How to Exit a Foreach Loop in C#
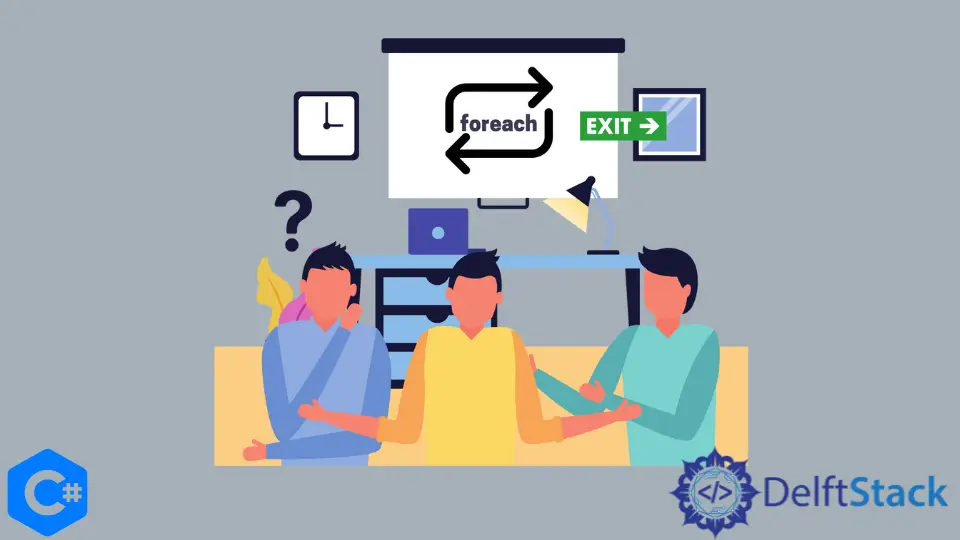
This guide will teach us how to exit a foreach
loop in C#. This is yet another simple method, and there’s no complexity involved.
Let’s dive into this guide and see the implementation of this code.
Exit a foreach
Loop in C#
There are two ways that you can use to exit a foreach
loop or any other loop for that matter. Exiting from a foreach
loop is the same as exiting from any other loop.
Both of these ways are very common, and they are the ones that are mostly used in many other languages as well. For instance, C, C++, Java, etc.
Either we can use the break
method or the return
method. Both of these ways can be used to exit a foreach
loop.
Take a look at the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace For_Each_Loop {
class Program {
static void Main(string[] args) {
int[] numbers_arr = { 4, 5, 6, 1, 2, 3, -2, -1, 0 }; // Example Array
foreach (int i in numbers_arr) {
System.Console.Write("{0} ", i);
Console.ReadKey(); // To Stay On Screen
if (i == 3) {
break; // IF You want To break and end the function call
}
}
foreach (int i in numbers_arr) {
System.Console.Write("{0} ", i);
Console.ReadKey(); // To Stay On Screen
if (i == 2) {
return; // IF You want To break and end the function call
}
}
}
}
}
We use both break
and return
in the above code. All you need to do is write break;
or return;
inside the foreach
loop according to your needs.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn