The #if DEBUG in C#
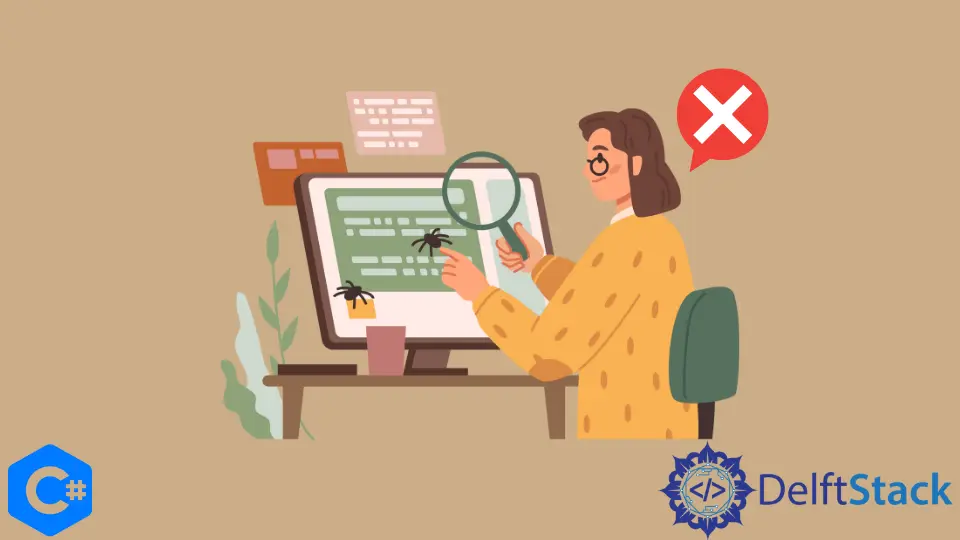
This article will discuss the #if DEBUG
statement in the C# programming language.
the #if DEBUG
in C#
The #if DEBUG
instruction operates in the same manner as an if
condition in the program, which means that it will determine whether or not the program is now operating in the debug
mode.
If we run the program in the debug
mode inside Visual Studio, the condition of the program will immediately become true
, and it will execute the code block specified for the true
scenario.
A conditional directive that starts with a #if
directive must conclude with an explicit #endif
directive to function properly.
Implementation of #if DEBUG
in C#
Let us take an example to understand the working of #if DEBUG
in the C# programming language.
Importing the library is the first step, followed by developing an ifDebugBySaad
class and a main()
method inside that class to launch the program’s operations.
using System;
class ifDebugBySaad {
static void Main(string[] args) {}
}
Initialize a variable name
of string
datatype and assign it a value.
String name = "Saad Aslam";
After that, we will construct a condition that will determine whether or not the application is operating in debug
mode and if the answer is true
, the if
block will be carried out.
#if DEBUG
Console.WriteLine("My name is: " + name);
We use the #else
statement if the program is not running in debug
mode to print a message to the user. The conclusion of an if
the #endif
directive indicates a block.
#else
Console.WriteLine("The program is not in debug mode");
#endif
If we want the condition #if DEBUG
to always evaluate to true
, we need to put the #define DEBUG
directive at the very beginning of our code before we declare any libraries.
Full Code:
using System;
class ifDebug {
static void Main(string[] args) {
String name = "Saad Aslam";
#if DEBUG
Console.WriteLine("My name is: " + name);
#else
Console.WriteLine("The program is not in debug mode");
#endif
}
}
Output:
My name is: Saad Aslam
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn