How to Upload a File to FTP in C#
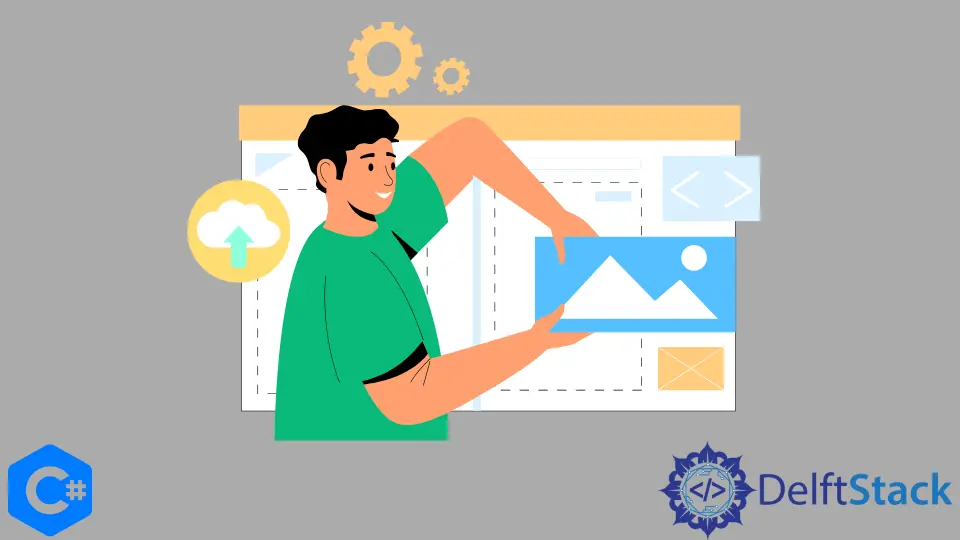
This guide teaches how to upload a file to FTP in C#. This is a very simple procedure.
We assume that you are already familiar with the concepts and basics of FTP. Let’s dive into this guide.
Upload a File to FTP in C#
First of all, define the file path and store it inside a string and pass that string in FileInfo
. The second step is to define your server URL; you’ll have to give a folder a name where you want to upload the file.
The third step is to combine these paths into one URL.
After that, you’ll have to enter your username and password and pass the one URL created earlier to FtpWebRequest
and store it inside the FtpWebRequest
object (in this case, it is req
).
You’ll have to choose the method as Uploading
. After that, set up the credentials.
The last step is to copy the same content to the request stream.
// Path of file to be uploaded
string file_path = "Your File path ";
string PureFileName = new FileInfo(file_path).Name;
// Your Server url
string ftp_server_url = "Enter your server url";
// Name of folder in which you want to upload
// leave it empty if you want to upload to root directory/folder
string folder = "";
// combining the path
String uploadUrl = String.Format("{0}{1}/{2}", ftp_server_url, folder, PureFileName);
// username
string username = "Your Username";
// password
string password = "Your Password";
// creating ftp request
FtpWebRequest req = (FtpWebRequest)WebRequest.Create(uploadUrl);
req.Proxy = null;
req.Method = WebRequestMethods.Ftp.UploadFile;
// setting ftp credentials
req.Credentials = new NetworkCredential(username, password);
req.UseBinary = true;
req.UsePassive = true;
// copy content to request stream
byte[] data = File.ReadAllBytes(file_path);
req.ContentLength = data.Length;
Stream stream = req.GetRequestStream();
stream.Write(data, 0, data.Length);
stream.Close();
// status from ftp server
FtpWebResponse res = (FtpWebResponse)req.GetResponse();
return res.StatusDescription;
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn