Exponent in C#
Minahil Noor
Feb 16, 2024
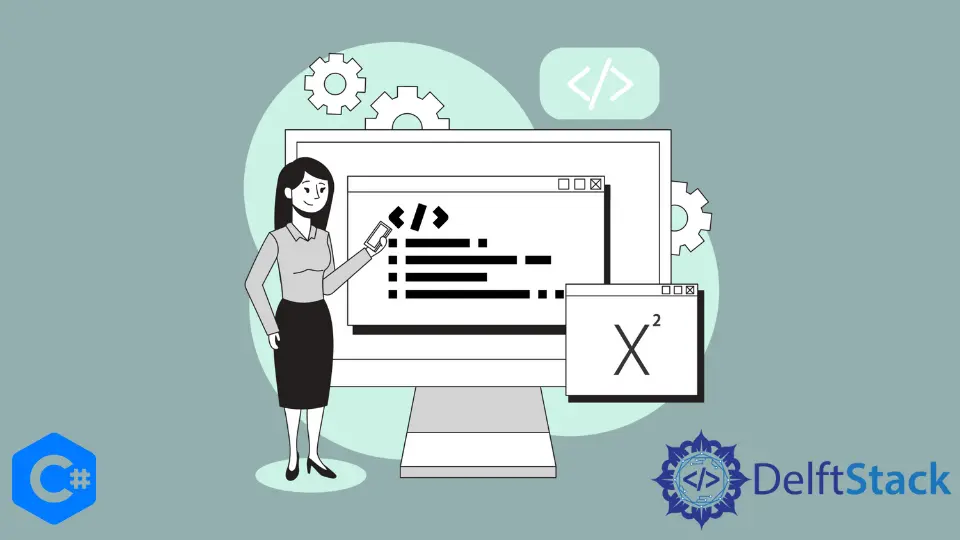
This article will introduce the exponent operator in C#.
Use the Pow()
Method as Exponent Operator in C#
In C#, there is no specific operator for the exponent. There is a method Math.Pow()
that we can use to raise a number to some power. The correct syntax to use this method is as follows.
Math.Pow(x, y);
This method returns the result after calculating x raised to the power y. The detail of its parameters is as follows.
Parameters | Description | |
---|---|---|
x |
base |
|
y |
exponent |
The program below shows how we can use the Pow()
method to raise a number to specific power.
using System;
public class Program {
public static void Main() {
double Result, Number1, Number2;
Number1 = 3;
Number2 = 4;
Result = Math.Pow(Number1, Number2);
Console.WriteLine(Result);
}
}
Output:
81
The function has returned the result after calculating 3 raised to the power 4(3x3x3x3).