How to Play a Sound in C#
-
Use
System.Media.SoundPlayer
to Play a Sound inC#
-
Use the
SystemSounds.Play
Method to Play a Sound inC#
- Embed the Windows Media Player Control in a C# Solution to Play a Sound
-
Add the
Microsoft.VisualBasic
Reference on the C# Project to Play a Sound
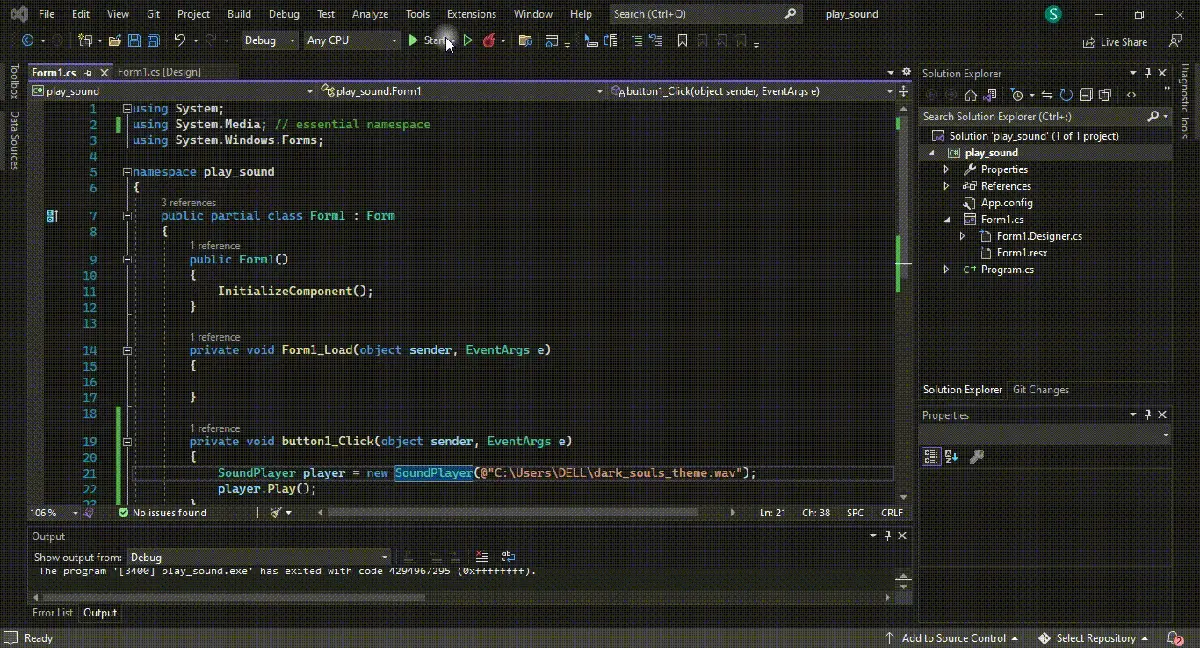
This tutorial will teach you how to play audio files or sounds in Windows Forms using C#. You can’t deny sound’s importance in immersing the audience in game development or technical applications.
C# programming offers four primary ways to implement audio files or sounds to your Windows applications, each useful and practical in unique ways. With such a rich platform as .NET
for multiplayer, you can easily create custom sound generators for WAV
or other format files.
Furthermore, it is possible to build a byte-based buffer of the wave files (sound files) directly in memory using C# as the WPF cones with excellent built-in multiplayer (audio) support.
The important thing to understand here is the SoundPlayer
class which plays sound based on WAV
or wave format which makes it not extremely useful instead, the MediaPlayer
and MediaElement
classes allow the playback of MP3 files.
Use System.Media.SoundPlayer
to Play a Sound in C#
The SoundPlayer
class can help play a sound at a given path at run time as it requires the file path, name, and a reference to the System.Media
namespace.
You can declare a reference to the System.Media
namespace and use SoundPlayer _exp = new SoundPlayer(@"path.wav");
without mentioning the namespace at the object’s declaration.
Afterward, execute it using the Play()
method with something like _exp.Play();
. Most importantly, file operations like playing a sound should be enclosed within appropriate or suitable structured exception handling blocks for increased efficiency and understanding in case something goes wrong; the path name is malformed, read-only sound file path, null
path, and invalid audio file path name can lead to errors and require different exception classes.
using System;
using System.Media; // essential namespace for using `SoundPlayer`
using System.Windows.Forms;
namespace play_sound {
public partial class Form1 : Form {
// Initialize form components
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
// object creation and path selection
SoundPlayer player = new SoundPlayer(@"C:\Users\DELL\dark_souls_theme.wav");
// apply the method to play sound
player.Play();
}
}
}
Output:
Use the SystemSounds.Play
Method to Play a Sound in C#
The functionality (SystemSounds
class offers) is one of the simplest ways of implementing sound in your C# applications in addition to its ability to offer different system sounds with a single line of code; SystemSounds.Beep.Play();
.
