C# If-Else Short Hand
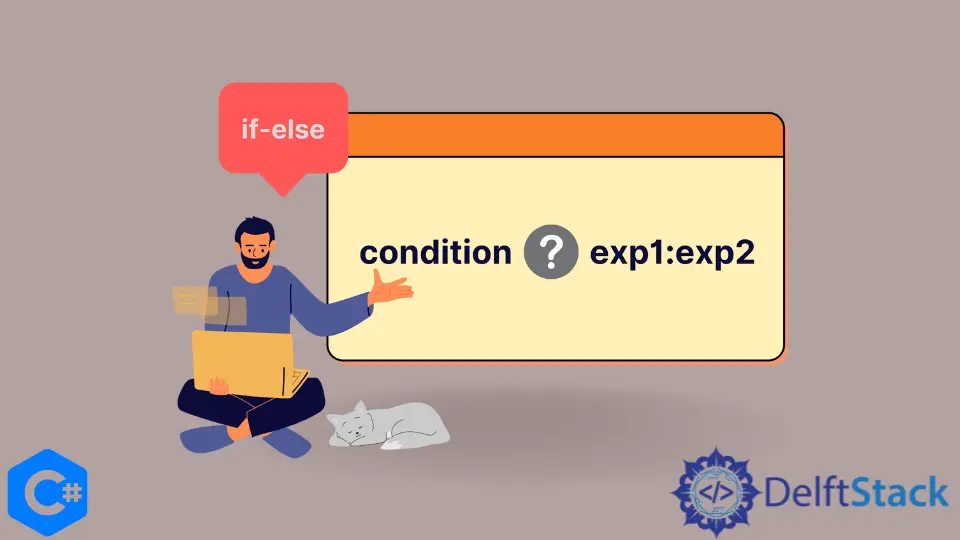
The If-Else
statements are used to execute conditional code blocks. We specify a condition in the if
block. On meeting that condition, the if
code block is executed.
Otherwise, the else
code block is executed. This tutorial will look at the ternary operator ?:
, the if-else
shorthand in C#.
Using the Ternary Operator in C#
The ternary operator
gets its name because it takes three arguments as input: the condition, the if
code block, and the else
code block.
All three are wrapped in a one-line shorthand, making code concise and clean. It helps achieve the same functionality as if-else
in minimalist code.
using System;
class Program {
public static void Main() {
int exp1 = 5;
double exp2 = 3.0;
bool condition = 5 > 2;
var ans = condition ? exp1 : exp2;
Console.WriteLine(ans);
}
}
Output:
5
In the above example, the ternary operator will first evaluate the given condition. If the specified condition is true
, we move to exp1
, separated by a ?
condition. Otherwise, we move to exp2
, separated from exp1 by a :
.
The power of ternary operators doesn’t stop here as we know if-else
statements can be nested. Ternary operators can also achieve the same with much lesser code.
Using the Nested Ternary Operators in C#
using System;
class Program {
public static void Main() {
int alcoholLevel = 90;
string message = alcoholLevel >= 100
? "You are too drunk to drive"
: (alcoholLevel >= 80 ? "Come on live a little" : "Sober :)");
Console.WriteLine(message);
}
}
Output:
Come on live a little
In the above example, we output multiple messages based on the alcohol level of a person using nested ternary operators, all packed in a single line of code.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn