How to Implement OCR in a C# Project
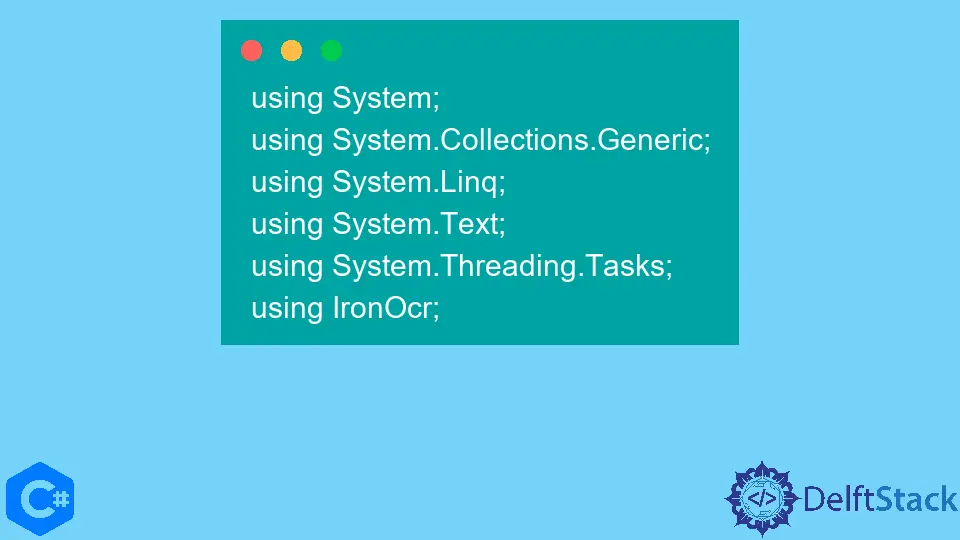
Today’s article will discuss how to implement OCR in a project using the C# programming language.
Add NuGet
Reference Package
For optical character recognition
or OCR operations, we’ll add the IronOcr
package. To do that, follow the below steps.
-
Open
Visual Studio
, create aConsole Application
, and name it. -
Right-click on the
Solution Explorer
panel and SelectManage NuGet Packages
. -
Now click on the
Browse
option, search forIronOcr
and install it.
Implement OCR in C#
The following example of the Tesseract 5 API will allow us to better understand OCR, a process that enables the conversion of picture data into text.
To begin, import the following libraries:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using IronOcr;
First, a new object for the Iron Tesseract
should be created and given the name ocrbyZeeshan
.
var ocrbyZeeshan = new IronTesseract();
We need to construct an object for the OcrInput
variable, allowing us to add one or more image files.
using (var inputfile = new OcrInput()) {}
We are utilizing the OcrInput
object method AddImage
. The function wants us to give the accessible image path.
using (var inputfile = new OcrInput()) {
inputfile.AddImage(@"Shani.png");
var Result = ocrbyZeeshan.Read(inputfile);
Console.WriteLine(Result.Text);
Console.ReadKey();
}
The image we used, named Shani.png
, is below.
Complete Source Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using IronOcr;
namespace ImplementOCRbyZeeshan {
class Program {
static void Main(string[] args) {
var ocrbyZeeshan = new IronTesseract();
ocrbyZeeshan.Language = OcrLanguage.English;
ocrbyZeeshan.Configuration.TesseractVersion = TesseractVersion.Tesseract5;
using (var inputfile = new OcrInput()) {
inputfile.AddImage(@"Shani.png");
var Result = ocrbyZeeshan.Read(inputfile);
Console.WriteLine(Result.Text);
Console.ReadKey();
}
}
}
}
Output:
My Name is Muhammad Zeeshan. Let's code.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn