How to Find Substring in a String in C#
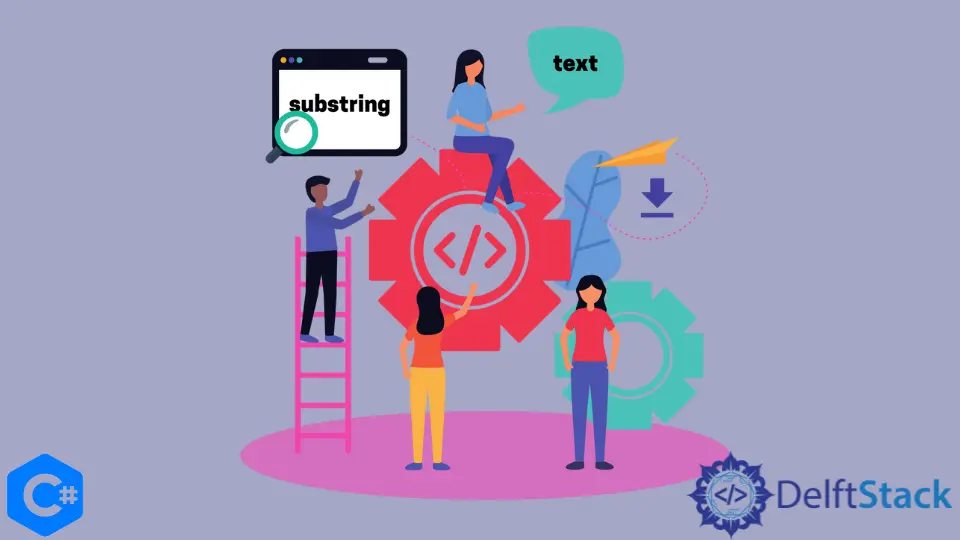
This tutorial will discuss the method for extracting the text between two words within a string in C#.
Extract Text From a String in C#
If we have a string variable that has a value like Hi, I am a string variable
and we want to find the text between the words Hi
and string
, we can use the String.IndexOf()
method along with the String.SubString()
method to achieve this goal.
The String.IndexOf(x)
method gets the index of a particular string x
inside the string. The String.SubString(x, y)
method extracts a sub-string on the basis of the start index x
and end index y
. We can get the indices of the starting and the ending strings inside the main string with the String.IndexOf()
function. We can then extract the text between both strings by passing both words’ indices to the String.SubString()
function. The following code example shows us how we can extract text from a string with the String.IndexOf()
and String.SubString()
methods in C#.
using System;
namespace text_from_string {
class Program {
public static string stringBetween(string Source, string Start, string End) {
string result = "";
if (Source.Contains(Start) && Source.Contains(End)) {
int StartIndex = Source.IndexOf(Start, 0) + Start.Length;
int EndIndex = Source.IndexOf(End, StartIndex);
result = Source.Substring(StartIndex, EndIndex - StartIndex);
return result;
}
return result;
}
static void Main(string[] args) {
string s = "Hi, I am a string variable.";
string word1 = "Hi";
string word2 = "string";
string text = stringBetween(s, word1, word2);
Console.WriteLine(text);
}
}
}
Output:
, I am a
In the above code, we define the function stringBetween()
that takes the main string and both words as parameters and returns the texts between the words inside the main string. We initialized the starting index StartIndex
of the text with the Source.IndexOf(Start, 0) + Start.Length
statement. This statement gets the index of the Start
string inside the Source
string and then increments it with the length of Start
string so that the Start
does not come in the text result. The same procedure is carried out for the ending index EndIndex
of the text. We then returned the text by giving the StartIndex
as starting index and EndIndex-StartIndex
as the length of the new string to the String.SubString()
function.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn