HTML to PDF in C#
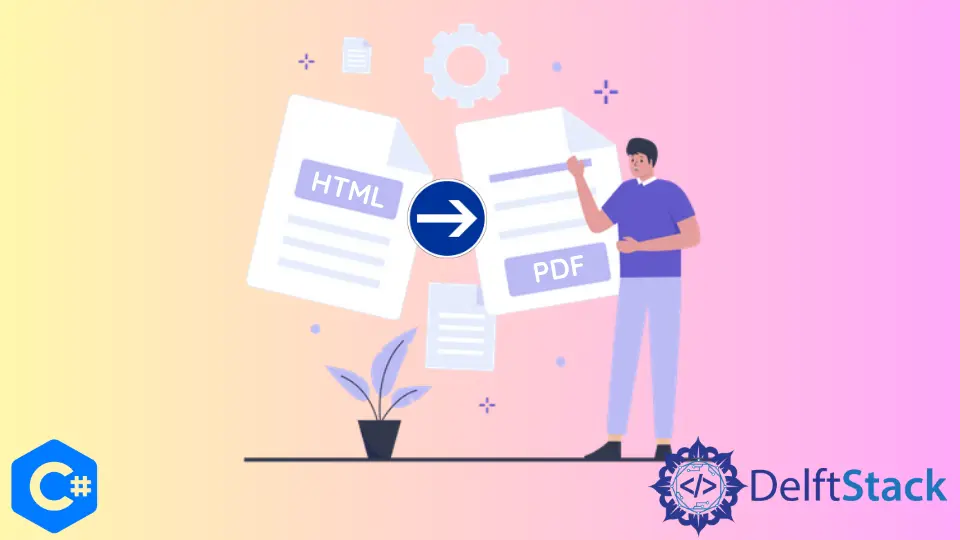
This tutorial will introduce the method to convert an HTML string to PDF in C#.
HTML to PDF With the HtmlRenderer.PdfSharp
Package in C#
The HtmlRenderer.PdfSharp
package is used to generate a pdf file from HTML snippets in C#. This package makes it very easy to convert a string containing an HTML snippet into a PDF document that contains the web view of that HTML snippet. The HtmlRenderer.PdfSharp
package is an external package and does not come pre-installed with the .NET
library. We have to install this package separately using the NuGet package manager. The command to install the HtmlRenderer.PdfSharp
package is given below.
dotnet add package HtmlRenderer.PdfSharp-- version 1.5.0.6
The following code example shows us how to convert a string containing an HTML snippet to a PDF file with the HtmlRenderer.PdfSharp
package in C#.
using PdfSharp;
using PdfSharp.Pdf;
using System;
using System.IO;
using TheArtOfDev.HtmlRenderer.PdfSharp;
namespace html_to_pdf {
class Program {
static void Main(string[] args) {
string htmlString =
"<h1>Document</h1> <p>This is an HTML document which is converted to a pdf file.</p>";
PdfDocument pdfDocument = PdfGenerator.GeneratePdf(htmlString, PageSize.A4);
pdfDocument.Save("C:/File/HTML to PDF Document.pdf");
}
}
}
C:\File\HTML to PDF Document.pdf
File:
In the above code, we converted the HTML snippet inside the string variable htmlString
to a PDF file with the HtmlRenderer.PdfSharp
package in C#. We first initialized the string htmlString
with an HTML snippet. We then created an instance of the PdfDocument
class with the PdfGenerator.GeneratePdf()
function in C#. In the end, we saved the document to a specified path with the PdfDocument.Save()
function. The file content can be seen in the screen snippet displayed above.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn