Double Question Mark in C#
Minahil Noor
Feb 16, 2024
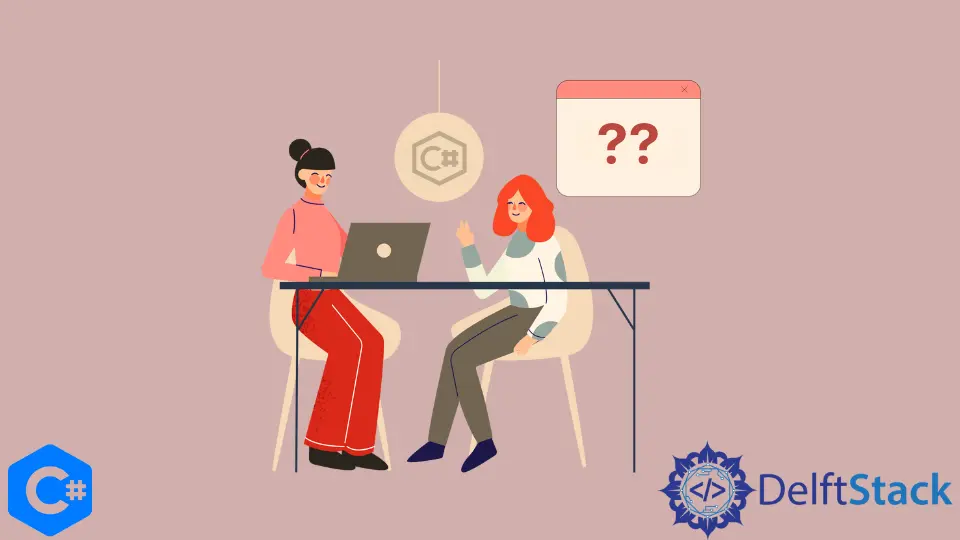
This article will introduce double question mark meaning in C#.
Use the ??
Operator as a Null Coalescing Operator in C#
We use the ??
operator as a null coalescing operator in C#. It returns the value of its left-hand operand if it isn’t null. If it is null, then it evaluates the right-hand operand and returns its result. The ??
operator does not evaluate its right-hand operand if the left-hand operand evaluates to non-null. The correct syntax to use this symbol is as follows.
A ?? B
In the above example, B
is returned if A
is null.
The program below shows how we can use the null coalescing operator.
using System;
public class Program {
public static void Main() {
int? a = null;
int b = a ?? 10;
Console.WriteLine(b);
}
}
Output:
10
In the above code, we can see that the value of b
is 10 as a
is null.