However, there are some limitations to using the SystemSounds
class as you can only get access to five different system sounds, including; Asterisk
, Beep
, Exclamation
, Hand
, and Question
, and in case, you disable system sound in Windows, this method will not work, and all the sounds will be replaced with silence.
On the other hand, it makes it extremely easy to play sounds the same way your Windows does, and it’s always a good choice that your C# application respects the user’s choice of silence.
Declare a reference to the System.Media
in your C# project or use something like System.Media.SystemSounds.Asterisk.Play();
to play a sound.
using System;
using System.Media; // essential namespace for `SystemSounds`
using System.Windows.Forms;
namespace play_sound {
public partial class Form1 : Form {
// initialize components
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
SystemSounds.Asterisk.Play(); // you can use other sounds in the place of `Asterisk`
}
}
}
Output:
The Play()
method plays the sound asynchronously associated with a set of Windows OS sound-event types. You cannot inherit the SystemSounds
class, which provides static methods to access system sounds.
It is a high-level approach that enables C# applications to use different system sounds in typical scenarios that help these applications to seamlessly fit into the Windows environment. It’s possible to customize the sounds in the Sound
control panel so that each user profile can override these sounds.
Embed the Windows Media Player Control in a C# Solution to Play a Sound
It is possible to add the Windows Media Player control using Microsoft Visual Studio to play a sound in C#. The Windows Media Player is in the VS Toolbox, and to use its functionality in your C# application, first, add its components to your Windows Form.
Install the latest version of Windows Media Player SDK on your system and access it using your VS toolbox by customizing it from the COM
components to add the Windows Media Player
option. The default name of Windows Media Player in Visual Studio is axWindowsMediaPlayer1
, and you can change it to something more rememberable.
Add the Windows Media Player ActiveX
control to your C# project and implement it in a button-click event. Furthermore, you can choose two modes: an Independent
mode, analogous to the media opened through the Open
method, and a Clock
mode, a media target corresponding timeline and clock entries in the Timing tree, which controls the playback.
using System;
using System.Windows.Media; // essential namespace
using System.Windows.Forms;
namespace play_sound {
public partial class Form1 : Form {
private MediaPlayer audio_player = new MediaPlayer();
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
OpenFileDialog dialog_audio = new OpenFileDialog();
dialog_audio.Filter = "MP3 files (*.mp3)|*.mp3|All files (*.*)|*.*";
if (dialog_audio.ShowDialog() == DialogResult.OK) {
audio_player.Open(new Uri(dialog_audio.FileName));
audio_player.Play();
}
}
private void button2_Click(object sender, EventArgs e) {
// to pause audio
audio_player.Pause();
}
private void button3_Click(object sender, EventArgs e) {
// to resume the paused audio
audio_player.Play();
}
}
}
Output:
Most importantly, the MediaPlayer
class inherits Windows Media Player technology to play audio files and system sound in several modern formats. With its help, you can control the playback process of the sound file mentioned in or carried by the MediaPlayer
instance.
Add the Microsoft.VisualBasic
Reference on the C# Project to Play a Sound
The Audio
class is incredibly famous in Visual Basic for playing a sound, and you can benefit from it by using it in C# by including the Visual Basic bindings in your application. However, there is a C#-specific version of the Audio
class called the SoundPlayer
class, but it does not allow easy byte-based buffer building of the wave directly in memory.
Add the Microsoft.VisualBasic
reference to your C# project as an initial step and create an object from the Audio
class like static Audio my_obj = new Audio();
and add the sound file address like my_exp.Play("m:\\path.wav", AudioPlayMode.WaitToComplete);
.
You can customize or set the AudioPlayMode
by yourself, which allows you to customize the playback time of the sound.
using System;
using System.Windows.Forms;
using Microsoft.VisualBasic; // essential namespace
using Microsoft.VisualBasic.Devices; // essential namespace
namespace play_sound {
public partial class Form1 : Form {
static Audio audio_player = new Audio();
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
audio_player.Play(@"C:\Users\DELL\dark_souls_theme.wav", AudioPlayMode.WaitToComplete);
}
}
}
Output:
It is a good practice to use the Background
or BackgroundLoop
tool to resume the other tasks while the audio continues to play in the background.
It is optional; however, you can create custom sounds using nothing more than the C# code by learning the uncompressed WAV file and can create the sound directly in your system’s memory.
This tutorial taught you how to effectively play a sound file in C#.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub