How to Deep Copy in C#
- Understanding Deep Copy vs. Shallow Copy
- Using the BinaryFormatter Class for Deep Copying
- Considerations When Using BinaryFormatter
- Conclusion
- FAQ
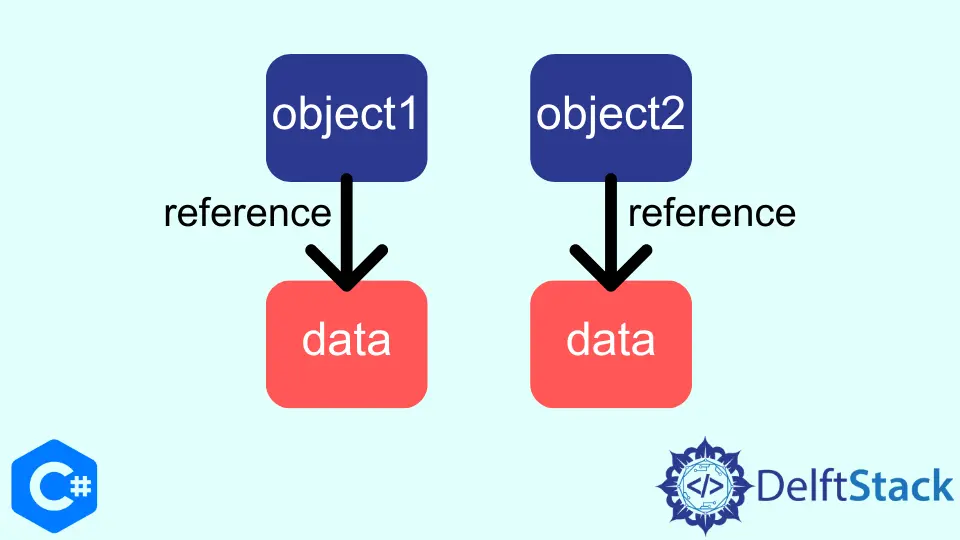
Deep copying in C# is a crucial concept that every developer should understand, especially when working with complex objects. Unlike shallow copying, which merely copies the reference of an object, deep copying creates an entirely new instance of an object along with all the objects referenced by it. This ensures that changes made to the copied object do not affect the original object. One of the most effective ways to achieve deep copying in C# is by utilizing the BinaryFormatter
class.
In this article, we will explore how to implement deep copies in C# using this powerful tool, along with practical examples and detailed explanations.
Understanding Deep Copy vs. Shallow Copy
Before diving into the methods for deep copying, it’s essential to understand the difference between deep and shallow copying. A shallow copy creates a new object, but it does not create copies of the objects that the original object references. Instead, it copies the references to those objects. Consequently, if the original object is modified, the shallow copy reflects those changes.
On the other hand, a deep copy duplicates every object in the object graph, ensuring that the new object is entirely independent of the original. This distinction is vital in scenarios where mutable objects are involved, as it can prevent unintended side effects.
Using the BinaryFormatter Class for Deep Copying
The BinaryFormatter
class in C# is a straightforward way to perform deep copying of objects. It serializes the object into a binary format and then deserializes it back into a new object instance. This method is particularly useful for complex objects that contain nested references.
Here’s how you can implement deep copying using the BinaryFormatter
class:
using System;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
[Serializable]
public class Person
{
public string Name { get; set; }
public Address Address { get; set; }
}
[Serializable]
public class Address
{
public string City { get; set; }
public string Country { get; set; }
}
public class Program
{
public static T DeepCopy<T>(T obj)
{
using (var memoryStream = new MemoryStream())
{
var formatter = new BinaryFormatter();
formatter.Serialize(memoryStream, obj);
memoryStream.Seek(0, SeekOrigin.Begin);
return (T)formatter.Deserialize(memoryStream);
}
}
public static void Main()
{
var original = new Person
{
Name = "John Doe",
Address = new Address { City = "New York", Country = "USA" }
};
var copy = DeepCopy(original);
copy.Name = "Jane Doe";
copy.Address.City = "Los Angeles";
Console.WriteLine($"Original Name: {original.Name}, City: {original.Address.City}");
Console.WriteLine($"Copy Name: {copy.Name}, City: {copy.Address.City}");
}
}
Output:
Original Name: John Doe, City: New York
Copy Name: Jane Doe, City: Los Angeles
In this example, we define two classes, Person
and Address
, both marked with the [Serializable]
attribute. The DeepCopy
method uses BinaryFormatter
to serialize the object to a memory stream and then deserializes it back into a new object. When we modify the copied object’s properties, the original remains unchanged, demonstrating the effectiveness of deep copying.
Considerations When Using BinaryFormatter
While BinaryFormatter
is a powerful tool for deep copying, there are several considerations to keep in mind. First, both the object and all its members must be marked as [Serializable]
. If any member is not serializable, an exception will be thrown during the serialization process.
Moreover, BinaryFormatter
can be less performant for large objects or complex object graphs due to the overhead of serialization and deserialization. Additionally, security concerns have been raised regarding its use, particularly with untrusted data. Therefore, it’s essential to evaluate whether BinaryFormatter
is the right choice for your specific use case.
Conclusion
Deep copying in C# is an essential technique that allows developers to create independent copies of complex objects. By using the BinaryFormatter
class, you can easily serialize and deserialize objects, ensuring that modifications to copies do not affect the originals. While this method is effective, always consider the implications of serialization, especially regarding performance and security. Understanding these concepts will enhance your ability to manage object states effectively in your applications.
FAQ
-
What is the difference between deep copy and shallow copy?
A deep copy creates an independent copy of an object and all the objects it references, while a shallow copy copies only the reference to the object. -
Can I use BinaryFormatter for all types of objects?
No, only objects marked with the [Serializable] attribute can be serialized using BinaryFormatter. -
Are there alternatives to BinaryFormatter for deep copying in C#?
Yes, alternatives include using JSON serialization libraries like Newtonsoft.Json or System.Text.Json for deep copying. -
What are the performance implications of using BinaryFormatter?
BinaryFormatter can be slower for large or complex object graphs due to the overhead of serialization and deserialization. -
Is BinaryFormatter safe to use with untrusted data?
No, BinaryFormatter can pose security risks when used with untrusted data, as it may allow for deserialization attacks.
using the BinaryFormatter class. This comprehensive guide covers the differences between deep and shallow copying, provides practical code examples, and discusses considerations for using BinaryFormatter effectively. Enhance your C# skills and manage object states efficiently with deep copying techniques.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn