to generate a random integer number in C#.
Use the Next()
Method to Generate a Random Integer Number in C#
We will use the Next()
method to generate a random integer number in C#. This method generates a random integer. It has three overloads. To use this method, we will have to create an object of the Random
class. The correct syntax to use this method is as follows.
Random myObject = new Random();
myObject.Next();
This method returns a random integer.
The program below shows how we can use the Next()
method to generate a random integer.
using System;
public class Program {
public static void Main() {
Random myObject = new Random();
int ranNum = myObject.Next();
Console.WriteLine("The Random Number is: " + ranNum);
}
}
Output:
The Random Number is: 880084995
The random number generated by the function is too large. We can pass a number to this function so that it will return a random number less than that number.
The program below shows how we can use the Next()
method to generate a random integer less than a specific number.
using System;
public class Program {
public static void Main() {
Random myObject = new Random();
int ranNum = myObject.Next(100);
Console.WriteLine("The Random Number is: " + ranNum);
}
}
Output:
The Random Number is: 96
We can also set a range. The function will return the random number between that range.
The program below shows how we can use the Next()
method to generate a random integer within a range.
using System;
public class Program {
public static void Main() {
Random myObject = new Random();
int ranNum = myObject.Next(100, 150);
Console.WriteLine("The Random Number is: " + ranNum);
}
}
Output:
The Random Number is: 145