LINQ Group by in C#
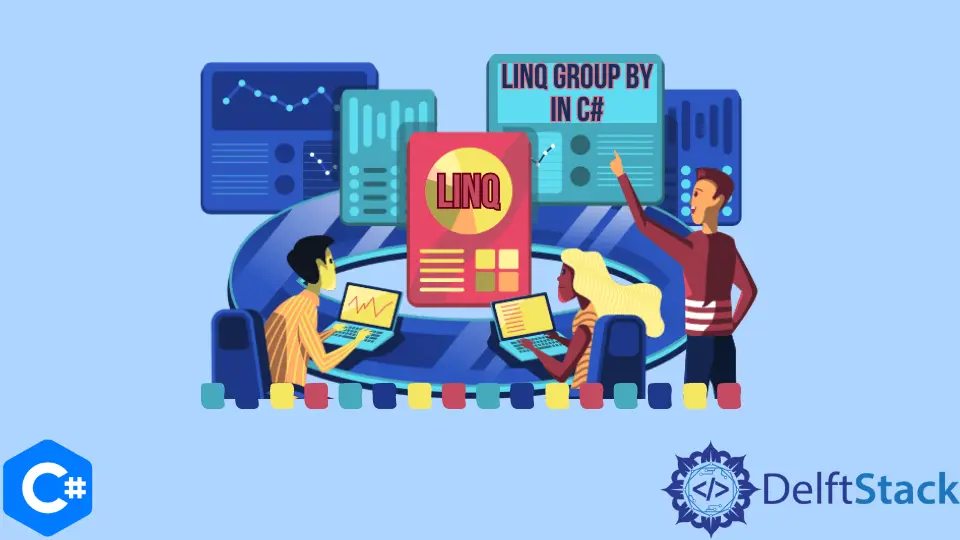
This tutorial will discuss the method to group a list of objects by a value in C#.
LINQ Group by in C#
The LINQ integrates SQL-like query functionality with data structures in C#.
Suppose we have a list of objects of the following class.
class Car {
public string Brand { get; set; }
public int Model { get; set; }
}
Brand
is the name of the car’s brand, and Model
is the model number of the car. The Brand
property of multiple objects can be the same, but the Model
number needs to be different for each object. If we want to group the list of objects by the brand name, we can use the GroupBy
method in LINQ. The following code example shows us how we can group objects of a specific class by some value with the GroupBy
method in LINQ.
using System;
using System.Collections.Generic;
using System.Linq;
namespace linq_goup_by {
public class Car {
public string Brand { get; set; }
public int Model { get; set; }
public Car(string b, int m) {
Brand = b;
Model = m;
}
}
class Program {
static void Main(string[] args) {
List<Car> cars = new List<Car>();
cars.Add(new Car("Brand 1", 11));
cars.Add(new Car("Brand 1", 22));
cars.Add(new Car("Brand 2", 12));
cars.Add(new Car("Brand 2", 21));
var results = from c in cars group c by c.Brand;
foreach (var r in results) {
Console.WriteLine(r.Key);
foreach (Car c in r) {
Console.WriteLine(c.Model);
}
}
}
}
}
Output:
Brand 1
11
22
Brand 2
12
21
In the above code, we first declared and initialized a list of objects cars
and then grouped the values by the Brand
property and save it in the results
variable.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn