How to Popup A Message in C#
- Using MessageBox.Show Method
- Customizing the MessageBox
- Displaying Error Messages
- Using Async/Await with MessageBox
- Conclusion
- FAQ
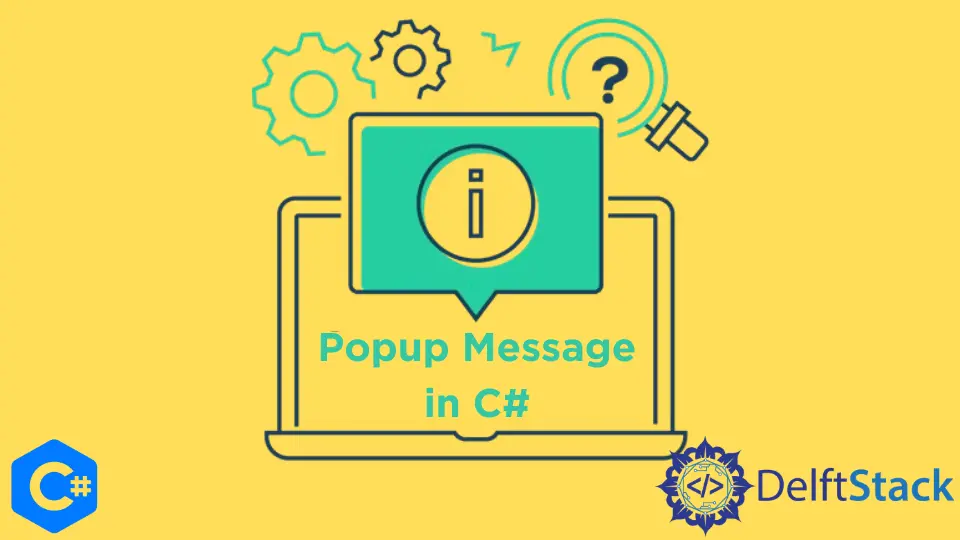
When developing applications in C#, you might find yourself needing to communicate information to users. One effective way to do this is by using popup message windows. The MessageBox class in C# provides a simple yet powerful way to display messages, alerts, warnings, or confirmations to users. Whether you want to inform users of an error, prompt them for confirmation, or simply share information, the MessageBox is your go-to solution.
In this article, we will explore various methods to create popup messages in C#, complete with code examples and explanations to help you implement them seamlessly in your projects.
Using MessageBox.Show Method
The most straightforward way to display a popup message in C# is by using the MessageBox.Show
method. This method allows you to create a message box with a title, a message, and various buttons for user interaction. Here’s how you can do it:
using System;
using System.Windows.Forms;
class Program
{
static void Main()
{
MessageBox.Show("Hello, this is a popup message!", "Popup Title");
}
}
Output:
Hello, this is a popup message!
In this example, we start by including the System.Windows.Forms
namespace, which contains the MessageBox class. The MessageBox.Show
method takes two parameters: the message you want to display and the title of the popup window. When executed, this code will display a simple message box with the specified text and title. This method is particularly useful for displaying information without requiring any additional user input.
Customizing the MessageBox
While the basic MessageBox.Show
method is effective, you may want to customize the popup further by adding buttons and icons. This can make your message box more informative and user-friendly. Here’s an example of how to customize the MessageBox:
using System;
using System.Windows.Forms;
class Program
{
static void Main()
{
DialogResult result = MessageBox.Show(
"Do you want to continue?",
"Confirmation",
MessageBoxButtons.YesNo,
MessageBoxIcon.Question);
if (result == DialogResult.Yes)
{
MessageBox.Show("You chose to continue.");
}
else
{
MessageBox.Show("You chose to cancel.");
}
}
}
Output:
Do you want to continue?
In this code, the MessageBox.Show
method is enhanced with additional parameters. The third parameter specifies the buttons to display, in this case, “Yes” and “No,” while the fourth parameter sets an icon indicating a question. The method returns a DialogResult
that you can use to determine which button the user clicked. Based on the user’s choice, you can display another message box, providing a more interactive experience. This customization is essential for scenarios where user confirmation is required.
Displaying Error Messages
Another common use of popup messages is to display error messages. This is crucial for applications that need to handle exceptions or user input errors gracefully. Here’s how you can implement an error message popup:
using System;
using System.Windows.Forms;
class Program
{
static void Main()
{
try
{
int result = DivideNumbers(10, 0);
MessageBox.Show("Result: " + result.ToString());
}
catch (DivideByZeroException)
{
MessageBox.Show("Error: Division by zero is not allowed.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
static int DivideNumbers(int a, int b)
{
return a / b;
}
}
Output:
Error: Division by zero is not allowed.
In this example, we attempt to divide two numbers, which can lead to a DivideByZeroException
. When this exception occurs, we catch it and display an error message using the MessageBox.Show
method. The parameters include an error message, a title, and specify that only an “OK” button should be shown, along with an error icon. This approach is effective for informing users of issues without crashing the application, ensuring a smoother user experience.
Using Async/Await with MessageBox
In modern C# applications, especially those that are asynchronous, you may want to display a MessageBox without blocking the main thread. Using async and await keywords can help with this. Here’s how you can achieve this:
using System;
using System.Threading.Tasks;
using System.Windows.Forms;
class Program
{
static async Task Main()
{
await ShowMessageAsync("This is an async message box.", "Async Popup");
}
static Task ShowMessageAsync(string message, string title)
{
return Task.Run(() => MessageBox.Show(message, title));
}
}
Output:
This is an async message box.
In this code, we define an asynchronous method ShowMessageAsync
that wraps the MessageBox.Show
call in a task. This allows the main thread to remain responsive while the message box is displayed. By using await
, we ensure that the program waits for the message box to close before proceeding. This technique is particularly useful in applications with a user interface where you want to avoid freezing the UI during long-running operations.
Conclusion
Creating popup messages in C# is a straightforward process thanks to the versatile MessageBox class. Whether you need to display simple information, prompt for user confirmation, or handle errors, the MessageBox provides a user-friendly way to communicate with your application’s users. By customizing the MessageBox and using asynchronous programming patterns, you can enhance user experience and maintain application responsiveness. With the examples provided in this article, you should now have a solid foundation for implementing popup messages in your C# applications.
FAQ
-
How can I customize the buttons in a MessageBox?
You can customize the buttons by passing theMessageBoxButtons
enumeration as a parameter to theMessageBox.Show
method. -
Can I display icons in a MessageBox?
Yes, you can display icons by using theMessageBoxIcon
enumeration in theMessageBox.Show
method. -
Is it possible to create a non-blocking MessageBox?
Yes, you can create a non-blocking MessageBox using async and await, allowing the main thread to remain responsive. -
What happens if I don’t handle exceptions in my application?
If you don’t handle exceptions, your application may crash or behave unexpectedly, leading to a poor user experience. -
Can I use MessageBox in console applications?
Yes, you can use MessageBox in console applications, but you need to reference theSystem.Windows.Forms
namespace.
using the MessageBox class. This comprehensive guide covers various methods, including customization options, error handling, and asynchronous message boxes. Learn to enhance user interaction in your applications with practical code examples and detailed explanations. Perfect for beginners and experienced developers alike, this article provides everything you need to know about displaying popup messages in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn