How to Convert Int to Float in C#
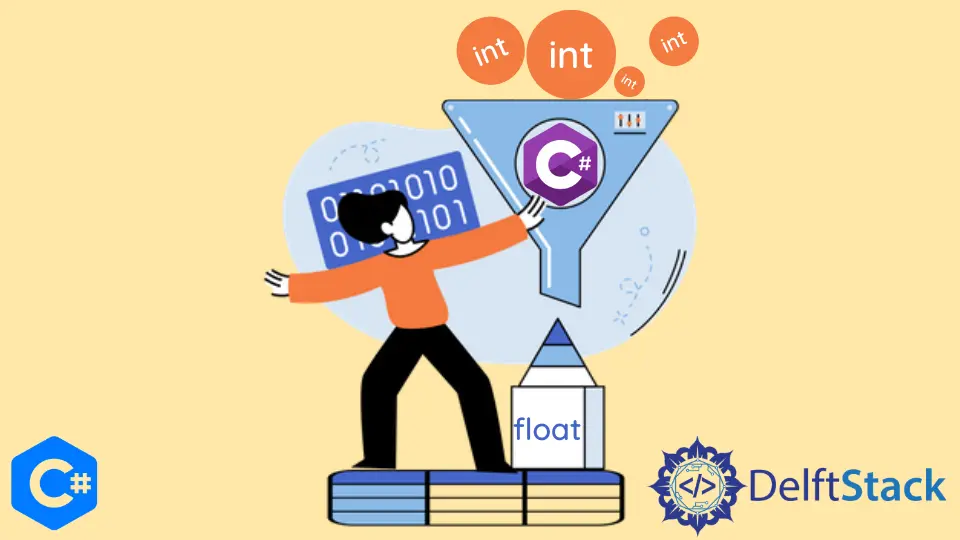
This guide will teach us to convert an int
to a float
in C#. It can be done simply by casting.
We can easily convert an int
to a float
or decimal
using the typecasting method. Let’s take a look.
Convert Int
to Float
in C#
We can use the type casting to convert an int
to float
. By writing (float)
behind the int
variable.
For instance, if your int
variable is temp_int
, to convert the value inside to a float
value, all you need to do is to write (float)temp_int
. Take a look at the following code.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Int_to_Float {
class Program {
static void Main(string[] args) {
// using type cast we can convert INT TO FLOAT OR DECIMAL...........
int temp_int = 1;
float temp_float = (float)temp_int;
decimal temp_decimal = 3;
float temp_float_decimal = (float)temp_decimal;
Console.WriteLine("INT TO FLOAT= " + temp_float);
Console.WriteLine("FLOAT TO DECIMAL= " + temp_float_decimal);
Console.ReadKey();
}
}
}
The output of the code is given below.
output:
INT TO FLOAT= 1
FLOAT TO DECIMAL= 3
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Csharp Int
- How to Convert a Char to an Int in C#
- How to Convert Char to Int in C#
- How to Convert Int to Byte in C#