C# List Index
- Understanding C# Lists
- Accessing List Items by Index
- Handling Index Out of Range Exceptions
- Using the Find Method to Retrieve Items
- Conclusion
- FAQ
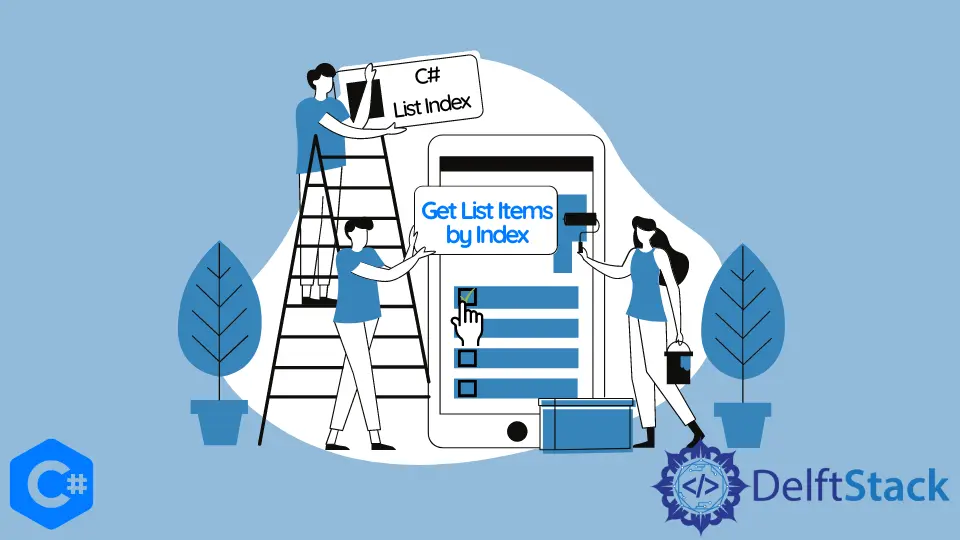
When working with lists in C#, accessing elements by their index is a fundamental skill that can significantly enhance your programming efficiency. Whether you’re developing a small application or a large-scale software project, understanding how to manipulate lists is crucial.
In this tutorial, we will explore how to get a list item by index in C#. We will cover several methods, including basic index access, error handling, and using built-in methods to retrieve items. By the end of this article, you will be equipped with the knowledge to confidently work with lists in C#. Let’s dive in!
Understanding C# Lists
Before we get into accessing list items by index, it’s essential to understand what a list is in C#. A list is a collection that can hold a variable number of items. Unlike arrays, lists can dynamically resize themselves, making them incredibly flexible for various programming scenarios. Lists in C# are part of the System.Collections.Generic namespace, which means they can store any data type, including custom objects.
To create a list, you typically use the List<T>
class, where T
represents the type of elements in the list. Here’s a quick example:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<string> fruits = new List<string> { "Apple", "Banana", "Cherry" };
}
}
In this example, we create a list of strings called fruits
. Now that we have a basic understanding of lists in C#, let’s explore how to access items by their index.
Accessing List Items by Index
The most straightforward way to access an item in a list is by using its index. In C#, list indices are zero-based, meaning that the first item is at index 0, the second item is at index 1, and so forth. Here’s how you can retrieve an item from a list by its index:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<string> fruits = new List<string> { "Apple", "Banana", "Cherry" };
string firstFruit = fruits[0];
Console.WriteLine(firstFruit);
}
}
Output:
Apple
In this code snippet, we create a list of fruits and then access the first item using fruits[0]
. The output confirms that we successfully retrieved the item “Apple”. This method is efficient and direct, making it ideal for scenarios where you know the index of the item you want to access.
Handling Index Out of Range Exceptions
While accessing list items by index is simple, it’s crucial to handle potential errors, particularly when the index might be out of range. If you try to access an index that doesn’t exist, C# will throw an ArgumentOutOfRangeException
. To prevent your application from crashing, you can implement error handling. Here’s how to do that:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<string> fruits = new List<string> { "Apple", "Banana", "Cherry" };
int index = 3;
try
{
string fruit = fruits[index];
Console.WriteLine(fruit);
}
catch (ArgumentOutOfRangeException)
{
Console.WriteLine("Index is out of range.");
}
}
}
Output:
Index is out of range.
In this example, we attempt to access an index (3) that doesn’t exist in the list. The try-catch
block allows us to handle the exception gracefully. Instead of crashing, the program outputs a friendly message indicating that the index is out of range. This method enhances the robustness of your application by ensuring that it can handle unexpected input.
Using the Find Method to Retrieve Items
Another effective way to access items in a list is by using the Find
method. This method allows you to search for an item based on a specific condition. It’s particularly useful when you don’t know the index but have criteria to find the item. Here’s an example:
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
List<string> fruits = new List<string> { "Apple", "Banana", "Cherry" };
string fruit = fruits.Find(f => f.StartsWith("B"));
Console.WriteLine(fruit);
}
}
Output:
Banana
In this code, we use the Find
method to search for a fruit that starts with the letter “B”. The lambda expression f => f.StartsWith("B")
defines the condition for the search. The method returns the first item that matches the condition, which in this case is “Banana”. This approach is beneficial when dealing with larger lists where you may not know the exact index of the item you want to retrieve.
Conclusion
In summary, accessing list items by index in C# is a fundamental skill that every developer should master. Whether you choose to access items directly, handle exceptions, or use methods like Find
, understanding how to manipulate lists will significantly improve your programming capabilities. Remember to always consider the potential for index errors, and don’t hesitate to use built-in methods to simplify your code. With these techniques at your disposal, you’re well on your way to becoming proficient in C# list management.
FAQ
-
What is a C# list?
A C# list is a collection that can store a variable number of items of the same type, allowing for dynamic resizing. -
How do I create a list in C#?
You can create a list using theList<T>
class, whereT
is the type of elements you want to store. -
What happens if I access an index that is out of range?
Accessing an out-of-range index will throw anArgumentOutOfRangeException
, which can be handled using a try-catch block. -
Can I use the Find method with custom objects?
Yes, theFind
method can be used with custom objects by providing a condition that matches the properties of the object. -
Are lists in C# thread-safe?
No, lists in C# are not thread-safe by default. You may need to implement your own synchronization if you’re accessing a list from multiple threads.
List Index teaches you how to access list items by index effectively. Learn about direct access, error handling, and using methods like Find to retrieve items. Enhance your programming skills with practical examples and best practices for working with lists in C#. Perfect for beginners and experienced developers alike.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn