How to Count Down Timer in C#
- Understanding the Timer Class
- Creating a Basic Count-Down Timer
- Customizing the Timer
- Conclusion
- FAQ
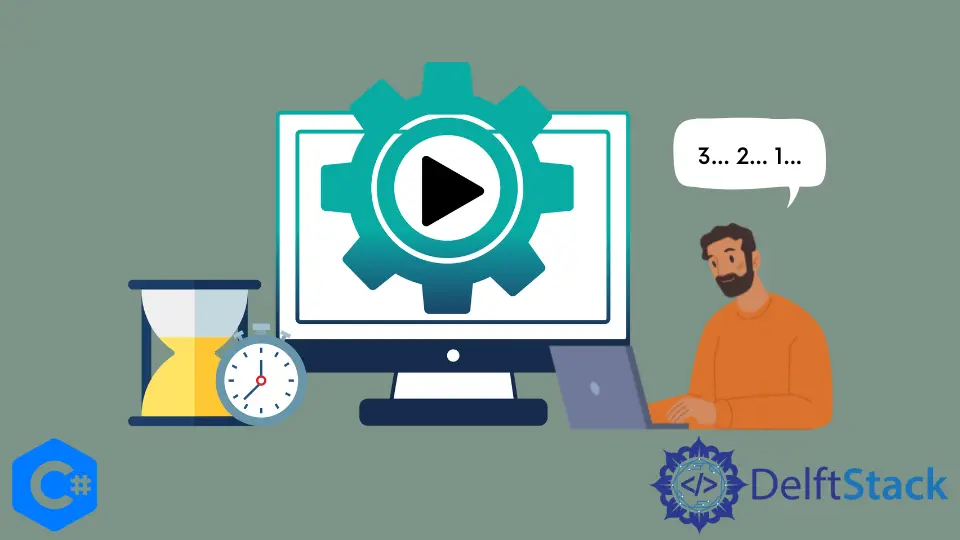
Creating a count-down timer in C# can be a fun and educational project, especially for those looking to enhance their programming skills. The Timer class in C# provides a straightforward way to implement a timer that counts down from a specified duration. Whether you’re building a simple application to track time for games, events, or other activities, understanding how to utilize the Timer class effectively is essential.
In this article, we’ll explore how to set up a count-down timer in C#, complete with code examples and detailed explanations. By the end, you’ll have a solid grasp of how to implement this functionality in your projects.
Understanding the Timer Class
The Timer
class) in C# is part of the System.Timers namespace and is designed to execute a method at specified intervals. When creating a count-down timer, you’ll primarily be interested in setting the interval and handling what happens when the timer elapses. The Timer class is versatile and can be used in various applications, from console apps to GUI-based applications.
To get started, you’ll want to ensure you have the necessary using directives at the top of your C# file:
using System;
using System.Timers;
This allows you to access the Timer class and other essential functionalities. Now, let’s look at how to create a basic count-down timer.
Creating a Basic Count-Down Timer
To implement a count-down timer, you can follow these steps:
- Initialize a Timer instance.
- Set the interval for the timer.
- Create an event handler to define what happens when the timer elapses.
- Start the timer.
Here’s a simple example of how to create a basic count-down timer that counts down from 10 seconds:
using System;
using System.Timers;
class Program
{
static Timer timer;
static int countdownTime = 10;
static void Main(string[] args)
{
timer = new Timer(1000);
timer.Elapsed += OnTimedEvent;
timer.AutoReset = true;
timer.Enabled = true;
Console.WriteLine("Countdown starting...");
Console.ReadLine();
}
private static void OnTimedEvent(Object source, ElapsedEventArgs e)
{
if (countdownTime > 0)
{
Console.WriteLine($"Time left: {countdownTime} seconds");
countdownTime--;
}
else
{
Console.WriteLine("Time's up!");
timer.Stop();
}
}
}
Output:
Countdown starting...
Time left: 10 seconds
Time left: 9 seconds
Time left: 8 seconds
Time left: 7 seconds
Time left: 6 seconds
Time left: 5 seconds
Time left: 4 seconds
Time left: 3 seconds
Time left: 2 seconds
Time left: 1 seconds
Time's up!
In this example, we set up a timer that ticks every second (1000 milliseconds). The OnTimedEvent
method is triggered each time the timer elapses. It checks if there’s time left in the countdown. If there is, it decrements the countdown and prints the remaining time. Once the countdown reaches zero, it stops the timer and prints a message indicating that time is up.
Customizing the Timer
The basic count-down timer can be customized further to fit your needs. For instance, you might want to allow the user to set the countdown duration or provide options to pause and resume the timer. Here’s an enhanced version of the previous example that allows user input for the countdown time:
using System;
using System.Timers;
class Program
{
static Timer timer;
static int countdownTime;
static void Main(string[] args)
{
Console.Write("Enter countdown time in seconds: ");
countdownTime = Convert.ToInt32(Console.ReadLine());
timer = new Timer(1000);
timer.Elapsed += OnTimedEvent;
timer.AutoReset = true;
timer.Enabled = true;
Console.WriteLine("Countdown starting...");
Console.ReadLine();
}
private static void OnTimedEvent(Object source, ElapsedEventArgs e)
{
if (countdownTime > 0)
{
Console.WriteLine($"Time left: {countdownTime} seconds");
countdownTime--;
}
else
{
Console.WriteLine("Time's up!");
timer.Stop();
}
}
}
Output:
Enter countdown time in seconds: 5
Countdown starting...
Time left: 5 seconds
Time left: 4 seconds
Time left: 3 seconds
Time left: 2 seconds
Time left: 1 seconds
Time's up!
In this version, the user is prompted to enter the desired countdown time. The rest of the functionality remains the same, but it allows for greater flexibility. You can further enhance this by adding features like pause, resume, or reset options, depending on your project requirements.
Conclusion
Creating a count-down timer in C# using the Timer class is a straightforward process that opens up many possibilities for application development. By understanding how to implement and customize the Timer class, you can create engaging applications that require time tracking. Whether you’re building a game, a productivity tool, or simply experimenting with C#, mastering the Timer class is a valuable skill.
As you continue to explore C#, consider experimenting with more advanced features, such as threading or asynchronous programming, to further enhance your timer applications. Happy coding!
FAQ
-
What is the Timer class in C#?
The Timer class in C# is a part of the System.Timers namespace that allows you to execute a method at specified intervals. -
Can I customize the countdown duration in my timer?
Yes, you can allow user input to set the countdown duration or modify the code to include preset durations. -
How can I pause and resume the timer?
You can manage the timer’s state by using theStop()
method to pause andStart()
to resume the timer. -
Is the Timer class suitable for GUI applications?
Yes, the Timer class can be used in both console and GUI applications, but for GUI apps, consider using the Windows Forms Timer for better integration. -
Can I use the Timer class for tasks other than countdowns?
Absolutely! The Timer class can be used for various time-based tasks, such as scheduling recurring events or executing background tasks at intervals.
using the Timer class. This guide provides step-by-step instructions, code examples, and tips for customizing your timer application. Perfect for beginners and experienced developers alike, discover how to track time effectively in your C# projects.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn