How to Disable and Enable Buttons in C#
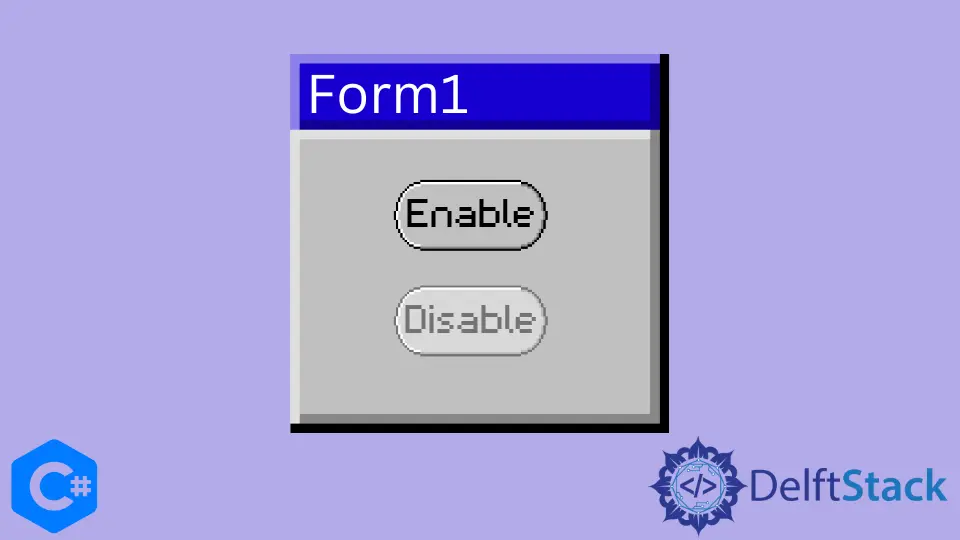
This guide will show how to disable and enable the buttons in C#. How to create and use them?
Create a Button in C#
Now there are two ways in which we can create a button. Both of them are explained below.
Use Toolbox to Create Button in C#
We can create a button using the toolbox in windows forms and drag it anywhere we please on that form. Then we can go to the properties of the button, where we can toggle with our button.
We can change the color, size, and shape of the button in the form.
Use buttonBase
Class to Create Button in C#
This method will use the buttonBase
class to create and assign our button operations. And we create it at runtime.
We must remember that we can create a button by using the toolbox, but we won’t be able to make any further changes.
The only change it allows is enabling the button as either true
or false
. So, to make these further changes, such as writing a condition.
We write some code to tell our button how to perform.
Combine These Two Methods to Create Button in C#
The easiest way to create a button is using a toolbox and then making the changes to that button through code. The button would be created in the form.
You can make the desired changes to it using its properties like shape, size, font, color, and many more. You’ll still be able to add further conditions by code.
In this example, this technique will be used.
Enable and Disable the Button in C#
The enabling and disabling of the button at runtime is by adding its properties one by one and adding the button to the windows form by adding an instance of the Button
class. By default, the button is true
.
Before you go ahead and take a look at the code down below, you must know that the library to include to make this all work is System.Windows.Forms
Here is an example.
using System.Windows.Forms;
public partial class FormOne : Form {
public FormOne() {
InitializeComponent();
}
public void FormOne_Load(object sender, EventArgs e) {
Mybutton.Enabled = true; // to enable the button
Label first = new Label(); // adding a label to the button
first.Text = "this is the first button, it is enabled";
// Mybutton.Enabled=false; // to disable the button
// Label first=new Label(); //adding a label to the button
// first.Text="this is the first button, it is now disabled";
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn