The nameof Expression in C#
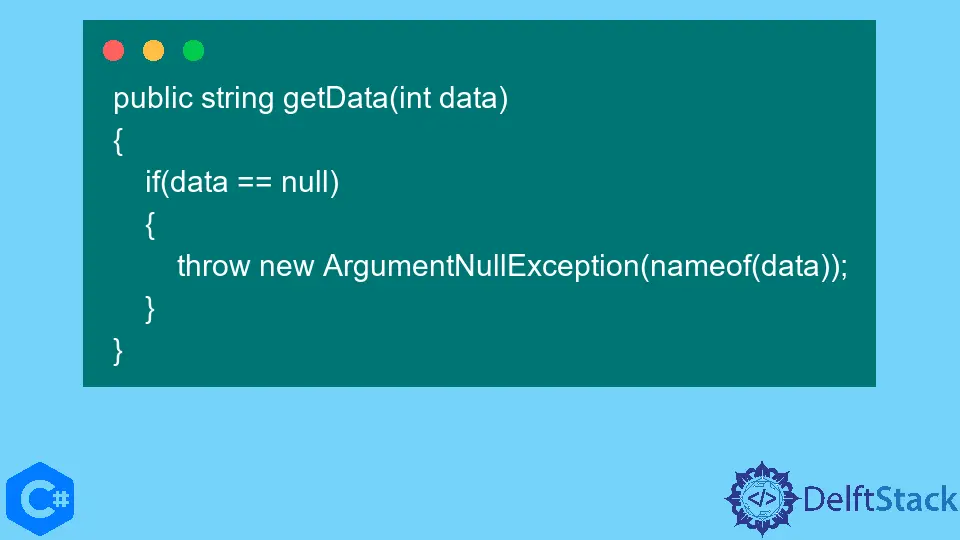
This tutorial will discuss the uses of the nameof expression in C#.
the nameof
Expression in C#
The nameof
expression gets the name of a variable, a data type, a field, or a member in C#. The nameof
expression takes an artifact as an input and returns the name of that particular artifact in string format. It is illustrated in the code example below.
using System;
namespace nameof_operator {
class Program {
static void Main(string[] args) {
int number = 15;
Console.WriteLine(nameof(number));
Console.WriteLine(nameof(System.Int32));
Console.WriteLine(nameof(nameof_operator));
}
}
}
Output:
number
Int32
nameof_operator
We printed the name of a variable, a data type, and a namespace with the nameof
expression in C#. The nameof
expression gets evaluated during the compile-time and does not affect the processing speed during run-time. The nameof
expression’s main use is in the argument checking for ArgumentException
and anything related to it. For example, if we have a variable named data
and we are displaying a message saying that the data
variable is not initialized if it is null. It will work just fine in a conventional program, but if we change the name of the data
variable to the input
variable, we also have to change the output message. This scenario can be elegantly handled with just a single nameof
expression, as shown in the coding example below.
public string getData(int data) {
if (data == null) {
throw new ArgumentNullException(nameof(data));
}
}
Now we don’t have to worry about changing a lot of code to rename our data
variable to something else.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn