How to Get File Name From the Path in C#
-
Understanding the
GetFileName()
Method - Handling Different Path Formats
- Extracting File Names Without Extensions
- Best Practices for File Path Handling
- Conclusion
- FAQ
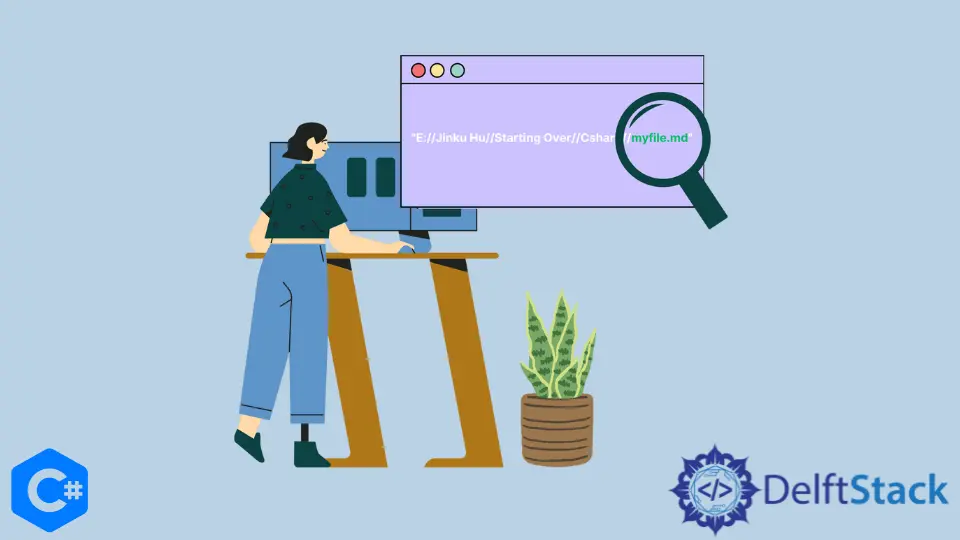
Extracting the file name from a given path in C# is a common task that developers encounter frequently. Whether you are working on a file management system, a data processing application, or simply need to manipulate file paths, knowing how to efficiently retrieve the file name is essential.
In this article, we will explore the GetFileName()
method provided by the System.IO
namespace, which makes this task straightforward and efficient. We’ll provide clear examples, explain how the method works, and discuss best practices to ensure that you can implement this functionality seamlessly in your projects. Let’s dive into the details!
Understanding the GetFileName()
Method
The GetFileName()
method is part of the Path
class in the System.IO
namespace. This method takes a string representing a file path and returns the file name and extension. It simplifies the process of extracting the file name from a full path, eliminating the need for complex string manipulation.
Here’s a simple example of how to use the GetFileName()
method:
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = @"C:\Users\Example\Documents\file.txt";
string fileName = Path.GetFileName(filePath);
Console.WriteLine(fileName);
}
}
Output:
file.txt
In this code, we first include the necessary namespaces. We then define a file path and use the GetFileName()
method to extract the file name. Finally, we print the result to the console.
The beauty of this method lies in its simplicity. You don’t have to worry about parsing the string or handling different directory separators. The method automatically takes care of that, making it a reliable choice for developers.
Handling Different Path Formats
When working with file paths, you may encounter various formats, including absolute and relative paths. The GetFileName()
method handles both types seamlessly. Here’s an example that demonstrates its versatility:
using System;
using System.IO;
class Program
{
static void Main()
{
string absolutePath = @"C:\Users\Example\Documents\file.txt";
string relativePath = @"..\Documents\file.txt";
Console.WriteLine(Path.GetFileName(absolutePath));
Console.WriteLine(Path.GetFileName(relativePath));
}
}
Output:
file.txt
file.txt
In this example, we define both an absolute and a relative path. The GetFileName()
method successfully extracts the file name from both paths. This flexibility is particularly useful when your application needs to handle user input or file paths from different sources.
By utilizing the GetFileName()
method, you can ensure that your application remains robust and adaptable to various scenarios. It abstracts away the complexity of different path formats, allowing you to focus on other aspects of your project.
Extracting File Names Without Extensions
Sometimes, you may need to extract just the file name without its extension. While the GetFileName()
method does not provide this functionality directly, you can achieve it by combining it with the GetFileNameWithoutExtension()
method. Here’s how:
using System;
using System.IO;
class Program
{
static void Main()
{
string filePath = @"C:\Users\Example\Documents\file.txt";
string fileNameWithoutExtension = Path.GetFileNameWithoutExtension(filePath);
Console.WriteLine(fileNameWithoutExtension);
}
}
Output:
file
In this code snippet, we utilize the GetFileNameWithoutExtension()
method to retrieve the file name without its extension. This can be particularly useful in scenarios where you want to display file names in a user-friendly manner or when you need to perform operations based on the file name alone.
Combining these methods allows for greater flexibility in file handling. You can easily switch between getting the full file name and just the base name, depending on your needs.
Best Practices for File Path Handling
When working with file paths in C#, it’s essential to follow best practices to ensure your code remains clean and efficient. Here are a few tips to keep in mind:
-
Use Path Class: Always utilize the
Path
class for file path manipulations. It provides a set of methods that handle platform-specific details, ensuring your code works across different operating systems. -
Validate Input: When dealing with user input for file paths, always validate the input to avoid exceptions. Check if the path is valid and if the file exists before attempting to extract the file name.
-
Exception Handling: Implement proper exception handling around file operations. This will help you manage errors gracefully and provide a better user experience.
-
Keep It Simple: Avoid unnecessary complexity in your code. Use built-in methods like
GetFileName()
andGetFileNameWithoutExtension()
to keep your code clean and readable.
By adhering to these best practices, you can ensure that your file handling code is not only functional but also robust and maintainable.
Conclusion
In conclusion, extracting the file name from a given path in C# is a straightforward task with the help of the GetFileName()
method from the System.IO
namespace. This method simplifies the process and allows developers to focus on other critical aspects of their applications. By understanding how to handle different path formats and utilizing best practices, you can effectively manage file paths in your projects. Remember to keep your code clean and efficient, and you’ll be well on your way to mastering file handling in C#.
FAQ
-
How do I get the file name from a URL in C#?
You can use thePath.GetFileName()
method on the URL string after converting it to a valid file path. -
Can I extract the file name from a path with multiple directories?
Yes, theGetFileName()
method will return the last segment of the path, which is the file name, regardless of how many directories are present. -
What if the file name contains special characters?
TheGetFileName()
method handles special characters appropriately, returning the file name as is.
-
Is there a way to get the file extension separately?
Yes, you can use thePath.GetExtension()
method to retrieve just the file extension from a path. -
Can I use these methods in a .NET Core application?
Absolutely! ThePath
class and its methods are available in .NET Core, making them suitable for cross-platform applications.
#. Learn to use the GetFileName() method effectively, handle different path formats, and extract file names without extensions. Perfect for developers looking to streamline file management in their C# applications.