How to Destroy Object in C#
- Assigning Null to an Object
- Using the Dispose Method
- Finalizers and the Garbage Collector
- Conclusion
- FAQ
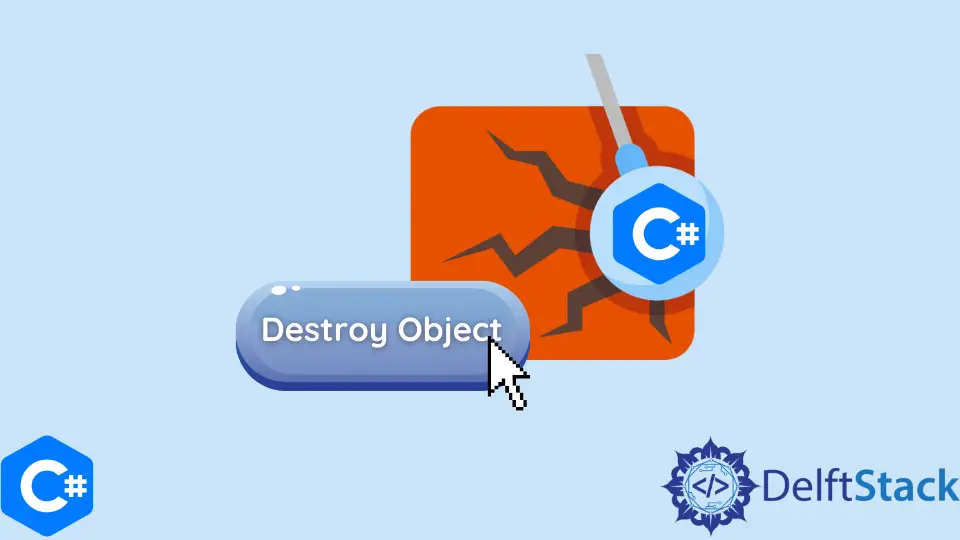
When working with C#, managing memory efficiently is crucial for optimal performance. One fundamental aspect of this is understanding how to destroy or deallocate objects. In C#, objects are typically destroyed by assigning a null value to them. This simple yet effective method signals to the garbage collector that the memory can be reclaimed, freeing up resources for other operations.
In this article, we will explore various methods to destroy objects in C#, providing clear examples and explanations to help you grasp the concept fully. Whether you are a beginner or an experienced developer, understanding object destruction is essential for writing efficient C# code.
Assigning Null to an Object
One of the most straightforward ways to destroy an object in C# is by assigning a null value to it. When you assign null to an object, you effectively remove its reference, making it eligible for garbage collection. This method is particularly useful when you no longer need an object and want to free up memory.
Here’s a simple code example demonstrating this method:
class Program
{
static void Main()
{
MyClass myObject = new MyClass();
myObject = null;
}
}
class MyClass
{
public int Value { get; set; }
}
In this example, we first create an instance of MyClass
and assign it to myObject
. Later, we set myObject
to null. By doing this, we remove the reference to the MyClass
instance, allowing the garbage collector to reclaim the memory. It’s important to note that setting an object to null does not immediately free the memory; it merely marks it for garbage collection. The garbage collector will run at its discretion, cleaning up unreferenced objects when necessary.
Using the Dispose Method
Another effective way to manage object destruction in C# is by implementing the IDisposable
interface. This is particularly relevant for objects that hold onto unmanaged resources, such as file handles or database connections. By using the Dispose
method, you can explicitly release these resources when they are no longer needed.
Here’s an example:
class ResourceHolder : IDisposable
{
private bool disposed = false;
public void Dispose()
{
Dispose(true);
GC.SuppressFinalize(this);
}
protected virtual void Dispose(bool disposing)
{
if (!disposed)
{
if (disposing)
{
// Release managed resources
}
// Release unmanaged resources
disposed = true;
}
}
}
class Program
{
static void Main()
{
using (ResourceHolder resource = new ResourceHolder())
{
// Use the resource
}
}
}
In this code, the ResourceHolder
class implements the IDisposable
interface. The Dispose
method is called when the object is no longer needed, allowing for a clean-up of both managed and unmanaged resources. The using
statement ensures that Dispose
is called automatically when the object goes out of scope. This pattern is essential for managing resources efficiently, especially in applications that require high performance or deal with limited resources.
Finalizers and the Garbage Collector
C# also provides a mechanism for object destruction through finalizers. A finalizer is a special method that is called by the garbage collector before an object is reclaimed. This allows you to perform any necessary cleanup. However, relying solely on finalizers can lead to performance issues, as they delay garbage collection.
Here’s how you can implement a finalizer:
class FinalizerExample
{
~FinalizerExample()
{
// Cleanup code here
}
}
class Program
{
static void Main()
{
FinalizerExample example = new FinalizerExample();
}
}
In this example, the FinalizerExample
class has a finalizer (indicated by the tilde ~
symbol). When the garbage collector determines that there are no references to an instance of FinalizerExample
, it calls the finalizer to perform any necessary cleanup. However, it’s important to use finalizers judiciously, as they can lead to memory leaks if not managed properly. Finalizers should be used only when necessary, preferably in conjunction with the IDisposable
pattern for optimal resource management.
Conclusion
Understanding how to destroy objects in C# is vital for effective memory management and application performance. Whether you choose to assign null values, implement the IDisposable
interface, or use finalizers, each method has its place in your coding toolkit. By mastering these techniques, you can write cleaner, more efficient code that maximizes resource utilization. Remember, good memory management practices not only enhance performance but also improve the overall stability of your applications.
FAQ
-
How do I know when to use null assignment?
Assign null when you no longer need an object, signaling the garbage collector to reclaim memory. -
What is the purpose of the Dispose method?
The Dispose method is used to release unmanaged resources explicitly, improving resource management. -
Are finalizers always necessary in C#?
No, finalizers should be used sparingly and only when necessary, as they can impact performance.
-
What happens if I forget to call Dispose?
Failing to call Dispose can lead to resource leaks, especially for unmanaged resources. -
How can I ensure my objects are cleaned up properly?
Use theusing
statement or implement theIDisposable
pattern to manage resources effectively.
effectively by assigning null values, implementing the IDisposable interface, and using finalizers. This guide offers clear examples and explanations to help you manage memory efficiently in your C# applications. Discover best practices for resource management and improve your coding skills today.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn