How to Convert String to Char in C#
-
Convert String to Character With the
char.Parse()
Function inC#
-
Convert String to Character With the
string[index]
Method inC#
-
Convert String to Array of Characters With the
string.ToCharArray()
Function inC#
-
Convert Array of Strings to List of Character Arrays With the LINQ Method in
C#
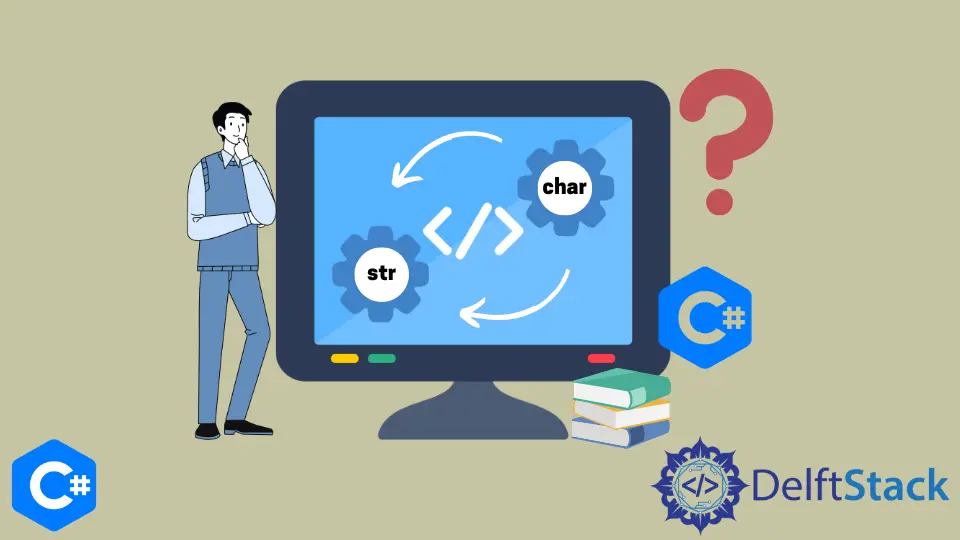
This tutorial will introduce the methods to convert a string to a character in C#.
Convert String to Character With the char.Parse()
Function in C#
If we have a string variable containing only a single character in it and want to convert it to a char variable, we can use the char.Parse()
function in C#. The char.Parse()
function is used to parse a string variable with length 1
to a character variable in C#. The char.Parse()
function takes a string variable as a parameter and returns a character. The following code example shows us how to convert a string to a character with the char.Parse()
function in C#.
using System;
namespace string_to_char {
class Program {
static void Main(string[] args) {
string str = "S";
char c = char.Parse(str);
Console.WriteLine(c);
}
}
}
Output:
S
In the above code, we converted the string variable str
containing the value S
to the character type variable c
with the value S
by using the char.parse()
function in C#. The approach cannot be used with a string variable that contains more than one character.
Convert String to Character With the string[index]
Method in C#
The string
data type also works like an array of characters in C#. We can get the character at the nth index of the string with the string[n]
method in C#. This method can also be used with a string variable that contains more than one character. The following code example shows us how to convert a string variable containing multiple characters to a character variable with the string[index]
function in C#.
using System;
namespace string_to_char {
class Program {
static void Main(string[] args) {
string str = "String";
char c = str[0];
Console.WriteLine(c);
}
}
}
Output:
S
In the above code, we converted the first element of the string variable str
into the character variable c
with the str[0]
method in C#.
Convert String to Array of Characters With the string.ToCharArray()
Function in C#
If we have a string variable containing multiple characters in it and want to convert the whole string into an array of characters, we have to use the string.ToCharArray()
function in C#. The string.ToCharArray()
function takes a string variable as an argument and returns an array of characters containing each character. See the following code example.
using System;
namespace string_to_char {
class Program {
static void Main(string[] args) {
string str = "String";
char[] charArray = str.ToCharArray();
Console.WriteLine(charArray);
}
}
}
Output:
String
In the above code, we converted all the characters inside the string variable str
to the array of characters charArray
with the str.ToCharArray()
function in C#.
Convert Array of Strings to List of Character Arrays With the LINQ Method in C#
The LINQ integrates query functionality with data structures in C#. The ToList()
function of LINQ is used to convert a collection of elements to a list in C#. We can convert an array of string variables to a list containing arrays of characters with LINQ and ToCharArray()
function in C#. The following code example shows us how we can convert an array of string variables to a list of character arrays with the LINQ method in C#.
using System;
namespace string_to_char {
class Program {
static void Main(string[] args) {
string[] stringArray = { "First", "Second", "Third" };
var charArraysList = stringArray.Select(str => str.ToCharArray()).ToList();
foreach (var charArray in charArraysList) {
Console.WriteLine(charArray[0]);
}
}
}
}
Output:
F
S
T
In the above code, we converted the array of strings stringArray
to a list of character arrays charArraysList
with LINQ in C#. We selected each string from the stringArray
and converted it to an array of characters with the string.ToCharArray()
function. Then, we converted all the character arrays to a list with the ToList()
function of LINQ and saved the result into the charArraysList
variable. In the end, we printed all the elements inside the charArraysList
.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn