How to Convert an Image to Base64 String in C#
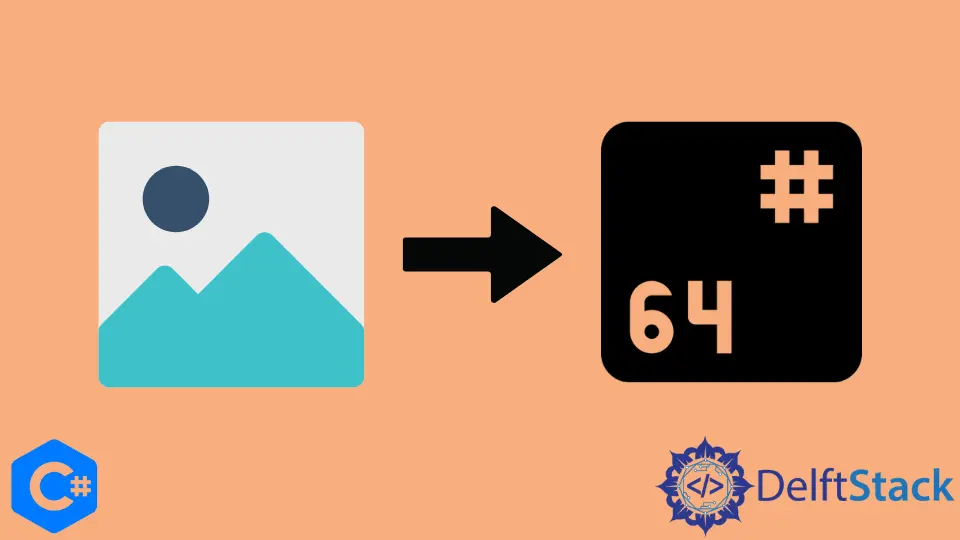
This article will illustrate how to convert an image into a base64 string using C#.
Encoding in base64 is a technique that is often used whenever there is a need to transform binary data into a textual representation. Numerous applications, such as sending an email using MIME and storing complicated data in XML, frequently use the base64 encoding standard.
Convert an Image to Base64 String in C#
Firstly, import the libraries to access the classes and functions in the program.
using System;
Create a class ImagetoBase64
and create a Main()
method inside this class.
class ImagetoBase64 {
public static void Main() {}
}
Inside the Main()
function, create a variable imageArray
of type byte array denoted as byte[]
that converts the selected image into a byte array using the ReadAllBytes()
function.
byte[] imageArray = System.IO.File.ReadAllBytes("E://image.jpg");
Create a new variable called base64Image
of the string type. This variable will convert the bytes of the picture into base64 using the Convert
class’s function ToBase64String().
This function takes a portion of an array of 8-bit unsigned integers and generates a string representation of it that is encoded using base-64 digits.
The parameters allow the user to define the subset as an offset inside the input array and the number of array items to be converted. It also enables determining whether or not the line breaks should be included in the return result.
string base64Image = Convert.ToBase64String(imageArray);
In the end, all that is left to do is display the picture once it has been transformed into base64 text.
Console.WriteLine("Base 64 string\n\n" + base64Image);
Complete Source Code:
using System;
class ImagetoBase64 {
public static void Main() {
byte[] imageArray = System.IO.File.ReadAllBytes("E://image.jpg");
string base64Image = Convert.ToBase64String(imageArray);
Console.WriteLine("Base 64 string\n\n" + base64Image);
}
}
Since the base64 is rather lengthy, let us insert some ...
to clarify that it continues like this.
Output:
/9j/4AAQSkZJRgABAQEASABIAAD/4gxYSUNDX1BST0ZJTEUAAQEAAAxITGlubwIQAABtbnRyUkdCIFhZWiAHzgACAAkABgAxAABhY3NwTVNGVAAAAABJRUMgc1JHQgAAAAAAAAAAAAAAAAAA9tYAAQAAAADTLUhQICAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABFjcHJ0AAABUAAAADNkZXNjAAABhAAAAGx3dHB0AAAB8AAAABRia3B0AAACBAAAABRyWFlaAAACGAAAABRnWFlaAAACLAAAABRiWFlaAAACQAAAABRkbW5kAAACVAAAAHBkbWRkAAACxAAAAIh2dWVkAAADTAAAAIZ2aWV3AAAD1AAAACRsdW1pAAAD......
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn