How to Open a Folder Using Process.Start in C#
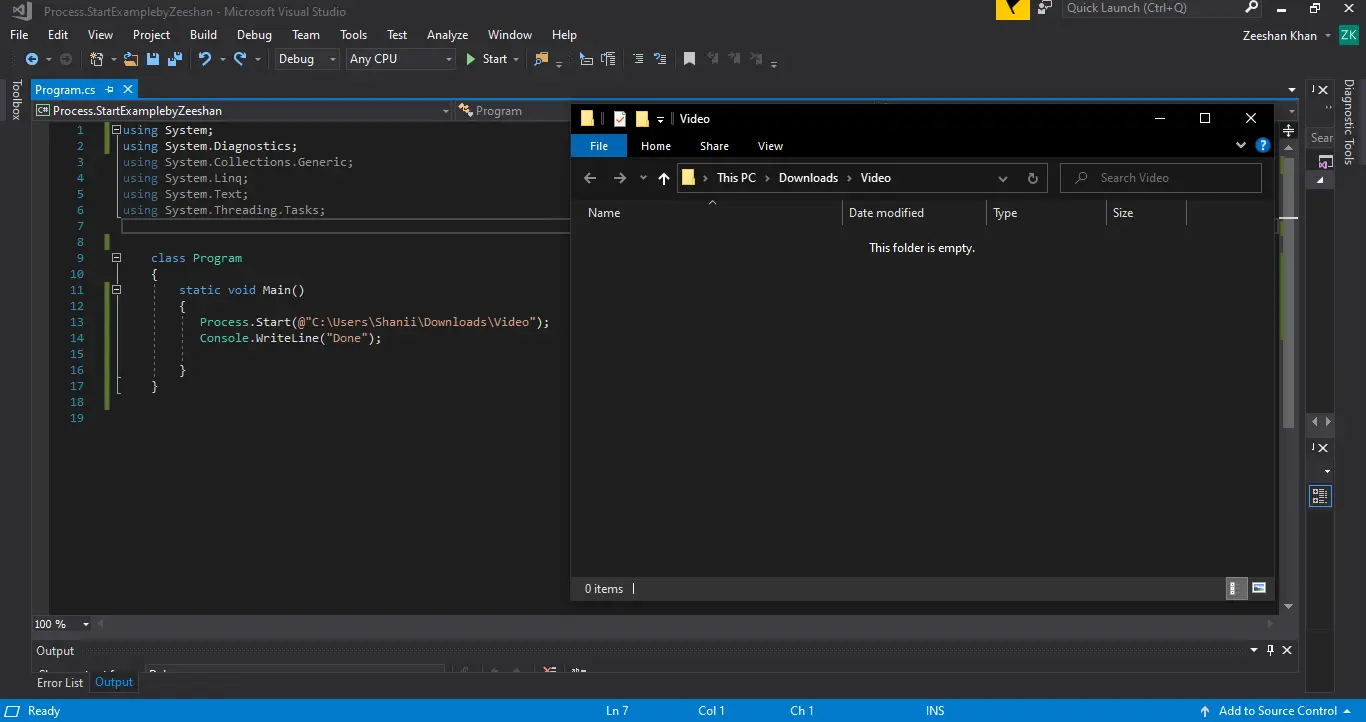
This article shows the steps necessary to open a folder using the Process.Start
function in C#.
the Process
Class in C#
The Process
allows developers to access both local and remote processes and start and stop processes running on the local system.
When a process is launched, a set of values defined by the term ProcessStartInfo
are brought into play. The System.Diagnostics
namespace is where you’ll find the Process
class.
Open a Folder Using Process.Start
in C#
Let’s have an example to understand open explorer or any specific folder. In the below example, we’ll open the Videos
folder, which is located in the Downloads
folder.
Follow the below steps to start explorer with Process.Start
.
-
Firstly, we have to import the following libraries.
using System; using System.Diagnostics; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
-
Let’s create a class called
Program
. Inside theProgram
class, we’ll make theMain()
function.class Program { static void Main() {} }
-
Now, we’ll pass the folder path to the
Process.Start()
function, as shown below.Process.Start(@"C:\Users\Shanii\Downloads\Video"); Console.WriteLine("Folder Opened");
Complete Source Code:
using System;
using System.Diagnostics;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
class Program {
static void Main() {
Process.Start(@"C:\Users\Shanii\Downloads\Video");
Console.WriteLine("Folder Opened");
}
}
Output:
The specified folder will be opened by executing the above example.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn