How to Add Items in C# ComboBox
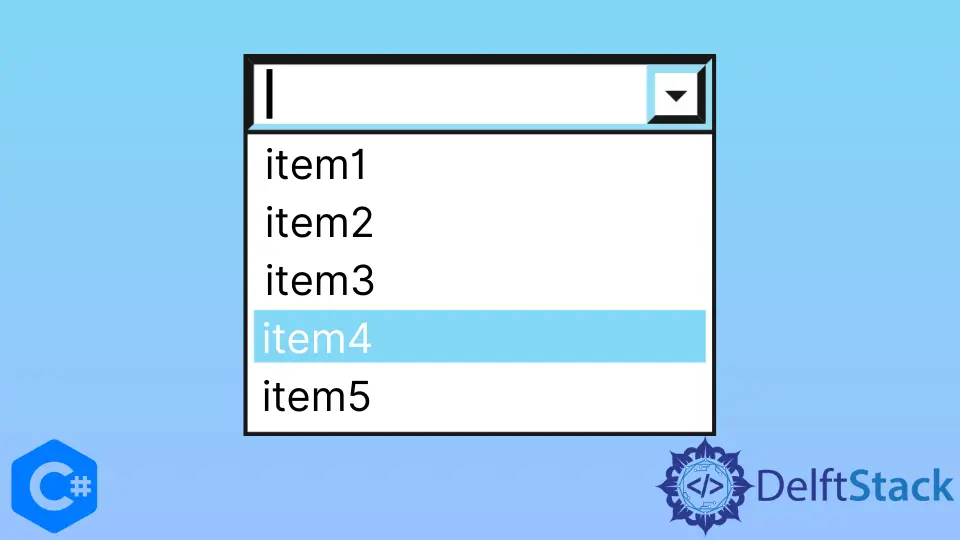
In this guide, we’ll see how to add items to ComboBox in C#.
Create a ComboBox in C#
Before adding an item to a ComboBox, we must understand how a ComboBox is created. We have two methods for creating a ComboBox.
We can use windows forms or the ComboBox
class at run time to create it in C#.
Use ToolBox to Create a ComboBox in C#
Using the toolbox, we can create a ComboBox control at design time. We can drag ComboBox from the toolbox, and it will be created.
We create as we want using its properties. By right-clicking on the newly created ComboBox, we can add items to it.
Use ComboBox
Class to Create a ComboBox in C#
Creating a ComboBox at run-time is a little more tricky and more complex than by toolbox(Design-time). In the following example, we’ll use the ComboBox
class to create a ComboBox and add an item to it.
Add an Item in ComboBox in C#
As mentioned above, adding an item in ComboBox can be a little more complex than using a toolbox. We have to use the Add()
method to add an item, and this method is from the ObjectCollection
class.
We use a ComboBox()
constructor to create a ComboBox so that we can add our items to it. We can also set its properties to our desires.
After creating a ComboBox, items can now be added. At last, we have to use the Add()
method to add the ComboBox control to the form.
Code:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp1 {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e) {
ComboBox comboBox1 = new ComboBox(); // creating a combobox
comboBox1.Location = new Point(327, 77);
comboBox1.Size = new Size(216, 26);
comboBox1.Name = "MY COMBOBOX";
comboBox1.Items.Add(389); // adding items in combobox
comboBox1.Items.Add(390);
comboBox1.Items.Add(391);
this.Controls.Add(comboBox1); // Adding this ComboBox to the form
}
}
}
Output:
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn