How to Make HTTP POST Web Request in C#
-
Make an HTTP POST Web Request With the
WebClient
Class inC#
-
Make an HTTP POST Web Request With the
HttpWebRequest
Class inC#
-
Make an HTTP POST Web Request With the
HttpClient
Class inC#
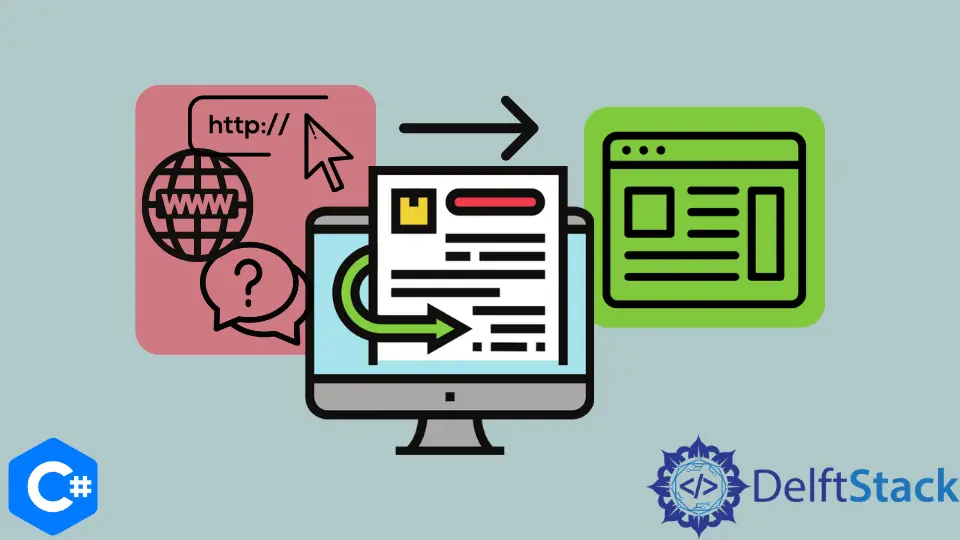
This tutorial will discuss methods to make an HTTP POST Web Request in C#.
Make an HTTP POST Web Request With the WebClient
Class in C#
The WebClient
class provides many methods to send data to and receive data from a URL in C#. We can make HTTP POST requests by using the WebClient.UploadValues(url, values)
function of the WebClient
class in C#. The following code example shows us how we can make a simple HTTP POST Web Request with the WebClient
class in C#.
using System.Net;
using System.Collections.Specialized;
var wb = new WebClient() var data = new NameValueCollection();
string url = "www.example.com" data["username"] = "myUser";
data["password"] = "myPassword";
var response = wb.UploadValues(url, "POST", data);
In the above code, we create the web client wb
for sending data to the url
. We initialize the data
variable that we want to send to the url
. We make the HTTP POST Web Request to the url
by specifying POST
inside the parameters of the wb.UploadValues(url, "POST", data)
function. The response from the url
is saved inside the reponse
variable.
Make an HTTP POST Web Request With the HttpWebRequest
Class in C#
The HttpWebRequest
class provides methods to interact directly with the server using HTTP protocol in C#. We can use the HttpWebRequest.Method = "POST"
property to specify that an HTTP web request is a POST request in C#. The following code example shows us how to make a simple HTTP POST web request with the HttpWebRequest
class in C#.
using System.Net;
using System.Text;
using System.IO;
string url = "http://www.example.com" var request = (HttpWebRequest)WebRequest.Create(url);
var postData = "username=" + Uri.EscapeDataString("myUser");
postData += "&password=" + Uri.EscapeDataString("myPassword");
var data = Encoding.ASCII.GetBytes(postData);
request.Method = "POST";
request.ContentType = "application/x-www-form-urlencoded";
request.ContentLength = data.Length;
using (var stream = request.GetRequestStream()) {
stream.Write(data, 0, data.Length);
}
var response = (HttpWebResponse)request.GetResponse();
In the above code, we created the HTTP web request request
to the url
. We specified that the request
is a POST request with the request.Method = "POST"
property. We initialized the data postData
that we want to send to the url
with the Uri.EscapeDataString()
function and encoded the data into bytes variable data
. We specify the length of the data that we want to send to the url
with the request.ContentLength = data.Length
property. In the end, we created a stream
and wrote data using the stream.Write()
function. We captured the response from the url
with the request.GetResponse()
function and stored it in the HTTPWebResponse
class object response
.
Make an HTTP POST Web Request With the HttpClient
Class in C#
The HttpClient
class provides methods for sending HTTP requests and receiving HTTP responses in C#. We can make an HTTP POST web request with the HttpClient.PostAsync(url, data)
function where url
is the URL, and the data
is the data that we want to send to the url
. The following code example shows us how to make a simple HTTP POST Request with the HttpClient
class.
using System.Net.Http;
readonly HttpClient client = new HttpClient();
var values =
new Dictionary<string, string> { { "username", "myUser" }, { "password", "myPassword" } };
string url = "http://www.example.com" var data = new FormUrlEncodedContent(values);
var response = await client.PostAsync(url, data);
In the above code, we created the read-only HTTP client client
and initialized the url
. We stored the data that we want to send to the url
in the dictionary values
. We then converted the values
to the application/x-www-form-urlencoded
type with the FormUrlEncodedContent()
function. In the end, we made an HTTP POST web request with the client.PostAsync(url, data)
function and stored the response from the url
inside the response
variable.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn