How to Convert DataTable to CSV in C#
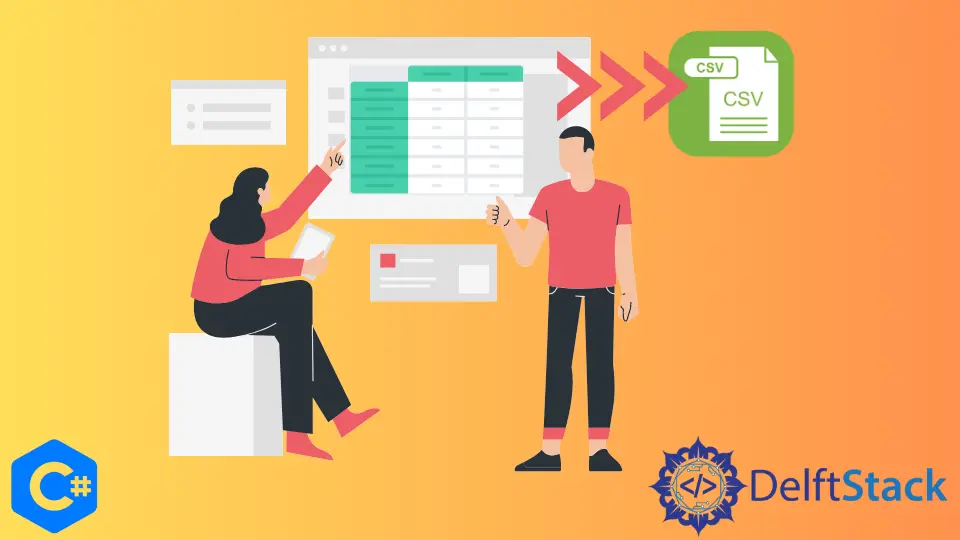
This article will walk you through converting a DataTable to a CSV file using C#. To begin, we must know what CSV is, so let’s get into it.
The data in a Comma Split Values, also known as a CSV file, are separated into their respective columns by commas rather than spaces. Another usage of a CSV file is to open the file in Excel immediately when the data are automatically put into the appropriate cells in Excel.
Convert DataTable to CSV in C#
The first step in converting a DataTable to a CSV file is to create a DataTable. The steps involved in doing so are outlined in the following paragraphs.
Create and Fill the DataTable
-
To begin, we must import the following libraries:
using System; using System.Collections.Generic; using System.Data; using System.IO; using System.Linq; using System.Text; using System.Threading.Tasks;
-
We created a class named
datacreation
with a method calledCreateData()
that generates a DataTable as its return value.
```c#
public static class datacreation {
public static DataTable CreateData() {}
}
```
<!--
test:
command: c#
expected_output: |
Compilation failed: 1 error(s), 0 warnings
-->
-
To begin with data entry, we’ve to create a
DataTable
object nameddt
inCreateData()
.DataTable dt = new DataTable();
-
After the object has been initialized, the next step is to add data columns to the DataTable
dt
. We have included theRollnum
,Firstname
,Lastname
, andSubject
fields, and you are free to add any other areas you need.
```c#
dt.Columns.Add("RollNum", typeof(int));
dt.Columns.Add("Firstname", typeof(string));
dt.Columns.Add("Lastname", typeof(string));
dt.Columns.Add("Subjects", typeof(string));
```
<!--
test:
command: c#
expected_output: |
Compilation failed: 1 error(s), 0 warnings
-->
-
Next, fill in the information in these columns or fields.
dt.Rows.Add(3227, "Zeeshan", "Khan", "C#"); dt.Rows.Add(3290, "Bubby", "Zeeshan", "Java"); dt.Rows.Add(3247, "Osama", "shanii", "C++"); dt.Rows.Add(3156, "Haseeb", "Bhatti", "Python"); dt.Rows.Add(3223, "Saad", "Shukhri", "Mongo Db"); dt.Rows.Add(3267, "Sheraz", "Malik", "MySQl"); dt.Rows.Add(3288, "Nabeel", "Sindho", "PHP"); dt.Rows.Add(3299, "Ahsan", "Chishti", "C");
<!--
test:
command: c#
expected_output: |
Compilation failed: 1 error(s), 0 warnings
-->
-
The
CreateData()
function will return a table of the DataTable type.return dt;
Convert the DataTable to CSV in C#
After we have created the DataTable, the next step is to write the code that will convert the DataTable to CSV.
