How to Clear TextBox in C#
-
Clear a TextBox With the
String.Empty
Property inC#
-
Clear a TextBox With the
TextBox.Text=""
Method inC#
-
Clear a TextBox With the
TextBox.Clear()
Function inC#
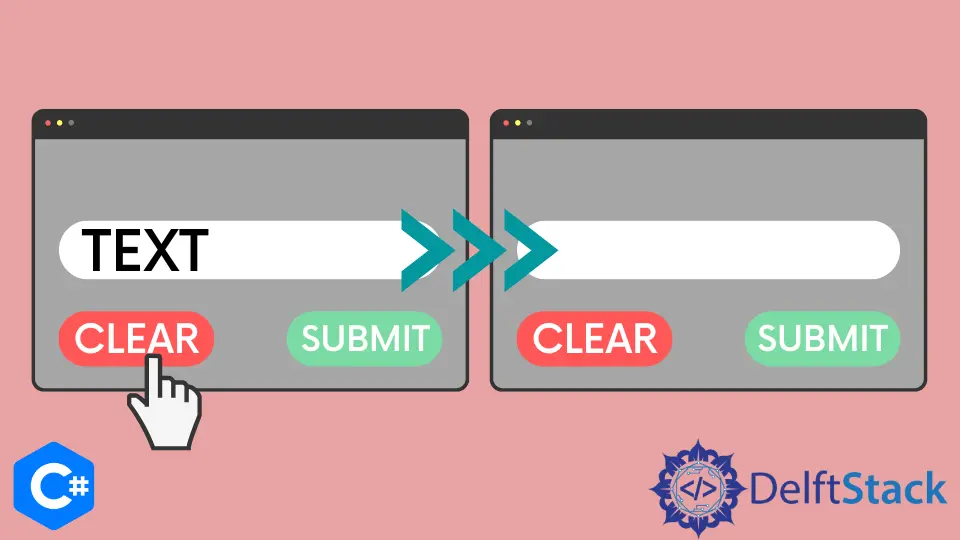
This tutorial will discuss the methods to clear a text box in C#.
Clear a TextBox With the String.Empty
Property in C#
The String.Empty
property represents an empty string in C#. It is equivalent to ""
in C#. We can set the text of our TextBox
to be equal to String.Empty
to clear our text box. The following code example shows us how we can clear a text box with the String.Empty
property in C#.
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
textBox1.Text = String.Empty;
}
}
}
In the above code, we cleared all the text inside the textBox1
text box by making it equal to String.Empty
in C#.
Clear a TextBox With the TextBox.Text=""
Method in C#
In the previous approach, the String.Empty
property represented an empty string and was equal to ""
. We can also clear our text box by making the TextBox.Text
property equal to ""
. The following code example shows us how we can clear a text box with the TextBox.Text=""
method in C#.
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
textBox1.Text = "";
}
}
}
In the above code, we cleared all the text inside the textBox1
text box, by making it equal to ""
in C#.
Clear a TextBox With the TextBox.Clear()
Function in C#
The TextBox.Clear()
function is used to clear all the text inside a text box in C#. The following code example shows us how we can clear a text box with the TextBox.Clear()
function in C#.
using System;
using System.Windows.Forms;
namespace check_if_textbox_is_empty {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
textBox1.Clear();
}
}
}
In the above code, we cleared all the text inside the textBox1
text box with the textBox1.Clear()
function in C#.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn