How to Convert String to Datetime in C#
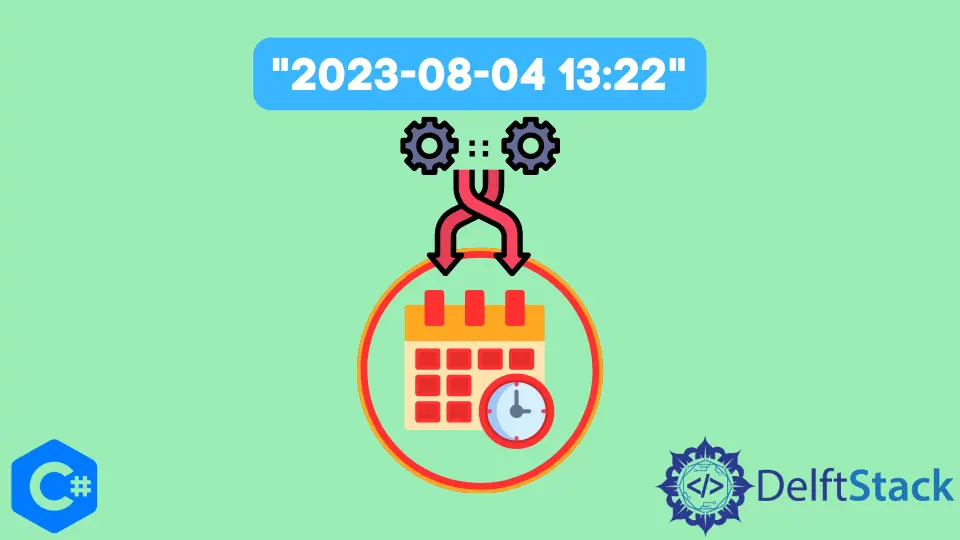
This guide will teach us to convert a string into a datetime in C#. We can also convert a string to datetime in a specific format, for instance, yyyy-mm-dd hh mm ss
.
We need to be familiar with the CultureInfo
to understand this. Let’s dive into this guide and learn everything about it.
Convert String to Datetime in C#
To convert a string to datetime, we already know that the string should be written in a specific format. A format that clearly shows the day, month, and year.
Only then would we proceed with this as mentioned above; this method requires knowledge about CultureInfo
. Let’s understand that first.
First of all, you need to import using System.Globalization;
library to use the culture info and its functions.
The syntax is as follows: CultureInfo
. CultureInfo
contains the information for the culture, writing system, names of the culture, the sort order of strings, and the actual formatting of dates and numbers.
The objects in it are returned by properties such as CompareInfo
. The cultures are grouped into one of the three invariant cultures.
The DateTimeFormat
and the NumberFormat
also reflect formatting conventions and string comparison.
Learn more about the CultureInfo
in this reference.
You need to pass your specific written string inside a DateTime.ParseExact()
along with the format and the culture information.
Once you have written the string in a specific format, you need to match the same format while passing it inside the DateTime.ParseExact()
. Now, let’s understand the code and the implementation of converting a string to a datetime.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Globalization; /// To use CultureInfo
namespace String_To_Date_Time {
class Program {
static void Main(string[] args) {
// First Method Using DateTime.ParseExact;
string str = "2022-11-22 13:22";
DateTime d = DateTime.ParseExact(str, "yyyy-MM-dd HH:mm", CultureInfo.InvariantCulture);
// Throws Exception if the Format Is Incorrect...
Console.WriteLine(d);
Console.Read();
}
}
}
We passed the string str
inside the function and the same format defined in a string. If the written string does not have a correct format, this function will throw the exception saying the format is incorrect.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Csharp String
- How to Convert String to Enum in C#
- How to Convert Int to String in C#
- How to Use Strings in Switch Statement in C#
- How to Convert a String to Boolean in C#
- How to Convert a String to Float in C#
- How to Convert a String to a Byte Array in C#