-
We’ve added a new method called
ConvertTocsv
to theimplementCSV
class. The variablesdt
andpath
are the parameters it takes.public static class implementCSV { public static void ConvertTocsv(this DataTable dt, string path) {} }
<!--
test:
command: c#
expected_output: |
Compilation failed: 1 error(s), 0 warnings
-->
-
In the
ConvertTocsv
method, we’ve created aStreamWriter
object calleds
, which will write data in the specified path.StreamWriter s = new StreamWriter(path, false);
-
We will use a
for
loop to split the data from the DataTable by inserting commas between each column.for (int i = 0; i < dt.Columns.Count; i++) { s.Write(dt.Columns[i]); if (i < dt.Columns.Count - 1) { s.Write(","); } } s.Write(s.NewLine);
<!--
test:
command: c#
expected_output: |
Compilation failed: 1 error(s), 0 warnings
-->
-
Following that, we will now utilize a
foreach
loop, which will write data from the DataTabledt
with a comma.foreach (DataRow dr in dt.Rows) { for (int i = 0; i < dt.Columns.Count; i++) { if (!Convert.IsDBNull(dr[i])) { string value = dr[i].ToString(); if (value.Contains(',')) { value = String.Format("\"{0}\"", value); s.Write(value); } else { s.Write(dr[i].ToString()); } } if (i < dt.Columns.Count - 1) { s.Write(","); } } s.Write(s.NewLine); }
<!--
test:
command: c#
expected_output: |
Compilation failed: 1 error(s), 0 warnings
-->
Complete Source Code
using System;
using System.Collections.Generic;
using System.Data;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace DataTableExport {
public static class datacreation {
public static DataTable CreateData() {
DataTable dt = new DataTable();
dt.Columns.Add("RollNum", typeof(int));
dt.Columns.Add("Firstname", typeof(string));
dt.Columns.Add("Lastname", typeof(string));
dt.Columns.Add("Subjects", typeof(string));
dt.Rows.Add(3227, "Zeeshan", "Khan", "C#");
dt.Rows.Add(3290, "Bubby", "Zeeshan", "Java");
dt.Rows.Add(3247, "Osama", "shanii", "C++");
dt.Rows.Add(3156, "Haseeb", "Bhatti", "Python");
dt.Rows.Add(3223, "Saad", "Shukhri", "Mongo Db");
dt.Rows.Add(3267, "Sheraz", "Malik", "MySQl");
dt.Rows.Add(3288, "Nabeel", "Sindho", "PHP");
dt.Rows.Add(3299, "Ahsan", "Chishti", "C");
return dt;
}
}
public static class implementCSV {
public static void ConvertTocsv(this DataTable dt, string path) {
StreamWriter s = new StreamWriter(path, false);
for (int i = 0; i < dt.Columns.Count; i++) {
s.Write(dt.Columns[i]);
if (i < dt.Columns.Count - 1) {
s.Write(",");
}
}
s.Write(s.NewLine);
foreach (DataRow dr in dt.Rows) {
for (int i = 0; i < dt.Columns.Count; i++) {
if (!Convert.IsDBNull(dr[i])) {
string value = dr[i].ToString();
if (value.Contains(',')) {
value = String.Format("\"{0}\"", value);
s.Write(value);
} else {
s.Write(dr[i].ToString());
}
}
if (i < dt.Columns.Count - 1) {
s.Write(",");
}
}
s.Write(s.NewLine);
}
s.Close();
}
}
}
Create a Windows Form
After creating the data in the DataTable and separating it using commas, the next step is constructing a Windows Form where the data can be shown and then converted to the .csv
format.
-
To get started, let’s create a Windows Form, as shown below, and inside it, we’ll put a
DataGridView
and a button calledConvert data to CSV
. ADataGridView
that displays the created data and a button that converts the DataTable into CSV are included. -
When you have finished executing the Windows Form, it will look like this:
Write the Code for the Windows Form
After we have created the Windows Form, the next step is to write the code for the Convert data to CSV
button.
-
Initialize an object and populate it with data by calling the methods listed below.
DataTable dt = datacreation.CreateData();
-
OpenSavefileDialog()
can be used to save the file with the given name.string filename = OpenSavefileDialog();
-
ConvertTocsv
will be used to create a CSV file from the data we’ve created.dt.ConvertTocsv(filename);
Windows Form Source Code
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {
DataTable dt = datacreation.CreateData();
dataGridView1.DataSource = dt;
}
private void btnCSV_Click(object sender, EventArgs e) {
DataTable dt = datacreation.CreateData();
string filename = OpenSavefileDialog();
dt.ConvertTocsv(filename);
}
private string OpenSavefileDialog() {
string filename = null;
SaveFileDialog saveFileDialog = new SaveFileDialog();
saveFileDialog.Filter = "csv File|*.csv";
saveFileDialog.Title = "Save";
DialogResult dialogResult = saveFileDialog.ShowDialog();
if (dialogResult == DialogResult.OK) {
filename = saveFileDialog.FileName;
}
return filename;
}
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedInRelated Article - Csharp CSV
- How to Write Data Into a CSV File in C#
- How to Read a CSV File and Store Its Values Into an Array in C#
- How to Read a CSV File Into a DataTable in C#
- How to Parse CSV File in C#