Default Access Modifiers in C#
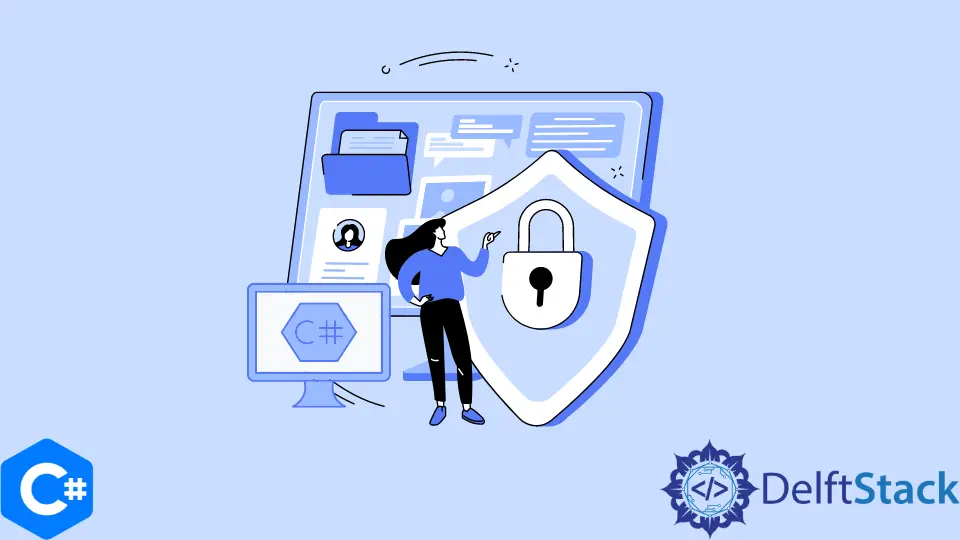
In this guide, we will learn about the access modifiers in C#.
What are access modifiers and their default state? How do you use them, and for what purpose do you use them?
This guide will discuss all relevant details regarding the access modifiers. Let’s dive in.
Default Access Modifiers in C#
To understand the concept of access modifiers, we first need to understand what they are. We assume that you are already familiar with the concepts of object-oriented programming.
Well, the access modifiers in C# are used to set the scope of accessibility of the class and its methods and field members. There are six types of these in C#.
For instance, if we set a public
access modifier to a class, it’ll be accessible everywhere in the program to everyone - with no restrictions for accessing the public
class.
Let’s take a look at all the access modifiers in C#.
public
: No restrictions for accessing the public members.private
: The access is limited to the class definition. If you don’t put any access modifier in C#, this is the default access modifier.protected
: Aprotected
class can be accessed within the class definitions and inside the inherited class.internal
: Access is only limited to the current project assembly.protected internal
: Only the current assembly and types inherited from the enclosing class have access. The variables are accessible to all members of the current project and the derived class.private protected
: Within the current assembly, access is limited to the contained class or types inherited from the containing class.
If you don’t assign any access modifier to a class and its members and methods, by default (which we discuss in this guide), the internal
access modifier will be assigned to the class. And the private
access modifier will be assigned to its methods and fields.
For instance, take a look at the following code.
namespace MyCsharp {
class csharprogram {
void method1() {}
class csharpin {}
}
}
We did not assign any access modifier to the class and its fields and methods in the above code. Hence, by default, the internal
access modifier is assigned to the class csharpprogram
, and the private
access modifier is assigned to its fields and methods.
So, the code will work the same as the following code.
namespace MyCsharp {
internal class csharprogram {
private void method1() {}
private class csharpin {}
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn