C# Equals() vs ==
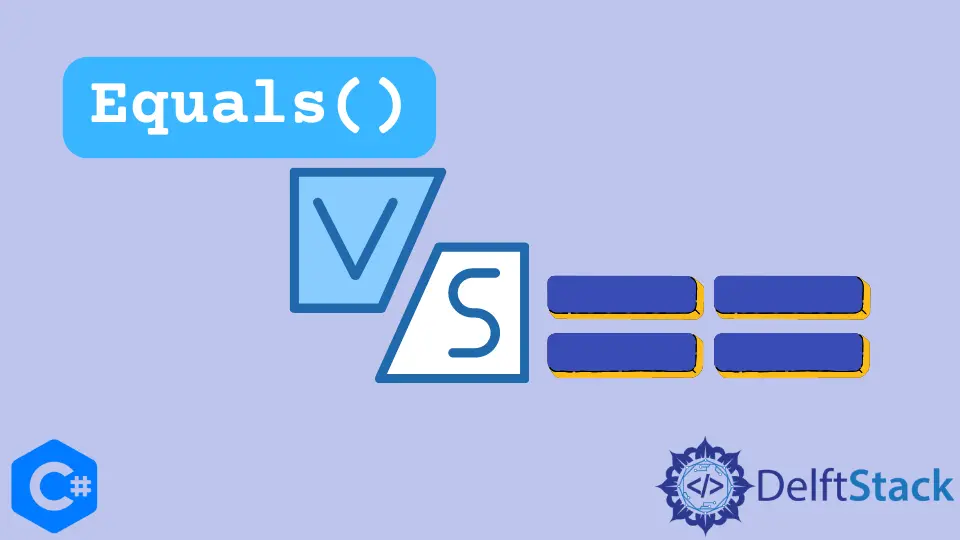
This tutorial will highlight the differences and similarities of the ==
operator and the Equals()
function in C#.
Difference Between the ==
Operator and the Equals()
Function in C#
The ==
operator is a comparison operator in C#. The ==
operator compares the reference identities of both operands. On the other hand, the Equals()
function compares the contents of two string variables in C#. Typically, both are used for comparing two values. But, they can have different results in different scenarios. The following code example will show a scenario where both the ==
operator and the Equals()
function are true.
using System;
namespace comparison {
class Program {
static void Main(string[] args) {
object var1 = "MMA";
object var2 = var1;
if (var1 == var2) {
Console.WriteLine("== is true");
}
if (var1.Equals(var2)) {
Console.WriteLine("Equals is true");
}
}
}
}
Output:
== is true
Equals is true
In the above code, we initialized an object variable var2
with var2 = var1
. It means that both var1
and var2
refer to the same identity in the memory. So, the ==
operator will result in a true
outcome. Since they both have the same contents inside them, the Equals()
function will also give true
.
Now, we will demonstrate another scenario in which the ==
operator gives false
but the Equals()
function returns a true
value. This scenario is discussed in the code example below.
using System;
namespace comparison {
class Program {
static void Main(string[] args) {
object var1 = "MMA";
char[] varc = { 'M', 'M', 'A' };
object var2 = new string(varc);
if (var1 == var2) {
Console.WriteLine("== is true");
}
if (var1.Equals(var2)) {
Console.WriteLine("Equals is true");
}
}
}
}
Output:
Equals is true
In the above code, we created the character array varc
and converted it to the object variable var2
by passing varc
in the constructor of a string variable. It means that var1
and var2
are both pointing at different identities in the memory. The ==
operator returns false
because it compares the reference identities of var1
and var2
. But, the Equals()
function returns true because it is comparing the contents of var1
and var2
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn