How to Override Properties in Subclasses in C#
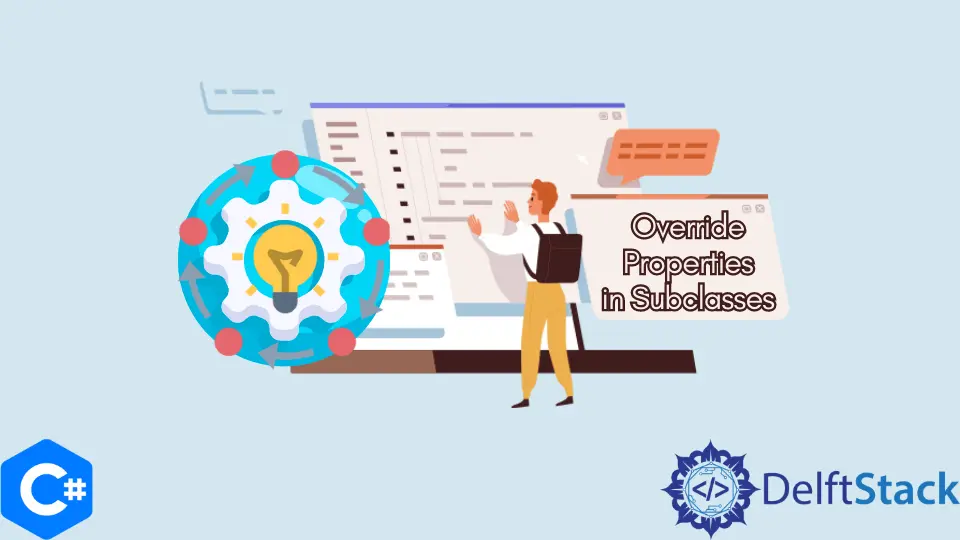
This article will teach you how to use C# to override fields or properties in subclasses. Let’s have an overview of overriding in C#.
Overview of Overriding in C#
Overriding allows a child class to implement an already present method in the parent class. This ability was previously only available to the parent class.
Since the subclass contains a method with a similar name to the one in the base class, the implementation in the subclass is prioritized over the one in the base class.
For example, let’s say we have an abstract base class and want to define a field or property with a unique value in each class inherited from this parent class. And we want to specify it in the base class so that we may refer to it in a method that belongs to the base class.
Use the abstract
Property to Override Properties in Subclasses in C#
Using an abstract
property, which inherited classes can then override, is the optimal approach for the above-case situation. This has several advantages, including that it is enforced, clean, and can be overridden.
abstract class Tree {
abstract public int MyLeaf { get; set; }
}
But it seems a little strange to return a hard-coded value rather than encapsulating a field, and doing so requires a few more lines of code rather than just one. We are required to declare a body for the set
operation, but this is of little significance, and there is probably a technique to get around it that we’re unfamiliar with.
class Root : Tree {
public override int MyLeaf {
get { return 1; }
set {}
}
}
Complete Example Code:
abstract class Tree {
abstract public int MyLeaf { get; set; }
}
class Root : Tree {
public override int MyLeaf {
get { return 1; }
set {}
}
}
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn